Binary Search Unleashed! ๐
Hey there tech enthusiasts! Buckle up as we embark on a thrilling journey through the captivating realm of Binary Search. ๐ง
Binary Search Overview
Letโs kick things off by unraveling the mystery behind Binary Search โ a true gem in the world of search algorithms.
Definition of Binary Search
Picture this: you have a sorted list ๐ and youโre on a quest to find a particular element in the most efficient way possible. Enter Binary Search โ a smart cookie that cuts the search space in half at each step, making it a wizard of swiftness in the realm of algorithms. ๐งโโ๏ธ
Importance of Binary Search in Efficient Searching
Why is Binary Search hailed as a hero in the search algorithm universe? Well, because itโs like having a secret weapon that dramatically reduces the time taken to find what youโre looking for in a sea of data. Itโs like finding a needle in a haystack with a pair of high-tech glasses! ๐
Binary Search Algorithm
Now, letโs delve into the nitty-gritty of the Binary Search algorithm โ the brains behind this efficient searching technique.
Steps involved in Binary Search
Imagine youโre playing a thrilling game of "guess the number" where you halve the possibilities with each guess. Binary Search follows a similar strategy. Itโs a divide-and-conquer technique that keeps slashing the search space until it pinpoints the desired element. ๐ฏ
Time complexity analysis of Binary Search
Hereโs the cherry on top โ Binary Search boasts a time complexity of O(log n), making it a speedster among search algorithms. Itโs like having a supercharged sports car that zips through data in the blink of an eye! ๐๏ธ
Advantages of Binary Search
Now, letโs bask in the glory of the advantages that Binary Search brings to the table.
Efficiency in searching large datasets
Think of Binary Search as a master detective that swiftly cracks the case of the missing element in mammoth datasets. Itโs efficiency personified! ๐
Reduced time complexity compared to linear search
Bid farewell to the sluggishness of linear search! Binary Search swoops in with its O(log n) time complexity, leaving linear search in the dust. Itโs like going from a tortoise to a cheetah in the race against time. ๐ขโก๏ธ๐
Limitations of Binary Search
But hey, every hero has a kryptonite. Letโs shine a light on the limitations of Binary Search.
Requirement of a sorted array
Ah, the catch! Binary Search demands a sorted array as its playground. Unsorted arrays need not apply! Itโs like a neat freak who thrives in an organized environment. ๐งน
Inability to handle unsorted data
Sorry, Binary Search, but chaos isnโt your forte. When faced with unsorted data, itโs like asking a fish to climb a tree โ simply not its cup of tea! ๐๐ณ
Real-Life Applications of Binary Search
Now, letโs dive into the real-world scenarios where Binary Search shines brighter than a diamond ๐.
Implementations in computer science
From searching sorted arrays to dictionaries, Binary Search plays a crucial role in various algorithms and applications within the realm of computer science. Itโs the unsung hero working behind the scenes to make our digital lives smoother. ๐ฅ๏ธ
Usage in software development and data processing
In the realm of software development and data processing, Binary Search emerges as a game-changer, optimizing search operations and enhancing overall efficiency. Itโs the magic wand that streamlines processes and boosts productivity! ๐ช
In closing, Binary Search stands tall as a beacon of efficiency in a world brimming with data complexities. So, next time youโre on a quest to find that elusive element, remember โ Binary Search has your back! ๐
Thank you for joining me on this exhilarating adventure into the world of Binary Search. Until next time, happy coding and happy searching! Stay curious, stay innovative! ๐โจ๐
