In C Programming, bitwise operations are used to manipulate individual bits of data. Here are some examples of bitwise operators.
C Programming is a type of programming language that manipulates numbers by manipulating the bits in each word of the number. It allows programmers to perform mathematical and logical operations on the binary form of the number. Bitwise operators operate on individual bits of the number and have the same function as their binary counterparts, but their results can only be a 1 or 0. A bitwise AND operation is performed when the result of an operation must be 1 if the first operand has a 1 in the position indicated by the second operand and a 0 in all other positions.
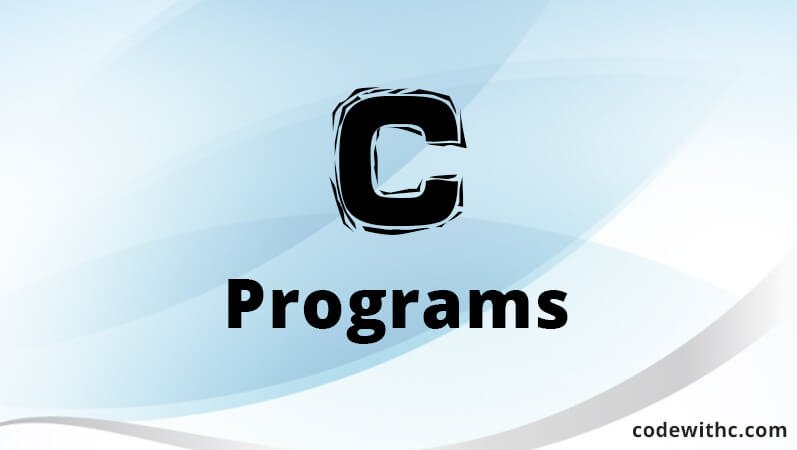
Similarly, a bitwise OR operation is performed if the first operand has a 1 in the position indicated by the second operand or if both operands have a 1 in the position indicated by the second operand, and the result is a 1 if either of these conditions are met. A bitwise XOR operation is performed if the first operand has a 0 in the position indicated by the second operand and a 1 in all other positions. The complement of a number is obtained by flipping all its bits. In a signed integer, this means that the last bit is set to a 1 and all the others are set to a 0. In a 32-bit integer, this means that the least significant bit is set to a 1 and the other 31 are set to a 0. Finally, a bitwise NOT operation can be performed on any integer to produce the inverse of the original number.
1 OR (bitwise)
Integer x = 6; / 0110b (0x06b) Integer y = 11; / 1011b (0x0b) Integer z = x | y; / 1111b (0x0f)
Cout "x = " c ", y = " y ", and z = " z endl; Result: x = 6, y = 11, z = 15.
The bitwise OR operator's truth table
True OR false = true True OR false = true False OR true = true False OR true = true
Integer x = 0 1 1 0
1 0 1 1 | Int y = 1 0 1 1
1 1 1 1 int z = 1 1 1 1 int z = 1 1 1 1 int
2 XOR (Bitwise)
Integer x = 6; / 0110b (0x06); Integer y = 11; / 1011b ( 0x0b) Integer z = x y ; / 1101b (0x0d)
Result: x = 6, y = 11, z = 13.
The bitwise XOR operator's truth table True XOR false = true
False XOR false = false False XOR true = false False XOR false = false False XOR false = false Integer x = 0 1 1 0
Int y = 1 0 1 1
Integer z = 1 1 0 1
3 AND (Bitwise)
Integer x = 6; / 0110b (0x06) Integer y = 11; / 1011b (0x0b) Integer z = x & y; / 0010 (0x02)
Cout "x = " c ", y = " y ", and z = " z endl; x = 6, y = 11, and z = 2 are the results.
The bitwise OR operator's truth table True AND False = False
False AND true = False False AND false = False True AND true = true Integer x = 0 1 1 0
& int y = 1 0 1 1
Int z = 0 1 0 0
4 Shift to the Right >>
/ 0010b Int x = 2
/0001b Int y = x >> 1 Result: a = 2, y = 1.
5.1.5 Shift
/ 0010b Int x = 2
/0100b Int y = x 1; Result: a = 2, y = 4