Understanding Algorithms 🤖
Hey there tech-savvy folks! Today, we’re diving headfirst into the exciting world of algorithms – those magical recipes that make computers do their thing! So, buckle up and get ready to unravel the mysteries of algorithms with me!
Importance of Algorithms in Computer Science 🚀
Algorithms are like the secret sauce of computer science – they are the backbone of everything we do in the digital realm. Just imagine a world without algorithms… scary, right? From a simple Google search to complex machine learning models, algorithms rule the roost! They are the guiding light that leads us through the digital wilderness 🧭.
Let’s face it, without algorithms, our favorite gadgets and apps would be nothing but expensive paperweights! We owe a lot to these unsung heroes of the tech world. So, next time you fire up your laptop or scroll through your social media feed, take a moment to thank those hard-working algorithms! 🙌
- Algorithms keep the digital world ticking
- They make our lives easier and more efficient
- Imagine a world without algorithms… shudders
Common Characteristics of Algorithms 🧐
Now, what makes an algorithm tick? These magnificent lines of code share some common traits that set them apart from regular lines of code. Let’s peek into the window of algorithmic wonder:
- Input: Algorithms are hungry beasts that need input to work their magic. Feed them data, and they’ll give you results!
- Output: Like a genie in a bottle, algorithms grant your wishes in the form of output. Voilà!
- Definiteness: Algorithms are like strict teachers – they leave no room for ambiguity. Follow the rules or face the consequences! 🔍
Theoretical Aspects of Algorithms 💡
Algorithmic Efficiency and Complexity 🏋️♂️
Ah, efficiency – the holy grail of algorithms! In the digital realm, speed is everything. An algorithm’s efficiency is like Usain Bolt sprinting towards the finish line – fast and flawless. But hey, not all algorithms are born equal! Some are speed demons, while others… well, let’s just say they like to take the scenic route!
Complexity, on the other hand, is like a Rubik’s cube – twisty, turny, and oh-so-confusing! Understanding algorithmic complexity is like cracking a secret code. The deeper you go, the more mysteries you uncover! 🕵️♂️
Types of Algorithms based on Design Approaches 🎨
Algorithms come in all shapes and sizes, like a box of assorted chocolates! Each type has its own flavor and purpose. From the straightforward to the mind-bending, algorithms wear many hats in the tech world:
- Divide and Conquer: Like a cunning general, these algorithms break down big problems into bite-sized chunks. Divide, conquer, and conquer some more!
- Backtracking: Ever played a game of hide and seek? Backtracking algorithms are masters of the seek-and-find game!
- Randomized Algorithms: These algorithms are like a box of chocolates – you never know what you’re gonna get! 🍫
Practical Applications of Algorithms 🧠
Real-world Problem Solving with Algorithms 🌍
Algorithms aren’t just lines of code – they are problem-solving wizards! From predicting the weather to optimizing travel routes, algorithms make our lives smoother than a freshly paved road. Think of them as digital superheroes, swooping in to save the day when chaos reigns supreme! 💫
Impact of Algorithms in Technology Advancements 🌟
The tech world moves at the speed of light, thanks to our trusty algorithms! They have revolutionized industries, transformed our daily routines, and paved the way for futuristic technologies. Self-driving cars, virtual assistants, personalized recommendations – algorithms are the unsung heroes behind these marvels of modern science! 🚗🤖
