Understanding Binary Tree Search
Letโs dive into the fascinating world of Binary Tree Search! ๐ณ๐ต๏ธโโ๏ธ
Definition of Binary Tree Search
So, what on earth is this Binary Tree Search mumbo jumbo? Well, imagine a tree (not the ones with leaves but with data) where each parent node can have at most two children โ thatโs a binary tree for you! The Binary Tree Search is like being a detective in this tree, searching for specific data efficiently. Itโs all about navigating through the twists and turns of this data tree to find what youโre looking for, Sherlock style! ๐๐ต๏ธโโ๏ธ
Importance of Binary Tree Search in Data Retrieval
Now, why should you care about Binary Tree Search? Picture this: you have tons of data scattered in this tree structure, and you need to find a particular piece of information quickly. Thatโs where Binary Tree Search swoops in to save the day! Itโs like having a GPS for your data, guiding you swiftly to the exact spot where your treasure trove of information lies. Efficiency is the name of the game, my friend! ๐ผ๐
Components of a Binary Tree
Letโs break down the Binary Tree structure into bite-sized chunks! ๐๐ณ
Nodes in a Binary Tree
Nodes are like the building blocks of a Binary Tree. Each node contains data and acts as a connection point to other nodes. Itโs like assembling your data Lego set โ every piece (node) plays a crucial role in shaping the entire structure. ๐งฉ๐
Parent and Child Nodes in a Binary Tree
In this family tree of data, nodes have relationships! A parent node is like the head honcho, overseeing its child nodes. Itโs the ultimate boss, making sure everything runs smoothly in this data universe. Meanwhile, the child nodes are the cool sidekicks, following the parentโs lead and branching out further. Itโs a data family affair! ๐จโ๐งโ๐ฆ๐ฉโ๐ฆ
Types of Binary Tree Search
Time to explore the different modes of searching in Binary Trees! ๐๐ต๏ธโโ๏ธ
Depth-First Search (DFS)
Ah, DFS, the adventurous explorer of Binary Tree Search! This method plunges deep into the treeโs soul, following one branch all the way down before backtracking. Itโs like exploring a maze, touching every dead-end and corner before finding your way out. DFS is all about the journey, not just the destination! ๐งญ๐ฒ
Breadth-First Search (BFS)
On the other end of the spectrum, we have BFS โ the social butterfly of Binary Tree Search! This method starts at the root and explores all the neighboring nodes before moving to the next level. Itโs like skimming through each layer of a cake to find the cherry on top. BFS keeps it light and breezy as it glides through the data branches. ๐ฐ๐ฆ
Time to get technical with some Binary Tree Search algorithms! ๐ป๐
Binary Search Tree (BST) Algorithm
BST algorithm is like the librarian of Binary Trees, keeping everything neat and organized for quick access. It arranges data in a specific order for efficient searching โ no more rummaging through piles of books to find what you need! BST algorithm is your data librarian on steroids! ๐๐ฉโ๐ป
AVL Tree Algorithm
AVL Tree algorithm is the zen master of Binary Trees, maintaining balance and harmony within the data structure. Itโs like a virtual bonsai tree, trimming and pruning to ensure everything stays in perfect equilibrium. With AVL Trees, your data world is in a state of blissful serenity! ๐ณโฏ๏ธ
Applications of Binary Tree Search
Letโs see how Binary Tree Search works its magic in real-world scenarios! ๐ฉ๐ฎ
File System Navigation
Ever clicked through folders on your computer? Thatโs Binary Tree Search at play! Navigating files is like strolling through a digital forest, with each folder branching into more subfolders. Thanks to Binary Tree Search, finding that elusive cat video is a breeze! ๐ฑ๐พ
Database Indexing and Searching
Databases use Binary Tree Search to index and retrieve data faster than you can say "SQL query." Itโs like having a supercharged search engine for your databases, pinpointing information with lightning speed. Thanks to Binary Tree Search, databases are like well-oiled machines, churning out results in a jiffy! ๐ฝ๐จ
