Using Operators in Python Programming for Effective Calculation
π Welcome, Python enthusiasts! Today, we are diving into the thrilling world of operators in Python programming π. Buckle up as we embark on a rollercoaster ride through arithmetic, comparison, logical, assignment, and bitwise operators. Letβs sprinkle some Python magic and unravel the mysteries of effective calculation using these awesome tools! π«
Handling Arithmetic Operators
Addition and Subtraction
Have you ever danced with the plus and minus signs in Python? ππΊ Letβs groove through addition and subtraction, overcoming challenges with the tricky order of operations along the way! π΅
- Using the plus and minus signs can lead to some funky outcomes! π
- Remember to watch out for the order of operations to avoid Python playing tricks on you! π©
Multiplication and Division
Time to unleash the power of the asterisk and slash symbols for multiplication and division! π Donβt fall into the integer division pitfall; itβs a wild ride! π’
- The asterisk and slash symbols are your companions in the journey of multiplication and division! π
- Beware of integer division lurking around the corner, ready to surprise you when you least expect it! π
Exploring Comparison Operators
Equal to and Not Equal to
Letβs talk about equality and inequality using double equals and exclamation equals in Python! π€ Avoid common mistakes and sail smoothly through your comparison journeys! β΅
- Double equals and exclamation equals are your trusty sidekicks in the land of equality comparisons! π―
- Donβt let common mistakes ruin your day; embrace the equality comparisons with confidence! πͺ
Greater than and Less than
Time to don the hats of greater and less than symbols for some thrilling comparison operations in Python! π© Remember, data types may differ, but your comparison skills will shine bright! β¨
- Greater than and less than symbols are your tools to compare values like a pro! π
- Embrace the challenge of managing different data types in comparisons; itβs all part of the fun in Python! π
Employing Logical Operators
AND Operator
Ready to meet the logical βandβ operator in action? Itβs your ticket to handling multiple conditions with finesse in Python! π«
- The logical βandβ operator is here to help you navigate through multiple conditions like a boss! π΅οΈββοΈ
- Juggle those conditions with ease using the βandβ operator; Python logic has never been more fun! πͺ
OR Operator
Time to unleash the power of the logical βorβ operator for inclusive conditions in Python! π Letβs make our code dance with flexibility! π
- The βorβ operator opens doors to inclusive conditions, letting your code breathe freely! π¬οΈ
- Embrace the magic of βorβ for versatile conditions; your Python code will thank you later! π
Mastering Assignment Operators
Simple Assignment
Assigning values using the equal sign seems simple, right? Letβs ensure smooth variable assignments in Python with some pizzazz! βοΈ
- The equal sign may look innocent, but it holds the key to seamless variable assignments in Python! π
- Donβt underestimate the power of simple assignment; itβs the foundation of your Python journey! ποΈ
Compound Assignment
Time to level up with operators like β+=β, β-=β, and more for efficient and concise code in Python! π Letβs simplify our Python adventures! π―
- Compound assignments are like shortcuts in Python; they bring efficiency and elegance to your code! β¨
- Embrace the magic of compound assignment operators; your Python code will thank you for the makeover! π
Unveiling Bitwise Operators
Bitwise AND and OR
Ready to dive into the world of bitwise operators using β&β and β|β for fascinating binary operations in Python? π€ Letβs decode the binary mysteries together! π
- β&β and β|β symbols hold the key to unlock the wonders of binary operations in Python; are you ready for the challenge? π²
- Understanding binary representation is the secret sauce to mastering bitwise operators; letβs decode the matrix! π΅οΈββοΈ
Bitwise Shift Operators
Time to shift bits left and right using β<<β and β>>ββa thrilling adventure of bitwise operations awaits! π Letβs navigate through the binary seas with confidence! π
- β<<β and β>>β operators are your companions in navigating the binary world with finesse in Python! π§
- Embrace the art of bitwise shifting; itβs like controlling the gears of a powerful machine in Python! π
π Overall, operators in Python are like magic wands that empower you to perform dazzling calculations and comparisons with ease and elegance. Remember, practice makes perfect, so keep coding and exploring the wonders of Python! π
π Thank you for joining me on this whimsical journey through Python operators! Stay curious, keep coding, and always rememberβPython is your playground for endless possibilities! ππ
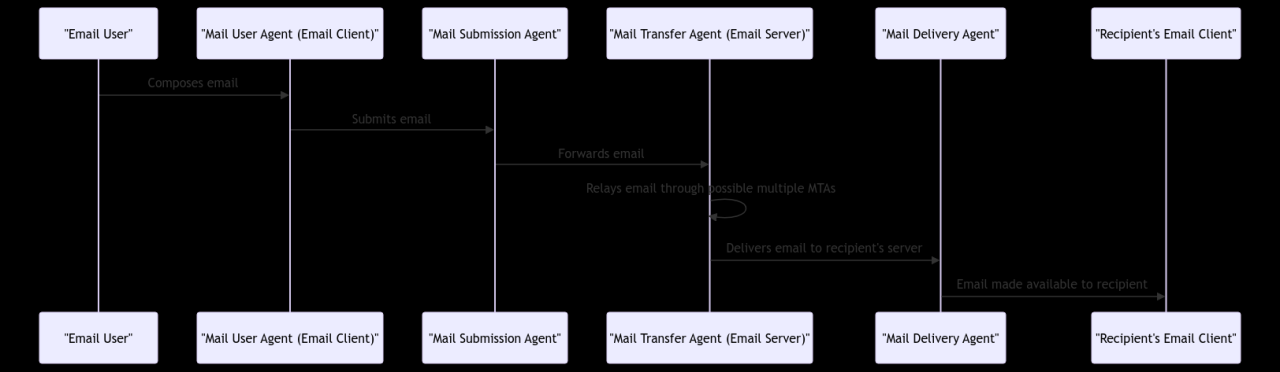
Program Code β Using Operators in Python Programming for Effective Calculation
# Importing the Operator module
import operator
# Function to demonstrate different operators
def demonstrate_operators():
# Initializing variables
a = 15
b = 4
# Using arithmetic operators
print('Arithmetic Operators')
print('a + b =', operator.add(a, b)) # Addition
print('a - b =', operator.sub(a, b)) # Subtraction
