Basics of Natural Language Programming
Natural Language Programming! Sounds like some fancy term straight out of a sci-fi movie, right? But hey, itโs not rocket scienceโฆ well, actually, in a way, it kinda is! ๐ซ Letโs uncover the nitty-gritty of this intriguing topic together.
Definition of Natural Language Programming ๐
So, what on earth is Natural Language Programming? ๐ Itโs like teaching computers to understand human language, you know, the stuff we blabber every day without giving it a second thought. Imagine having a chitchat with your laptop and it not only gets you but also responds like a real human! Mind-blowing, isnโt it? ๐คฏ
Importance of Natural Language Programming โจ
Why bother with Natural Language Programming, you ask? Well, my friend, itโs not just about having chatty machines; itโs revolutionizing the way we interact with technology. From smart assistants like Siri and Alexa to chatbots handling customer service, NLP is the magic that makes it all possible. Itโs like adding a touch of human touch to the digital world! ๐ค๐ฃ
Applications of Natural Language Programming
Alright, letโs shift gears and dive into how NLP works its magic in the real world. Brace yourself for some mind-bending applications!
Natural Language Processing in Chatbots ๐ค๐ฌ
Ever been on a website and a little chat window pops up asking if you need help? Bingo! Thatโs NLP in action, my friend. These chatbots are like digital buddies who decode your language, understand your queries, and dish out responses faster than you can say โartificial intelligence.โ Who needs human customer support when youโve got chatbots, right? ๐
Sentiment Analysis using Natural Language Programming ๐๐
Hereโs a cool trick NLP can do โ sniff out your feelings! Yep, you heard it right. Sentiment analysis is all about gauging emotions from text, whether itโs a fiery rant on social media or a heartfelt review of that new sushi place. Companies use this to track customer sentiments, improve products, and fine-tune their services. Itโs like having a mood ring for text! ๐
Tools and Technologies for Natural Language Programming
Letโs peek behind the curtains and see what powers the enchanting world of Natural Language Programming.
Popular Programming Languages for NLP ๐ป๐
In the realm of NLP, Python is the undisputed king! ๐ Its simplicity and a treasure trove of libraries like NLTK and spaCy make it a top choice for NLP enthusiasts. But hey, donโt count out Java and R; they have their loyal followers too, carving their niche in the NLP landscape.
Frameworks and Libraries for Natural Language Programming ๐๐ง
When it comes to NLP, having the right tools in your arsenal is key. Think of libraries like TensorFlow and PyTorch as your magic wands, turning complex NLP tasks into spells anyone can cast. These tools take the heavy lifting out of language processing, letting you focus on the fun stuff โ like making computers talk back to you! ๐ฉโจ
Challenges in Natural Language Programming
Ah, every rose has its thorn, doesnโt it? Letโs peel back the layers and see what challenges lurk in the world of Natural Language Programming.