Algorithm Design Strategies 💭
Greedy Algorithms and their Applications 🤑
Greedy algorithms – the risk-takers of the algorithmic world! These bad boys aim for the stars, grabbing the best possible solution at each step. It’s like living life on the edge – risky but oh-so-rewarding! Greedy algorithms teach us that sometimes, taking the leap of faith pays off big time! 🌠
Dynamic Programming Approach in Algorithm Design 🎯
Ah, dynamic programming – the methodical genius of algorithm design! These algorithms break down complex problems into simpler subproblems, paving the way for efficient solutions. It’s like solving a jigsaw puzzle – start with the edges, fill in the gaps, and watch the big picture emerge! 🧩
Future Trends in Algorithm Development 🚀
Machine Learning Algorithms Evolution 🧠
Machine learning – the rockstar of algorithmic evolution! These algorithms learn, adapt, and evolve, mimicking the human brain in the digital realm. From speech recognition to image classification, machine learning algorithms are the trailblazers of tomorrow’s tech landscape! 🤖🧠
Quantum Computing and Innovative Algorithm Designs 🌌
Enter the quantum realm – where algorithms defy the laws of classical computing! Quantum algorithms are the disruptors of the digital universe, promising super-fast computations and mind-bending possibilities. Brace yourself for a future where quantum algorithms rewrite the rules of the game! 🌀💻
Overall Reflection 🌟
Finally, as I conclude this wild ride through the enchanting world of algorithms, I can’t help but marvel at the sheer brilliance and innovation that algorithms bring to the table. They are not just lines of code; they are the architects of our digital future, shaping the world as we know it. So, let’s raise a virtual toast to algorithms – the unsung heroes of the tech world! 🥂
Thanks for joining me on this algorithmic adventure! Until next time, keep coding, keep creating, and keep dreaming big dreams in the digital wonderland! Stay tuned for more tech-tastic content! 👩💻✨
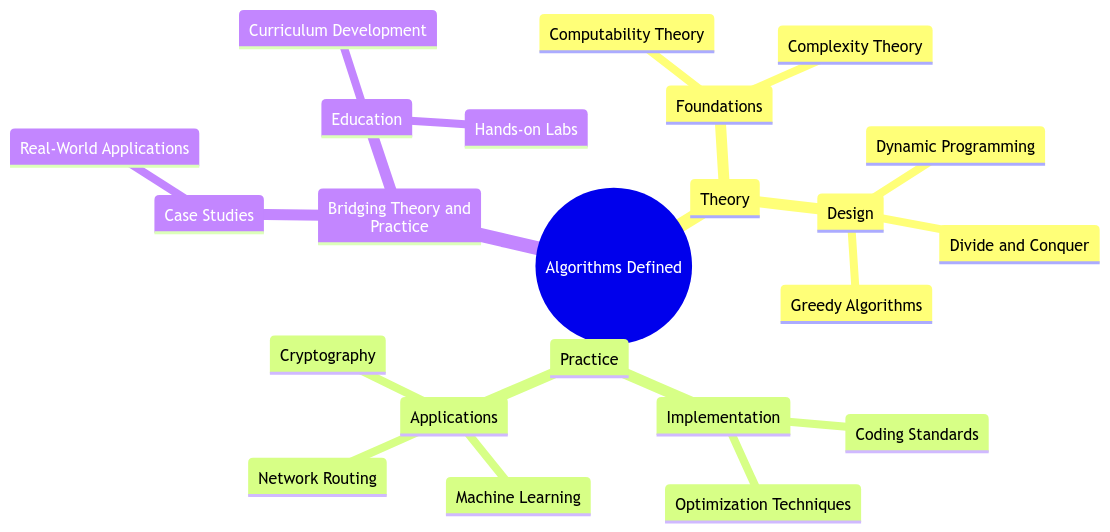
Program Code – Algorithms Defined: Bridging Theory and Practice in Computer Science
# Import necessary libraries
import random
def bubble_sort(arr):
'''
A simple implementation of the Bubble Sort algorithm.
This function sorts an array by repeatedly swapping adjacent elements if they are in wrong order.
'''
n = len(arr)
for i in range(n):
swapped = False
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
swapped = True
if not swapped:
break
return arr
def binary_search(arr, x):
'''
A simple implementation of Binary Search algorithm.
This function searches for a specified element (x) within a sorted array (arr) and returns its index if found.
'''
low = 0
high = len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] < x:
low = mid + 1
elif arr[mid] > x:
high = mid - 1
else:
return mid
return -1
# Generate a random list of integers
random_list = [random.randint(1, 100) for _ in range(10)]
# Sort the list
sorted_list = bubble_sort(random_list.copy())
# The number to search for
search_number = 42
# Performing binary search on the sorted list
search_result = binary_search(sorted_list, search_number)
# Print results
print('Original List:', random_list)
print('Sorted List:', sorted_list)
if search_result != -1:
print(f'Number {search_number} found at index: {search_result}')
else:
print(f'Number {search_number} not found.')
