In a C++ program, the stack memory is used for local variables that are created and destroyed in a function. The stack memory grows downward. When a function returns, its local variables go back to the place they came from.
C++ is a versatile language and it has been used by almost everyone. The stack memory model is a very important concept in the C++ programming language.
The C++ stack memory model is a way of representing the state of a program’s memory during execution. A stack is a data structure that stores data in a last-in-first-out fashion. The stack is used to represent the current state of a program, so it is important to understand how it works.
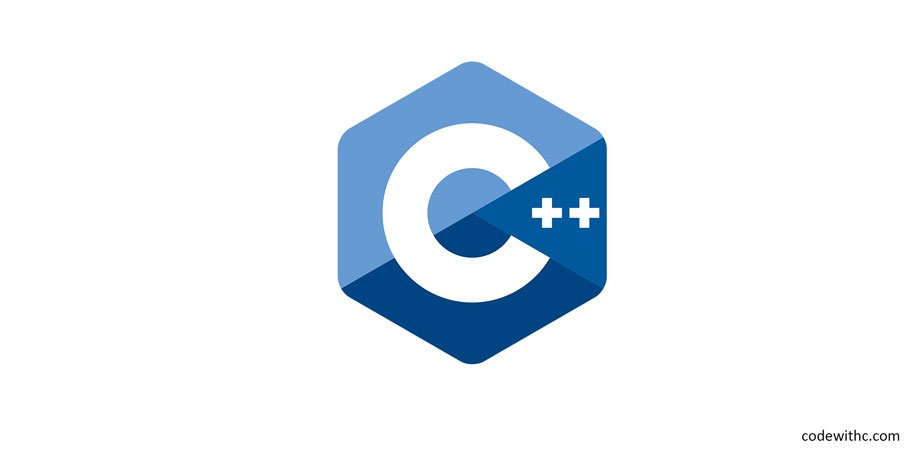
What is the stack in C++?
Stack means a collection of data that is placed in memory as per the sequence. Stack is used to store data while the program is running. When the program is started, it starts with the top of the stack, so it is called as the ‘top of the stack’.
Stack Memory Model
In the C++, the stack memory model is used by the compiler to decide where the values will be stored in memory. There are two types of stack memory models in the C++, which are called the normal and the non-normal stack memory model.
Normal Stack Memory Model
When you run a program in the normal stack memory model, the compiler places the variables of the stack in the same order in which they were declared. If the variables of the stack are not placed in the same order, the data will be corrupted.
Non-Normal Stack Memory Model
In this type of memory model, the compiler does not follow the order of the variables. If you use the non-normal stack memory model, then the compiler will place the variables in any order that he wants.
Normal and Non-Normal Stack Memory Model
If the normal stack memory model is used, then the variables will be stored in the following way:
- First, the local variable is placed on the stack.
- Next, the return value is placed.
- After that, the parameters are placed.
- Finally, the main function is placed.
If the non-normal stack memory model is used, then the variables will be stored in the following way:
- First, the local variable is placed on the stack.
- Next, the return value is placed.
- After that, the parameters are placed.
- Finally, the main function is placed.
The C++ compiler has the ability to choose the type of stack memory model. But it is not compulsory to use the normal stack memory model. You can use the non-normal stack memory model also.
The stack is used for two main reasons: to handle exceptions and to keep track of variables.
Why Use A C++ Stack?
A stack is a data structure that is used to store information. It is the last-in-first-out method of storing data. That means that the data is added to the end of the data structure and is accessed in the reverse order. This is done to ensure that the data is in the correct order.
The C++ stack memory model is used in programming languages such as C and C++. Because it is used in programming languages, it is important to understand how the stack is used in these languages. For example, in C, the stack is used to handle exceptions. If a program encounters an exception, it stops executing and goes into a special state called an exception handler. When the exception is handled, control is returned to the place where the exception was encountered.
Memory Management
When a C++ program executes, a stack is created to represent the current state of the program. The stack is used to represent the current state of the program because it is the only way to store information. The C++ stack is a last-in-first-out data structure. This means that data is added to the end of the data structure and is accessed in the reverse order.
When a C++ program executes, the stack is used to manage memory. It is used to store information that is needed when the program runs. Information is stored in the stack in a last-in-first-out fashion. When a program needs to access data, it moves the data from the stack to memory. Once the data is moved to memory, the program can access the data in the memory.
The C++ stack is used to represent the current state of the program. It is used to handle exceptions and to keep track of variables. When a C++ program executes, a stack is created to represent the current state of the program. This stack is used to represent the current state of the program because it is the only way to store information.
- The C++ stack is a last-in-first-out data structure. This means that data is added to the end of the data structure and is accessed in the reverse order. This is done to ensure that the data is in the correct order.
- A C++ stack is used to store information that is needed when the program runs. Information is stored in the stack in a last-in-first-out fashion. When a program needs to access data, it moves the data from the stack to memory. Once the data is moved to memory, the program can access the data in the memory.
- The C++ stack is used to manage memory. It is used to store information that is needed when the program runs. Information is stored in the stack in a last-in-first-out fashion. When a program needs to access data, it moves the data from the stack to memory. Once the data is moved to memory, the program can access the data in the memory.
- The C++ stack is a last-in-first-out data structure. This means that data is added to the end of the data structure and is accessed in the reverse order. This is done to ensure that the data is in the correct order.
Example: Simple code that we then use to explain how the stack operates
void function2(int variable1)
{
int variable2{ variable1 };
}
void function1(int variable)
{
function2(variable);
}
int _tmain(int argc, _TCHAR* argv[])
{
int variable{ 0 };
function1(variable);
return 0;
}
It’s very easy to stack space for main. There is a single storage space for a variable. A new stack frame is created on top of the existing frame for _tmain.
Conclusion:
The C++ compiler has the ability to choose the stack memory model, but it is not compulsory to use the normal stack memory model. You can use the non-normal stack memory model also.