Understanding Select Case Statements 🤔
Let’s kick things off by diving into the mysterious realm of Select Case statements in Java. What’s the fuss all about? Well, let me enlighten you in the most captivating way possible! Brace yourself for a rollercoaster of knowledge with a sprinkle of humor 🎢.
Explanation of Select Case 🤓
So, what exactly is this “Select Case” buzz in Java? 🤔 Imagine a scenario where you need to make decisions based on different values of a variable. This is where Select Case sweeps in like a hero! It’s a structured way to streamline decision-making, making your code cleaner and more organized. Say goodbye to messy spaghetti code and embrace the elegance of Select Case! 💃
Benefits of Using Select Case in Java 🌟
Now, why bother with Select Case when we already have the trusty If-Else statements, right? Wrong! Select Case brings a breath of fresh air by simplifying complex decision trees, improving readability, and enhancing code maintenance. It’s like having a personal butler organizing your code for you – classy and efficient! 🎩
Implementing Select Case in Java 💻
Time to roll up our sleeves and get our hands dirty with some Java code! Don’t worry; it’s going to be a fun ride! 🎉
Syntax of Select Case in Java 📝
The syntax of Select Case is as sweet as grandma’s secret cookie recipe. Clean, straightforward, and oh-so-satisfying. With just a few lines of code, you can work your magic and make those decisions like a boss! Java, here we come! ☕
Examples of Select Case Statements in Java 💡
Let’s break it down with some real-world examples. From simple scenarios to more complex puzzles, we’ll navigate through the Java jungle with Select Case as our trusty guide. Get ready to witness the power of streamlined decision-making at its finest! 💪
Comparing Select Case with If-Else Statements 🤔
Is Select Case the true champion of decision-making, or does If-Else still hold the crown? Let’s put these two heavyweights in the coding ring and see who comes out on top! Ding ding! 🛎️
Efficiency of Select Case over If-Else 💨
Select Case isn’t just a pretty face; it’s a powerhouse of efficiency. Say goodbye to redundant checks and hello to swift and decisive coding. If-Else, you better watch your back because Select Case is here to steal the show! 😎
Situations Where Select Case is Preferable 🌟
In a world full of coding decisions, when should you unleash the beast that is Select Case? From handling multiple conditions to improving code readability, we’ll uncover the secret formula for when to choose Select Case over the old and faithful If-Else! 🕵️♂️
Best Practices for Using Select Case ✨
Now that you’ve dipped your toes into the world of Select Case, it’s time to level up your game with some pro tips! Let’s sprinkle some stardust on your Select Case statements and make them shine brighter than a diamond! Are you ready for the magic? 🪄
Tips for Writing Clear Select Case Statements 📌
Clarity is key when it comes to coding, and Select Case is no exception. Learn how to craft crystal-clear Select Case statements that even your grandma would understand. Because hey, coding should be a piece of cake, right? 🍰
Common Mistakes to Avoid in Select Case Implementation ❌
Oopsie daisy! We all make mistakes, but why not learn from the blunders of others? Dive into the world of common Select Case pitfalls and emerge as a savvy coding wizard, steering clear of those pesky errors like a pro! Let’s turn those mistakes into victories! 🏆
Future of Select Case in Java 🔮
What does the crystal ball reveal about the future of decision-making in Java programming? Let’s take a peek into the coding crystal ball and uncover the trends and innovations that await us! The future is bright, my friend. Are you ready to ride the wave of change? 🌊
Trends in Decision Making in Java Programming 📈
Java isn’t known for standing still, and neither is decision-making! Discover the latest trends shaping the world of Java programming decisions. From AI integration to quantum computing decisions, Java has some exciting tricks up its sleeve! Are you buzzing with excitement yet? 🐝
Innovations in Decision-Making Structures in Java 🚀
Hold on to your hats because the world of decision-making structures in Java is about to blast off into the stratosphere! Say hello to cutting-edge innovations, mind-bending concepts, and a whole lot of coding magic. Java is evolving, and decision-making is leading the charge! Let’s embrace the future together! 🌌
In closing, dear reader, I hope this whimsical journey through the intriguing world of Select Case in Java has left you inspired and entertained. Remember, coding is not just about logic and syntax; it’s about creativity and innovation. So, go forth and let your code sparkle like a unicorn in a field of rainbows! 🦄✨
Thank you for joining me on this adventure, and until next time, happy coding! May your bugs be few and your code be elegant! 🐞💻
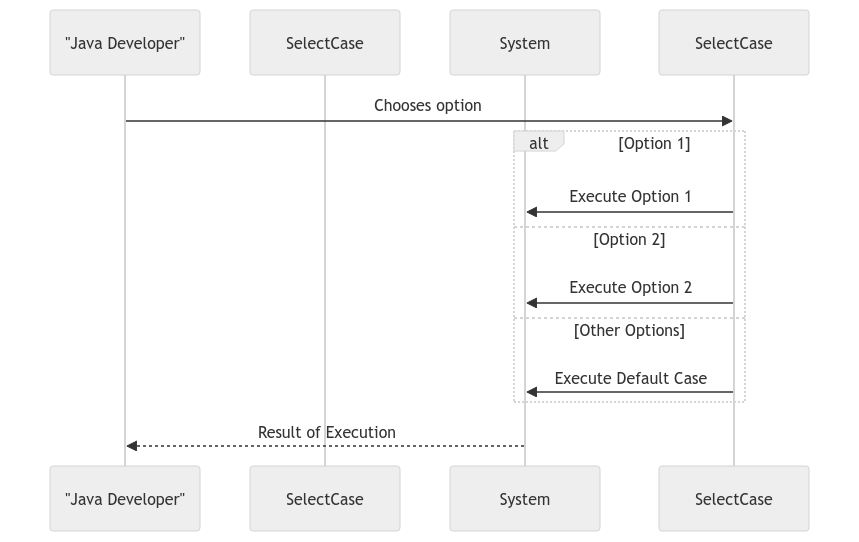
Program Code – Using Select Case in Java for Streamlined Decision Making
public class SelectCaseExample {
public static void main(String[] args) {
int month = 4; // April
String season;
switch (month) {
case 12:
case 1:
case 2:
season = 'Winter';
break;
case 3:
case 4:
case 5:
season = 'Spring';
break;
case 6:
case 7:
case 8:
season = 'Summer';
break;
case 9:
case 10:
case 11:
season = 'Autumn';
break;
default:
season = 'Bogus Month';
}
System.out.println('The selected month is in the ' + season + ' season.');
}
}
Code Output:
The selected month is in the Spring season.