print('a * b =', operator.mul(a, b)) # Multiplication
print('a / b =', operator.truediv(a, b)) # Division
print('a // b =', operator.floordiv(a, b)) # Floor Division
print('a % b =', operator.mod(a, b)) # Modulus
print('a ** b =', operator.pow(a, b)) # Exponent
print()
# Using comparison operators
print('Comparison Operators')
print('a == b :', operator.eq(a, b)) # Equal to
print('a != b :', operator.ne(a, b)) # Not equal to
print('a > b :', operator.gt(a, b)) # Greater than
print('a < b :', operator.lt(a, b)) # Less than
print('a >= b :', operator.ge(a, b)) # Greater than or equal to
print('a <= b :', operator.le(a, b)) # Less than or equal to
print()
# Logical operators (example with boolean values)
print('Logical Operators')
x = True
y = False
print('x and y is', operator.and_(x, y)) # Logical AND
print('x or y is', operator.or_(x, y)) # Logical OR
print('not x is', operator.not_(x)) # Logical NOT
print()
# Calling the function
demonstrate_operators()
Code Output:
Arithmetic Operators
a + b = 19
a - b = 11
a * b = 60
a / b = 3.75
a // b = 3
a % b = 3
a ** b = 50625
Comparison Operators
a == b : False
a != b : True
a > b : True
a < b : False
a >= b : True
a <= b : False
Logical Operators
x and y is False
x or y is True
not x is False
Code Explanation:
This program is a humble demonstration of using various operators available in Python by leveraging the built-in operator
module, thus showcasing the utility and power of Python for effective calculations and logical operations.
How does it work? The highlight of this program is how it nicely unfolds into three categories β arithmetic, comparison, and logical operators, making it easier to perceive how each set functions distinctly.
In the Arithmetic Operators section, we operate on two integers a
and b
to perform common mathematical operations. The operator
module provides functions like add()
, sub()
, mul()
, etc., making the code readable and straightforward.
Following this, the Comparison Operators segment deals with comparing these numbers, returning boolean values as outcomes. Functions such as eq()
, ne()
, gt()
, etc., are used, representing an array of comparison operations such as equal to, not equal to, greater than, and so on.
Finally, the Logical Operators part uses boolean values (x
and y
) to demonstrate logical operations. The use of and_()
, or_()
, and not_()
mimics the AND, OR, and NOT operations, pivotal in condition and flow control statements in Python.
The programβs architecture cleverly divides the use of operators into distinct sections, making it a helpful reference for understanding and utilizing operators in Python effectively. Through this, it achieves its objective of not just performing calculations, but also of serving as an educational tool for understanding the versatility of operators in Python.
Frequently Asked Questions about Using Operators in Python Programming for Effective Calculation
What are operators in Python programs?
Operators in Python are symbols that perform operations on variables and values. They are used to manipulate data and perform calculations in a program.
How can operators be categorized in Python?
Operators in Python can be categorized into different types such as arithmetic operators, assignment operators, comparison operators, logical operators, bitwise operators, and more. Each type serves a specific purpose in programming.
Can you give examples of operators commonly used in Python programs?
Sure! Some common operators used in Python programs include addition (+), subtraction (-), multiplication (*), division (/), assignment (=), equal to (==), less than (<), greater than (>), logical AND (and), logical OR (or), bitwise AND (&), and bitwise OR (|).
How do operators contribute to effective calculation in Python programming?
Operators play a crucial role in performing mathematical and logical operations efficiently in Python programs. By using the right operators, programmers can manipulate data and perform calculations accurately and quickly.
Are there any advanced operators in Python that programmers should be aware of?
Yes, Python also offers advanced operators such as the modulus operator (%), exponentiation operator (**), floor division operator (//), and more. These operators provide additional functionality for complex calculations in programs.
How can I ensure the correct use of operators in my Python programs?
To utilize operators effectively in Python programming, itβs essential to understand the precedence and associativity of operators. By following the operator precedence rules and using parentheses when needed, programmers can avoid errors and ensure accurate calculations.
Can I create custom operators in Python for specific tasks?
While Python does not natively support the creation of custom operators, programmers can achieve similar functionality by defining functions or using existing operators creatively to tailor operations to their specific requirements.
Where can I find more information on using operators in Python programming?
For additional resources and tutorials on operators in Python programming, you can refer to online documentation, Python programming books, and coding forums to deepen your understanding and enhance your programming skills.