Call Function by Name in Python In the earlier days, we were using functions by their name and we didn’t use arguments. When we learned to program, we understood how to call functions by their names and the arguments used in calling functions.
We all know that the main thing behind writing a program is to save our time and resources. As we are writing a program, we will face various problems in the coding. We may face errors while creating a function or a variable, but we don’t know why it is happening.
We will know the reason when we will see a traceback. Traceback is a message that displays the error in the console. If we write a program with traceback, it will be helpful for us to fix the errors.
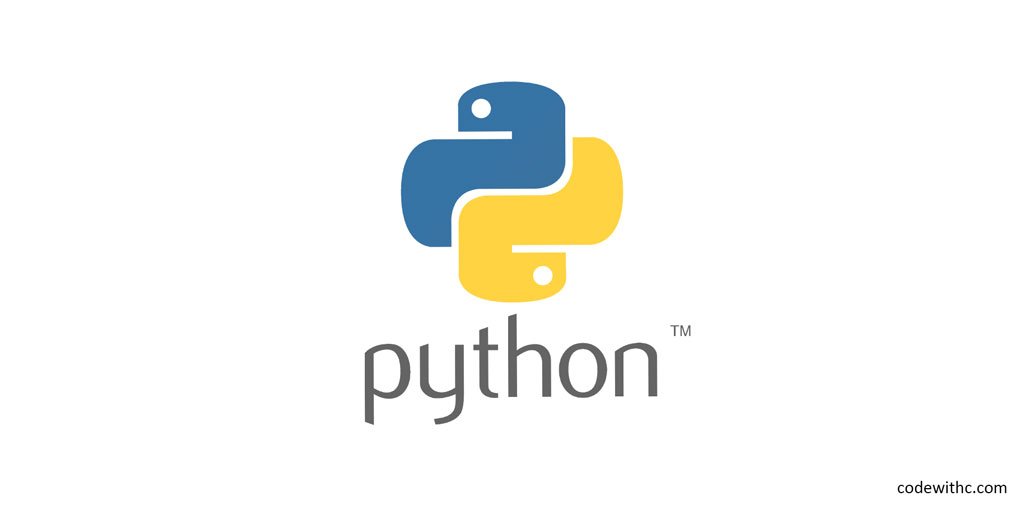
We can fix the errors by analyzing the traceback. We will get to know the reason behind the error and we will be able to find out the solution. But the traceback will be helpful when we are using the functions because we can see the name of the function that is causing the error.
Let’s learn how to call a function by its name in Python.
Suppose, we have two functions called ‘print_something’ and ‘add_one’. Now, we will call them by their names as print_something and add_one.
Python code:
print_something()
add_one()
Output:
Traceback (most recent call last):
File “C:/pythontest/test.py”, line 3, in
print_something()
NameError: name ‘print_something’ is not defined
Now, if we want to call a function by its name and use its arguments, we need to use the *args and **kwargs arguments. Let’s try to understand them.
Python code:
def print_something(*args, **kwargs):
print(f’Hello World! {args} and {kwargs}')
print_something()
print_something(‘Hi!’)
print_something(a=10, b=20, c=30)
Output:
Hello World! Hi! and {‘c’: 30, ‘b’: 20, ‘a’: 10}
We can see that the function ‘print_something’ takes the arguments in the form of ‘*args’ and ‘**kwargs’.
Let’s see another example.
Python code:
def add_one(x, y):
return x + y
add_one()
add_one(2, 3)
Output:
5
7
So, the arguments are passed to the function using the keyword arguments.