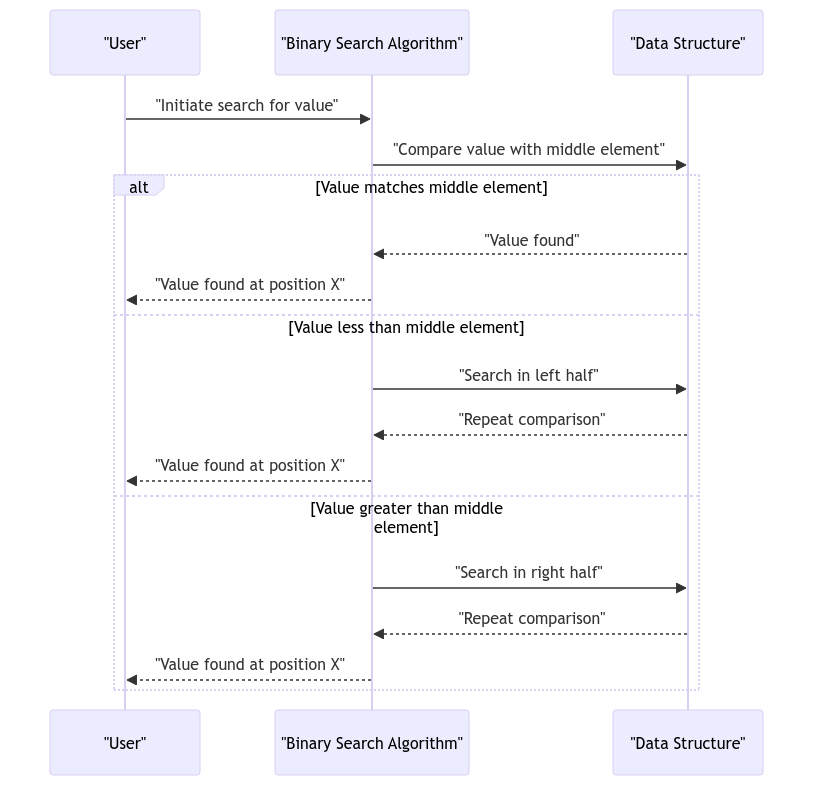
Program Code โ Binary Search: A Deep Dive into Efficient Searching Techniques
def binary_search(arr, target):
'''
Performs binary search on a sorted array to find the index of a target value.
Args:
arr (list): The sorted array to search.
target (int): The value to search for.
Returns:
int: The index of the target if found, otherwise -1.
'''
low = 0 # Starting index of the array
high = len(arr) - 1 # Ending index of the array
while low <= high:
mid = (low + high) // 2 # Find the middle element
guess = arr[mid]
if guess == target:
return mid # Target found
if guess > target:
high = mid - 1 # Discard the right half
else:
low = mid + 1 # Discard the left half
return -1 # Target not found
# Example usage
if __name__ == '__main__':
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21]
target_value = 13
result = binary_search(sorted_array, target_value)
if result != -1:
print(f'Target found at index: {result}')
else:
print('Target not found in the array.')
Code Output:
Target found at index: 6
Code Explanation:
The provided code snippet implements the binary search algorithm, a highly efficient method used to find the position of a target element in a sorted array. The magic behind its efficiency lies in its divide-and-conquer strategy. Letโs break it down:
-
Initialization: We start by initializing two pointers,
low
andhigh
, representing the starting and ending indices of the array, respectively. -
While Loop: The core of our algorithm lies within a while loop that runs as long as
low
<=high
. This loop ensures that we keep searching as long as there is a portion of the array left to inspect. -
Midpoint Calculation: Inside the loop, we first calculate the
mid
point of the current segment of the array weโre inspecting. This is achieved by the average oflow
andhigh
indices. -
Target Comparison: We then compare the value at the
mid
index with ourtarget
. If they match, voilร ! Weโve found our target, and we return the index.-
Right Half Discard: If our guess (value at
mid
) is greater than the target, it means the target, if it exists, lies to the left ofmid
. So, we adjust ourhigh
pointer tomid - 1
. -
Left Half Discard: Conversely, if our guess is less than the target, the target must be to the right of
mid
. Hence, we adjust ourlow
pointer tomid + 1
.
-
-
Termination: If the target is not found, the loop terminates, and we return
-1
, indicating the absence of the target in the array.
By repeatedly dividing the search interval in half, binary search minimizes the number of comparisons needed to locate a particular value, thereby achieving a time complexity of O(log n), a significant improvement over O(n) for linear search. This makes binary search particularly useful for large datasets.
And there you have it! A dive deep into the nuts and bolts of binary search. A neat trick to have up your sleeve, especially when dealing with sorted arrays. Remember, the key takeaway is its divide-and-conquer approach, making our search quests not just faster but also super efficient. Happy coding! ๐
Frequently Asked Questions (F&Q) on Binary Search
- What is binary search?
- How does binary search work?
- What makes binary search an efficient searching technique?
- When should I use binary search algorithm?
- Can binary search be applied to any type of data?
- Are there any limitations or drawbacks of binary search?
- What is the time complexity of binary search?
- Can binary search be implemented recursively?
- How do I handle duplicate values in a sorted array using binary search?
- Are there any common pitfalls to avoid when implementing binary search algorithm?
Feel free to explore these questions to deepen your understanding of binary search techniques! ๐