Printing the solution of Tower of Hanoi is a well-known problem in C programming language, and its solution using recursive function is very popular. In this post, the source code in the C program for Tower of Hanoi has been presented in two different ways of programming, with a sample output screen common to both of them. For the sake of simplicity in understanding the code, and for making the code more user-friendly, I have added multiple comments in the program source code.
Before going through the program for Tower of Hanoi in C, here, I’d like to give a brief introduction to Tower of Hanoi and the working procedure of the C source code for this puzzle. Tower of Hanoi is a mathematical puzzle with three rods and ‘n’ numbers of discs; the puzzle was invented by the French mathematician Edouard Lucas in 1883. The objective of this puzzle is to transfer the entire stack to another rod.
Rules of Tower of Hanoi:
- Only a single disc is allowed to be transferred at a time.
- Each transfer or move should consist of taking the upper disk from one of the stacks and then placing it on the top of another stack i.e. only a top most disk on the stack can be moved.
- A larger disk cannot be placed over a smaller disk; the placing of disk should be in increasing order.
How does Tower of Hanoi in C work?
The source code for solving Tower of Hanoi in C is based on recursion. So, the key to solving this puzzle is to break the problem down into a number of smaller problems and further break these into even smaller ones, so that it is made a typical best-suited problem for the application of the recursive function.
The algorithm or the working procedure, which is to be repeated for a finite number of steps, is illustrated below:
A, B and C are rods or pegs and n is the total number of discs, 1 is the largest disk and 5 is the smallest one.
– Move n-1 discs from rod A to B which causes disc n alone in on the rod A
– Transfer the disc n from A to C
– Transfer n-1 discs from rod B to C so that they sit over disc n
Tower of Hanoi in C Using Recursion:
/* C program for Tower of Hanoi*/
/*Application of Recursive function*/
#include <stdio.h>
void hanoifun(int n, char fr, char tr, char ar)//fr=from rod,tr =to rod, ar=aux rod
{
if (n == 1)
{
printf("\n Move disk 1 from rod %c to rod %c", fr, tr);
return;
}
hanoifun(n-1, fr, ar, tr);
printf("\n Move disk %d from rod %c to rod %c", n, fr, tr);
hanoifun(n-1, ar, tr, fr);
}
int main()
{
int n = 4; // n immplies the number of discs
hanoifun(n, 'A', 'C', 'B'); // A, B and C are the name of rod
return 0;
}
The aforementioned source code of this puzzle is the outcome of application of recursive function. In the program source code, hanoifun() is the recursive function with four arguments, namely – n, fr, tr and ar. “n” is of integer data type and the other three variables are of character data type.
In this C program for Tower of Hanoi, the objective of defining n is to store numbers of discs, and the other character variables fr,tr and ar stand for from rod, to rod and auxiliary rod, respectively. There is nothing to be provided as input in this program. Copy the source code in Code::Blocks and run it.
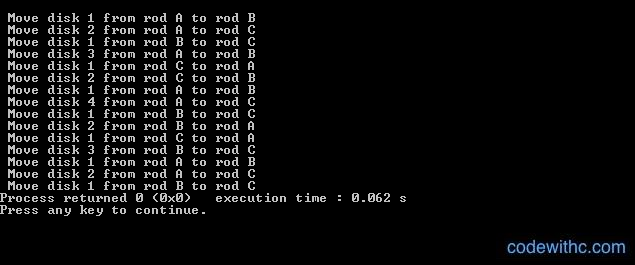
Alternative C source code for Tower of Hanoi puzzle is given below. It is almost similar to the above one, except that in this code, you can input the number of discs.
/* C program for Tower of Hanoi*/
#include <stdio.h>
void towerfun(int, char, char, char);
int main()
{
int n;// defined to store the number disc
printf("Enter the number of disks : ");
scanf("%d", &n);
printf("The sequence of moves involved in the Tower of Hanoi are :\n");
towerfun(n, 'A', 'C', 'B'); // A, B, C are tower
return 0;
}
void towerfun(int n, char fr, char tr, char ar)
{
if (n == 1)
{
printf("\n Move disk 1 from rod %c to rod %c", fr, tr);
return;
}
towerfun(n - 1, fr, ar, tr);
printf("\n Move disk %d from rod %c to rod %c", n, fr, tr);
towerfun(n - 1, ar, tr, fr);
}
Also see,
Tower of Hanoi Algorithm/Flowchart
Pascal’s Triangle C Program
Fibonacci Series C Program
Do have any other alternative (completely different) source code for Tower of Hanoi in C? If yes, feel free to share them with us. The source codes provided here have been coded perfectly, and are error-free. Your feedback and queries relevant to this topic can be discussed in and brought directly to us from the comments box below.