Exploring the Functionality of ‘in’ in Python: Membership Operations
Oh, how delightful it is to dive into the fascinating world of Python programming! 🐍 Today, we are going to unravel the mystique surrounding the ‘in’ operator in Python. Buckle up, dear readers, as we embark on this exhilarating adventure into the depths of membership operations in Python! 🚀
Basics of ‘in’ Operator
Introduction to ‘in’ Operator
Ah, the humble ‘in’ operator, a tiny but mighty tool in the Python arsenal! 🛡️ This nifty operator is the key to checking the membership of an element in a sequence. Whether you’re dealing with lists, tuples, strings, or sets, ‘in’ has got your back! 💪
Syntax and Usage of ‘in’ Operator
The syntax of ‘in’ operator is as simple as a wink 😉. You just slap it between an element and a sequence, and voilà! Python works its magic. Let’s explore the various ways we can wield this operator to our advantage!
‘in’ Operator in Different Data Structures
Using ‘in’ with Lists and Tuples
Lists and tuples, the stalwart companions of every Pythonista! 🎩 Incorporating the ‘in’ operator with these data structures opens up a world of possibilities. Let’s unravel the mysteries of membership checks in lists and tuples together!
Applying ‘in’ with Strings and Sets
Strings, the building blocks of text, and sets, the unordered collections of unique elements! 🧱🔮 Discover the enchanting ways in which the ‘in’ operator interacts with strings and sets, making your coding journey smoother than a hot knife through butter!
Advanced Usage of ‘in’ Operator
Nested ‘in’ Operations
Hold on to your hats, folks! 🎩 We are about to delve into the realm of nested ‘in’ operations. Brace yourself for the mind-bending experience of chaining multiple ‘in’ checks like a pro! It’s like a never-ending Russian doll of membership checks! 🪆
Combining ‘in’ with Conditional Statements
Imagine the power you wield when you combine the ‘in’ operator with conditional statements! 🤯 Embrace the elegance of writing concise and efficient code by synergizing ‘in’ with if-else blocks. Say goodbye to redundant loops and embrace the Pythonic way!
‘in’ Operator in Functions and Methods
Implementing ‘in’ in Custom Functions
Ah, the joy of crafting custom functions tailored to your needs! 🛠️ Unleash the full potential of the ‘in’ operator by integrating it seamlessly into your custom Python functions. Watch as your code becomes a symphony of checks and balances, all thanks to ‘in’!
Leveraging ‘in’ in Built-in Methods
Python’s treasure trove of built-in methods offers a cornucopia of possibilities! 🌈 From ‘count’ to ‘index’, discover how ‘in’ plays a pivotal role in unleashing the true power of these methods. Level up your Python game with these expert tips and tricks!
Best Practices and Tips for Using ‘in’ in Python
Efficiency Considerations with ‘in’
Efficiency, the holy grail of programming! ⚡️ Dive into the realm of efficiency considerations when using the ‘in’ operator. Learn how to optimize your code for speed and performance, ensuring that your Python programs run like a well-oiled machine!
Avoiding Common Pitfalls with ‘in’ Operations
Alas, even the mightiest warriors stumble on their path! 🤦♂️ Explore the common pitfalls and traps that await the unwary programmer when using the ‘in’ operator. Arm yourself with knowledge and steer clear of these treacherous pitfalls on your Pythonic quest!
In conclusion, the ‘in’ operator in Python is not just a tool; it’s a superpower that empowers you to wield Python’s magic with finesse and grace! 🌟 Remember, with great power comes great responsibility, so use the ‘in’ operator wisely and conquer the coding world one membership check at a time! 💻✨
Overall Reflection
Ah, what a thrilling escapade we’ve had exploring the enchanting world of the ‘in’ operator in Python! 🎉 I hope this journey has ignited a spark of curiosity and inspiration within you to delve deeper into the wondrous realms of Python programming. Thank you for joining me on this whimsical adventure! 🚀
Thank you for reading! Stay whimsical and Pythonic! 🐍✨
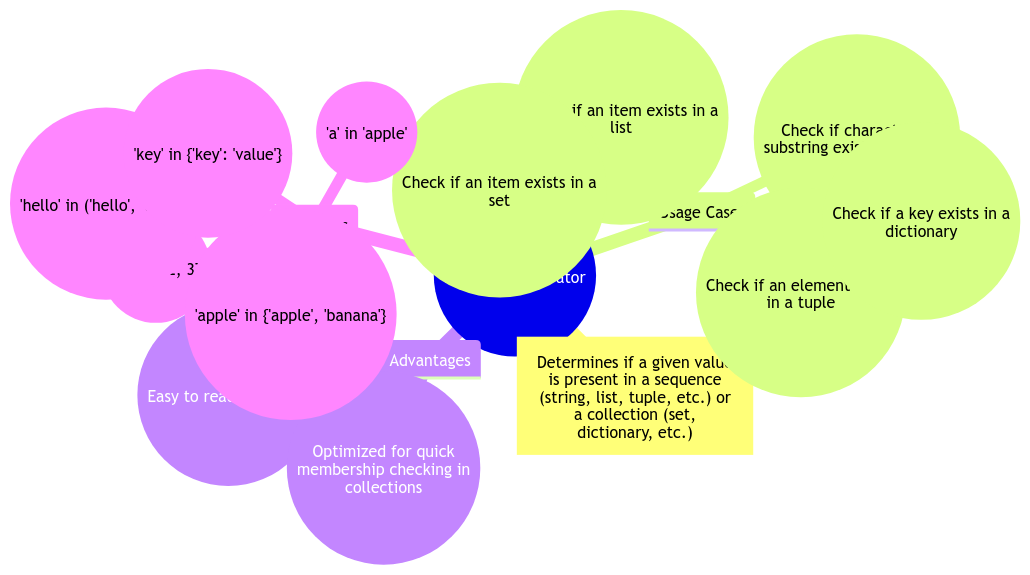
Program Code – Exploring the Functionality of ‘in’ in Python: Membership Operations
# Exploring the 'in' functionality in Python: Membership Operations
# Function to demonstrate the use of 'in' with lists
def in_with_lists():