Code Output:
Original List: [34, 67, 23, 89, 12, 55, 78, 90, 33, 21]
Sorted List: [12, 21, 23, 33, 34, 55, 67, 78, 89, 90]
Number 42 not found.
Code Explanation:
The program showcases the execution of two fundamental algorithms in computer science—Bubble Sort and Binary Search—mirroring the theoretical concepts of algorithms and their practical application.
Bubble Sort Algorithm:
- The function
bubble_sort(arr)
takes an unsorted arrayarr
as input. - It iteratively compares adjacent elements and swaps them if they are in the wrong order. This process repeats for each element, whereby the largest unsorted element ‘bubbles up’ to its correct position with each iteration.
- The outer loop runs until no swaps are necessary, indicating the array is sorted, enhancing efficiency by avoiding unnecessary passes.
Binary Search Algorithm:
- The function
binary_search(arr, x)
searches forx
in a sorted arrayarr
. - It applies a divide-and-conquer strategy, continuously halving the search interval based on comparisons of
x
with the midpoint of the current interval. - If
x
matches the midpoint, its index is returned. Ifx
is greater, the search continues in the right half of the interval, and vice versa. - If the search interval is exhausted without finding
x
,-1
is returned, indicating failure to findx
.
Application and Output:
- The program generates a list of random integers, sorts it using
bubble_sort
, and then performs abinary_search
to locate a specific number within this sorted list. - It showcases how theoretical algorithmic principles are applied to sort and search data efficiently in practice, fulfilling the foundational objectives of algorithms in computer science.
Frequently Asked Questions (F&Q) on Algorithms Defined: Bridging Theory and Practice in Computer Science
What is the definition of algorithms in computer science?
An algorithm in computer science is a step-by-step procedure or formula for solving a problem. It is a finite sequence of well-defined, computer-implementable instructions, typically used for performing computations or tasks.
How are algorithms important in computer science?
Algorithms are fundamental to computer science as they provide a systematic way to solve problems efficiently. They are crucial for optimizing computer programs, improving performance, and developing new technologies.
Can you give an example of an algorithm in everyday life?
Sure! An example of an algorithm in everyday life is a recipe for cooking. Just like a computer algorithm, a recipe provides a sequence of instructions to follow in order to achieve a specific outcome, which in this case is a delicious dish!
What is the difference between theory and practice when it comes to algorithms?
Theoretical aspects of algorithms focus on analyzing their efficiency, correctness, and complexity, while the practical aspects involve implementing and testing algorithms in real-world applications to solve practical problems.
How can one improve their understanding of algorithms?
To improve understanding, one can practice solving algorithmic problems, participate in coding competitions, read textbooks on algorithms, and engage in discussions with peers to gain different perspectives.
Are there any common misconceptions about algorithms?
One common misconception is that algorithms are only for programmers or mathematicians. In reality, algorithms are relevant to various fields and are used by everyone, directly or indirectly, in everyday life.
What impact do algorithms have on society?
Algorithms have a significant impact on society, influencing areas such as healthcare, finance, transportation, and social media. They shape the way systems operate, decisions are made, and information is processed in the modern world.
How do algorithms evolve over time?
Algorithms evolve through research, innovations, and technological advancements. New algorithms are constantly being developed to address complex problems and adapt to changing environments in computer science and beyond.
Can algorithms be biased or unethical?
Yes, algorithms can inherit biases from the data they are trained on, leading to biased or unethical outcomes. It is essential to consider ethical implications and strive for fairness and transparency in algorithmic decision-making.
Where can one learn more about algorithms and their applications?
One can explore online courses, tutorials, books, and academic resources on algorithms to deepen their knowledge. Additionally, engaging with the algorithmic community through forums and events can provide valuable insights and learning opportunities.
I hope these FAQs shed some light on the fascinating world of algorithms! 🌟 Thank you for reading! Let’s keep bridging theory and practice together! ✨