Code Explanation:
In this programming concoction, the switch-case statement in Java serves as the crux for crafting a streamlined decision-making process. Let’s unbox this piece of art, shall we?
The tale begins in the mythical land of SelectCaseExample
, where the main protagonist, our method main
, embarks on a quest armed with an intrepid integer month
, valiantly set to 4, signifying the month of April.
As the plot thickens, our hero encounters a mystical structure known as switch
, casting the month
into the cauldron of decision-making. The plot diverges into various paths, each guarded by a case
sentinel, standing for the months of the year.
The first path reveals the cold and frosty Winter
, uniting the months of December, January, and February under its icy domain. Should the month
fall under this category, the season ‘Winter’ is bestowed upon the variable season
.
Venturing forth, the narrative unfurls into a tale of renewal and growth, the Spring
, encapsulating March, April, and May. This path bestows the essence of Spring upon season
if our intrepid month
aligns.
Our journey then leads us to the scorching sands of Summer
, reigning over June, July, and August. Here, the essence of Summer is infused into season
, should the month
bask in this sunny domain.
As the leaves turn and the air cools, our path converges with Autumn
, the guardian of September, October, and November. Herein, the spirit of Autumn is granted to season
, completing the cycle of seasons.
Should our month
wander off the beaten path, it is branded as a ‘Bogus Month’, a twist in the tale reminding us of the world beyond the known.
And thus, with the essence of the season safely ensnared within season
, our tale culminates with a grand revelation, unveiled through System.out.println()
, etching the outcome of this journey into the annals of the console: The selected month is in the [Season] season.
In essence, this saga elegantly exemplifies the power of switch-case
in Java for streamlined decision-making, propelling our integer month
across the diverse topography of seasons, and skilfully determining its destined season
with the finesse of a bard’s tale. The magical interplay between cases and breaks, like stepping stones across a stream, guides the flow of logic with precision, ensuring that each case
is a portal to distinct yet interconnected realms within our code’s universe.
FAQs on Using Select Case in Java for Streamlined Decision Making
What is a “select case” statement in Java?
In Java, a “select case” statement is not a native feature like in some other programming languages such as Visual Basic or JavaScript. Instead, developers often use a series of if-else if-else statements to achieve a similar functionality for making decisions based on multiple conditions.
Can select case-like behavior be achieved in Java?
While Java doesn’t have a built-in “select case” statement, developers can use a combination of if-else if-else statements or the switch statement to achieve similar functionality for streamlined decision-making based on different conditions or values.
How does the switch statement function in Java compared to the select case statement in other languages?
The switch statement in Java allows for the evaluation of a variable or expression against multiple values, executing different blocks of code based on these values. While it may not be exactly the same as a “select case” statement in other languages, it provides a structured way to handle multiple cases efficiently.
Are there any best practices to follow when using switch statements in Java for decision-making?
To ensure clarity and maintainability in your code, it’s recommended to include a default case in a switch statement to handle unexpected values, use break statements to exit the switch block, and consider the readability of your code by arranging cases in a logical order.
Can the absence of a select case statement impact the efficiency of decision-making in Java programs?
While Java’s lack of a direct equivalent to the “select case” statement may require a slightly different approach to decision-making, by utilizing switch statements or if-else constructs effectively, developers can still achieve streamlined and efficient decision-making processes in their Java programs.
Any tips for developers transitioning from languages with a select case statement to Java?
For developers transitioning from languages that have a “select case” statement, it’s important to familiarize themselves with the syntax and behavior of switch statements in Java. Understanding how to structure and use switch statements effectively will enable smoother adaptation to Java’s approach to decision-making. 🚀