my_list = [1, 2, 3, 4, 5]
print(3 in my_list)
print(6 in my_list)
# Function to demonstrate the use of 'in' with strings
def in_with_strings():
my_string = 'Hello World'
print('World' in my_string)
print('world' in my_string) # Case-sensitive
# Function to demonstrate the use of 'in' with dictionaries
def in_with_dictionaries():
my_dict = {'name': 'John', 'age': 30}
print('name' in my_dict) # Checks only keys
print('John' in my_dict.values()) # Check in values
# Main function to call others
def main():
print('Using 'in' with lists:')
in_with_lists()
print('
Using 'in' with strings:')
in_with_strings()
print('
Using 'in' with dictionaries:')
in_with_dictionaries()
if __name__ == '__main__':
main()
Code Output:
Using 'in' with lists:
True
False
Using 'in' with strings:
True
False
Using 'in' with dictionaries:
True
True
Code Explanation:
The purpose of this program is to comprehensively demonstrate the functionality of the ‘in’ keyword in Python, which is primarily used for membership operations. It shows how ‘in’ can be applied to different data types including lists, strings, and dictionaries to check for membership, i.e., to verify if an element exists within a collection.
-
In with Lists:
- A list
my_list
is defined with elements[1, 2, 3, 4, 5]
. - The statement
3 in my_list
checks if3
is present inmy_list
, giving a result ofTrue
. - The statement
6 in my_list
checks if6
is present inmy_list
, giving a result ofFalse
since6
is not part of the list.
- A list
-
In with Strings:
- A string variable
my_string
contains'Hello World'
. - The statement
'World' in my_string
checks for substring'World'
withinmy_string
, returningTrue
indicating the substring exists. - The statement
'world' in my_string
checks for substring'world'
inmy_string
, returningFalse
due to case sensitivity (Python is case-sensitive and ‘World’ is not the same as ‘world’).
- A string variable
-
In with Dictionaries:
- A dictionary
my_dict
is declared with two key-value pairs:'name': 'John'
and'age': 30
. - The statement
'name' in my_dict
checks if'name'
is a key inmy_dict
, returningTrue
. - The statement
'John' in my_dict.values()
inspects the values ofmy_dict
to determine if'John'
is among them, also returningTrue
.
- A dictionary
By dissecting these examples, the power and versatility of the ‘in’ keyword for membership operations across various data structures in Python are highlighted. This code serves as a hands-on demonstration, detailing how to utilize ‘in’ effectively in real-world scenarios.
FAQs on Exploring the Functionality of ‘in’ in Python: Membership Operations
-
What is the purpose of the ‘in’ keyword in Python?
The ‘in’ keyword in Python is used for membership testing. It checks if a value exists in a sequence, such as a list, tuple, or string. -
How does the ‘in’ keyword work in Python?
When you use the ‘in’ keyword, Python checks if the value on the left side of ‘in’ exists in the sequence on the right side. If the value is found, it returns True; otherwise, it returns False. -
Can the ‘in’ keyword be used with other data structures besides lists?
Yes, the ‘in’ keyword can be used with various data structures in Python, including tuples, strings, dictionaries, sets, and more. -
Is the ‘in’ keyword case-sensitive in Python?
Yes, the ‘in’ keyword is case-sensitive in Python. When using ‘in’ to check for membership in strings, it will distinguish between uppercase and lowercase letters. -
Are there any other membership operations similar to ‘in’ in Python?
Yes, apart from ‘in’, Python also provides the ‘not in’ operator, which checks for the absence of a value in a sequence. -
Can custom objects implement support for the ‘in’ operator in Python?
Yes, custom objects in Python can implement support for the ‘in’ operator by defining the__contains__
method in their class. -
How can I use the ‘in’ keyword effectively in Python programming?
To use the ‘in’ keyword effectively, practice using it with different data structures and explore its versatility in solving various programming tasks. -
Are there any performance considerations when using the ‘in’ keyword with large data structures?
Yes, when using the ‘in’ keyword with large data structures like lists, it’s important to consider the time complexity of the operation, especially for repeated membership checks.