C++ Program to find the area of a triangle when its sides are given.
The area of a triangle is a basic topic of mathematics. We all know that a triangle has three sides athe nd area of a triangle is equal to the base times height. The area of a triangle is defined as A = bbh where b stands for base and height. To calculate the area of a triangle we need three sides of the triangle.
C ++ Program to find the area of a triangle
In this tutorial, I will discuss how to find the area of a triangle using a C++ program. C++ program is very helpful for students and professionals who want to practice their programming skills and get better grades.
# include
#include
# include
#include
using namespace std;
int main( )
{
int a,b,c;
float s, area;
cout<<"enter there sides of the triangle"; cin>>a>>b>>c;
if((a+b)<c||(b+c)<a||(a+c)<b)
cout<<"finding area is not possible";
else s=(a+b+c)/2;
area=sqrt(s*(s-a)*(s-b)*(s-c));
cout<<"area="<<area;
return 0;
}
Now we are going to learn how to find the area of a triangle when the sides are given using C++. This is the C++ program to find the area of a triangle when the sides are given
#include
using namespace std;
int main()
{
int a, b, c;
cout<<"Enter three numbers"<<endl;< p=""></endl;<>
cin>>a>>b>>c;
cout<<"The area of the triangle is"<<endl;< p=""></endl;<>
cout<<"\ta^2 + b^2 + c^2 = "<<a*a+b*b+c*c<<endl;< p=""></a*a+b*b+c*c<<endl;<>
return 0;
}
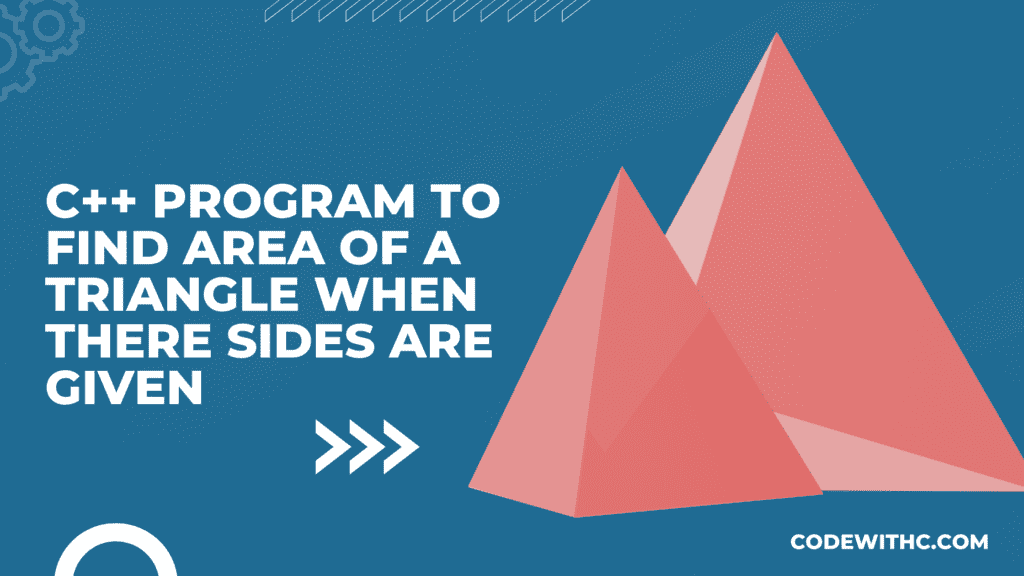
C++ Program: Area of a Triangle When its sides are Given
We can use the formula given below to find the area of a triangle when its sides are given.
Let ABC be a triangle. Then,
Area =
The area of a triangle is nothing but the sum of the areas of two triangles. The formula is very easy to understand and can be used in different situations. You can use this method to find the area of a triangle when you know the length of the sides. If the lengths of the sides are given, then we can also calculate the area of a triangle using this method. Here is a C++ program that will help you to find the area of a triangle.
#include
using namespace std;
int main()
{
//Enter the length of the side
double a,b,c;
cin>>a>>b>>c;
double area;
if(b*c==a*c+a*b)area=sqrt(a*a*(a/2.0));
else if((a+b)*(a+c)==a*b*c)area=a*sqrt((b*a)/2.0+c*(c*a)/2.0);
else if((b+c)*(a+c)==b*a*c)area=sqrt((b*a)*(b*a)/2.0+c*(c*a)*(c*a)/2.0);
else area=0;
cout<<"The area of the triangle is "<<area<<endl;< p=""></area<<endl;<>
return 0;
}