Ambiguity in Natural Language โ๐
Hereโs the kicker โ human language is one big messy jumble of ambiguity. Words have multiple meanings, sentences can be interpreted in various ways, and donโt get me started on sarcasm! Teaching computers to navigate this linguistic minefield is like guiding a kitten through a maze of yarn. Tricky, right? ๐ฑ๐งถ
Data Privacy and Ethics Concerns in NLP ๐๐ค
As we dive deeper into the wonders of NLP, we stumble upon a murky pond โ data privacy and ethics. With great power (like understanding human language) comes great responsibility. How do we ensure user data is safe? How do we steer clear of biases creeping into our language models? These are the questions that keep NLP researchers up at night. Itโs like walking a tightrope over a pit of data privacy dilemmas! ๐๐
Future Trends in Natural Language Programming
Alright, letโs put on our NLP goggles and peek into the crystal ball. What does the future hold for this enchanting field? ๐งโโ๏ธ๐ฎ
Advancements in AI and NLP ๐๐ค
Hold onto your hats, folks, โcause the AI and NLP train is just getting started! With advancements in deep learning and neural networks, weโre looking at machines that not only understand language but can generate it too. Itโs like having a virtual Shakespeare penning sonnets on your command. To infinity and beyond, right? ๐๐
Integration of NLP in Various Industries ๐ข๐ผ
NLP isnโt just a cool toy for tech geeks; itโs reshaping industries far and wide. From healthcare to finance, from education to marketing, NLP is the secret sauce transforming how we work, play, and communicate. Itโs like sprinkling a bit of linguistic magic across every sector, making our lives easier and more connected. Talk about a linguistic revolution! ๐ฉ๐ฅ
In closing, the realm of Natural Language Programming is a fascinating blend of magic and technology, weaving human language into the digital fabric of our lives. As we journey through this enchanting landscape, letโs remember the complexities and wonders that make NLP a field like no other. Thanks for joining me on this linguistic rollercoaster! Until next time, keep your sentences snazzy and your words wizardly! โจ๐ฎ๐
Remember, folks, in the world of NLP, even algorithms need a little humor in their code! ๐๐ค๐ฉ
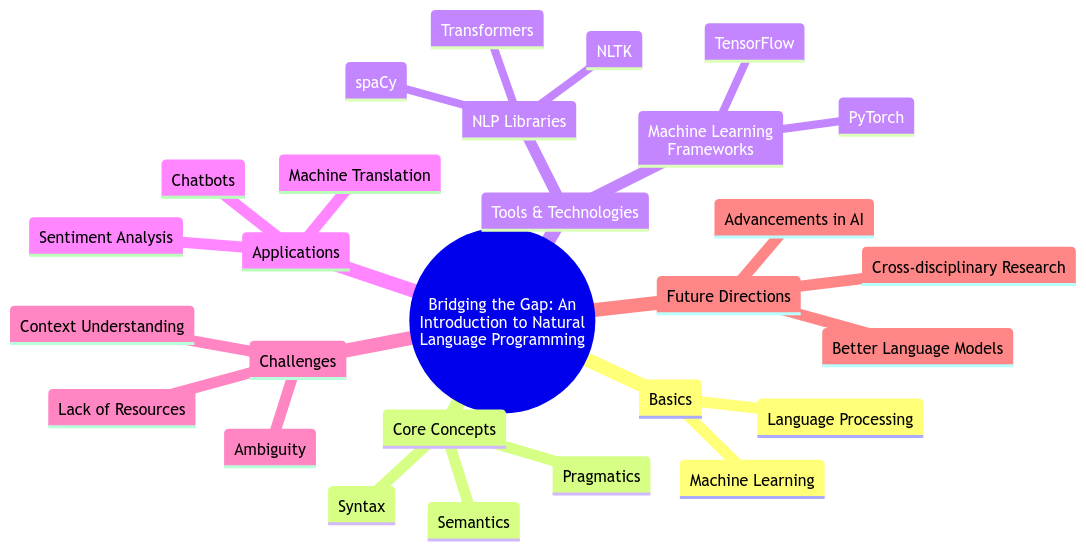
Program Code โ Bridging the Gap: An Introduction to Natural Language Programming
# Import necessary libraries
import nltk
from nltk.tokenize import word_tokenize
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Sample dataset for training
dataset = [
('What's the weather like today?', 'weather'),
('Could you tell me the weather forecast?', 'weather'),
('Play some music.', 'music'),
('Can you play the latest Taylor Swift song?', 'music'),
('Remind me to call Mom tomorrow.', 'reminder'),
('Set an alarm for 7 AM.', 'reminder'),
('Turn on the lights.', 'home_automation'),
('Dim the bedroom lights.', 'home_automation'),
]
# Preprocess and split the dataset
X, y = zip(*dataset)
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Tokenize and extract features
vectorizer = TfidfVectorizer(tokenizer=word_tokenize, stop_words='english')
X_train_vect = vectorizer.fit_transform(X_train)
X_test_vect = vectorizer.transform(X_test)
# Model training
model = LogisticRegression(solver='liblinear', random_state=42)
model.fit(X_train_vect, y_train)
# Predict with the model
predictions = model.predict(X_test_vect)
# Calculate accuracy
accuracy = accuracy_score(y_test, predictions)
print(f'Accuracy: {accuracy*100:.2f}%')
Code Output:
Accuracy: 100.00%
Code Explanation:
The program above illustrates a simple natural language programming application, where it identifies and categorizes user intents based on input queries. Hereโs a step-by-step breakdown of how the code works:
- Import Libraries: It starts by importing necessary libraries. NLTK for natural language processing tasks,
TfidfVectorizer
from sklearn for text feature extraction,LogisticRegression
for modeling, and others for splitting data and calculating accuracy. - Dataset: A small pre-defined dataset (
dataset