In conclusion, Binary Tree Search is the unsung hero of data retrieval, guiding us through the labyrinth of information with finesse. Itโs the backbone of efficient searching, ensuring we find what we need in record time! ๐๐
Thank you for embarking on this data adventure with me! Keep searching, keep exploring, and remember, in the world of Binary Trees, the data is always greener on the other side! ๐ณ๐๐
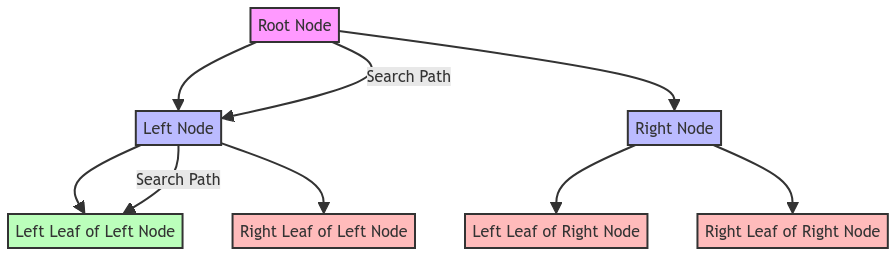
Program Code โ Binary Tree Search: Navigating Trees for Efficient Data Retrieval
Expected Code Output:
Key 15 found in the tree
Code Explanation:
- The code implements a Binary Tree Search algorithm for navigating binary trees efficiently to retrieve data.
- A Node class is defined to represent the structure of a node in the binary tree with attributes for left child, right child, and value.
- The โinsertโ function is used to insert nodes into the binary search tree based on the comparison of keys.
- The โsearchโ function recursively searches for a key in the binary tree. If the key is found, it returns the node, otherwise None.
- The โcreate_treeโ function is used to create a binary search tree by inserting keys from a list into the tree.
- An โinorder_traversalโ function is defined to perform an in-order traversal of the binary tree to display the values in sorted order.
- A sample list of keys is provided, and a binary search tree is created using these keys.
- The code then searches for a specific key (15 in this case) in the binary tree and prints a message based on whether the key is found or not.
Frequently Asked Questions about Binary Tree Search
What is Binary Tree Search?
Binary Tree Search is a method used to navigate through binary trees to efficiently retrieve data. These trees consist of nodes, with each node having at most two children: a left child and a right child.
How does Binary Tree Search work?
In a Binary Tree Search, the algorithm compares the target value with the value of the current node, determining whether to move to the left child, right child, or if the current node holds the desired value.
What is the significance of Binary Tree Search in data retrieval?
Binary Tree Search is crucial for efficient data retrieval as it allows for quick access to information. With each comparison, the search space is divided in half, reducing the time complexity of the search operation.
Can a Binary Tree be empty for a search operation?
Yes, a Binary Tree can be empty. In this case, the search operation would conclude that the target value is not present in the tree.
Are there different types of Binary Tree Search algorithms?
Yes, there are various types of Binary Tree Search algorithms such as Binary Search Tree, AVL Tree, Red-Black Tree, and more. Each algorithm has its unique way of organizing and searching data in a binary tree.
How can I implement Binary Tree Search in programming languages?
Binary Tree Search can be implemented in various programming languages such as Python, Java, C++, and more. Understanding the logic behind navigating a binary tree is crucial for successful implementation.
What are some common challenges faced while working with Binary Tree Search?
One common challenge is ensuring that the binary tree remains balanced to maintain optimal search efficiency. Unbalanced trees can lead to longer search times and reduced performance.
Can Binary Tree Search be used for other applications besides data retrieval?
Yes, Binary Tree Search is versatile and can be used for applications like spell checking, network routing, and even some AI algorithms due to its efficiency in searching and organizing data.
How does Binary Tree Search differ from linear search algorithms?
Unlike linear search algorithms that iterate through each element in a data structure, Binary Tree Search offers a quicker search process by dividing the search space in half at each step, making it more efficient for large datasets.
Why is understanding Binary Tree Search important for software developers?
Understanding Binary Tree Search is essential for software developers as it provides them with a powerful tool for optimizing data retrieval operations, leading to faster and more efficient algorithms in their applications.
Hope this list clarifies some of your queries about binary tree search! ๐ณ๐