This tutorial will show how to create a random file and enter some data into it in C++.
We will create a new file and we will enter some lines of text into it. There are several different types of random files. These include: The structure random file is structured, and the content of the random file is written by structures.
One of the main benefits of using structures is that we can easily access any information in a given file, including any of its structures.
Command-line arguments give you the ability to pass a string value from the command line to the program. The argument is usually a filename or path.
#include
#include
#include
struct data{
char str[ 255 ];
};
void main (int argc, char* argv[])
{
FILE *fp;
struct data line;
fp = fopen (argv[1], "wb");
if (fp == NULL) {
perror ("An error occurred in creating the file\n");
exit(1);
}
printf("Enter file content:\n");
gets(line.str);
while(strcmp(line.str, "stop") !=0){
fwrite( &line, sizeof(struct data), 1, fp );
gets(line.str);
}
fclose(fp);
}
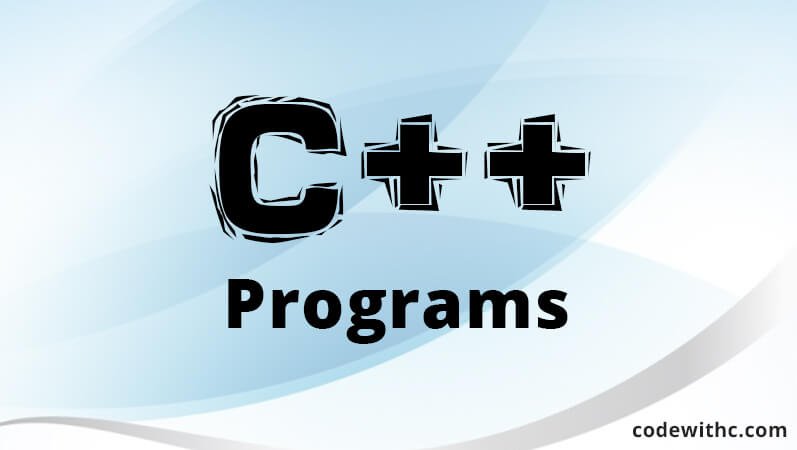
Let’s first define a structure using the “data” word as its name, which contains a string variable called “str,” that is a length of 255 characters.
We’ll then define a file pointer fp, which will point to a line of text, so the line becomes a structure containing a string, which is called str.
We will open a file named “test.txt” for writing, but we will only modify that file, leaving the original alone.
If the file is not compatible with write remote-only mode, it will not be opened. An error message will be displayed and the program will close.
You will be prompted to enter the file contents. You can assign the contents of the file to the variable str. Stop indicates the end of a field. So it compares what you have entered with what is stored in the stop field. Stop being entered into the file that we’re pointing at.
Because it is a random file, the text is written into the file through the structure line. fwrite is a function that writes the number of bytes equal to the size of the structure line into the file pointed at by the fp pointer at its current position.
This sentence has a member of the line structure and is being written into the file. The file pointed at by the file pointer is the input file, the fp file pointer is closed