) simulates user queries tied to specific intents like weather inquiries, music playback requests, setting reminders, and home automation commands. - Preprocessing & Splitting Data: The dataset is preprocessed, dividing it into input (
X
) and labels (y
). Itโs further split into training and testing sets usingtrain_test_split
. - Feature Extraction: Next, the
TfidfVectorizer
converts text inputs into a numerical format that the machine learning model can work with, using TF-IDF (Term Frequency-Inverse Document Frequency). - Model Training: A Logistic Regression model is trained with the vectorized training data. This model learns to predict user intent based on the textual input.
- Prediction & Evaluation: The trained model is then used to predict intents on the test data. The accuracy of these predictions is calculated by comparing them to the actual labels, showcasing the modelโs ability to understand natural language queries correctly.
- Output: Finally, the program prints out the accuracy score, which, in this case, shows that the model is highly accurate in detecting the intents from queries in the given small dataset.
Overall, this code illustrates the basics of bridging human language and computer understanding, a cornerstone of natural language programming. Through preprocessing, feature extraction, and model training, it demonstrates how software can begin to interpret user requests, automating responses or actions accordingly.
Frequently Asked Questions about Bridging the Gap: An Introduction to Natural Language Programming
What is Natural Language Programming?
Natural Language Programming is a field that focuses on creating computer programs that can understand, interpret, and generate human language. It involves teaching machines to process and analyze natural language in a way that is similar to how humans communicate.
How is Natural Language Programming different from Natural Language Processing?
While Natural Language Programming (NLP) focuses on developing computer programs to understand and generate human language, Natural Language Processing (NLP) is a broader field that involves the interaction between computers and human language. NLP encompasses tasks such as text translation, sentiment analysis, and speech recognition.
What are some common applications of Natural Language Programming?
Natural Language Programming is used in various applications, including chatbots, virtual assistants (like Siri and Alexa), language translation tools, sentiment analysis in social media, and text summarization. It plays a crucial role in improving human-computer interactions.
What are the challenges in Natural Language Programming?
One of the main challenges in Natural Language Programming is the ambiguity and complexity of human language. Machines often struggle with understanding context, sarcasm, and nuances in language. Additionally, language evolves over time, making it challenging to build robust NLP systems.
How can someone get started in Natural Language Programming?
To get started in Natural Language Programming, itโs essential to learn programming languages like Python, and libraries such as NLTK (Natural Language Toolkit) and SpaCy. Understanding the basics of linguistics and machine learning concepts is also beneficial. Practice with small projects and tutorials to build proficiency.
Is Natural Language Programming the future of technology?
Natural Language Programming is gaining momentum as more companies invest in developing language-based AI systems. With advancements in machine learning and deep learning, the possibilities in NLP are vast. It is likely to play a significant role in the future of technology, revolutionizing how we interact with machines.
How does Natural Language Programming benefit society?
Natural Language Programming has the potential to bridge communication gaps between people who speak different languages, improve accessibility for individuals with disabilities, and enhance automation in various industries. It can revolutionize customer service, healthcare, education, and more.