How to declare a function inside the structure of c programming. Declaring a function inside a structure is not possible in C language but it is possible in C++. In order to declare a function within a structure, you need to write the definition of the function before declaring the structure.
We all know that declaring a function is very important and without declaring a function you can’t call the function.
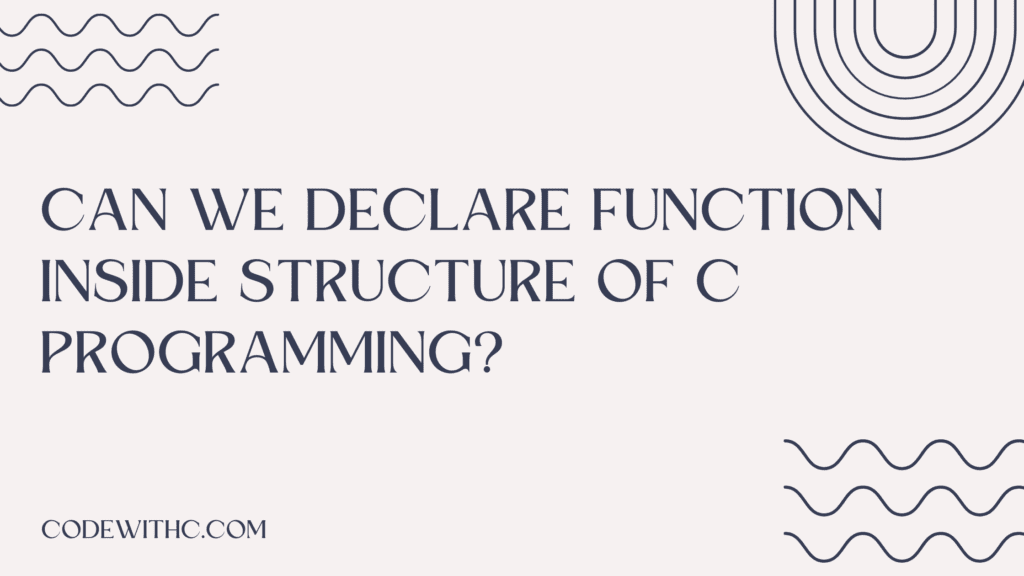
Here is a simple example of how to declare a function inside the structure.
Example – 1
struct student{
int roll_no;
char name[20];
double marks;
} student1;
void display_details(student s){
printf("Roll no: %d\n",s.roll_no);
printf("Name: %s\n",s.name);
printf("Marks: %lf",s.marks);
}
int main(){
display_details(student1);
}
Output:
Roll no: 7092
Name: Ara
Marks: 9.1
Example – 2
#include
struct employee
{
char name[10];
int age;
};
int main()
{
employee emp[2];
printf("Enter name: ");
scanf("%s",emp[0].name);
emp[0].age = 30;
emp[1].age = 18;
return 0;
}
Output:
Enter name: Brandi
Brandi30
Brandi18
Now, it is very simple to declare a function inside the structure but the problem is that we need to use the structure variable as the pointer of the structure.
Example – 3
#include
struct employee
{
char name[10];
int age;
};
int main()
{
employee emp[2];
printf("Enter name: ");
scanf("%s",emp[0].name);
emp[0].age = 30;
emp[1].age = 18;
return 0;
}
Error: Cannot convert ’employee’ to ‘int’ in assignment
So, what is the solution to this error?
Solution: Declare the function as follows:
#include
struct employee
{
char name[10];
int age;
void function(int *ptr, int n)
{
*ptr = n;
}
};
int main()
{
employee emp[2];
printf("Enter name: ");
scanf("%s",emp[0].name);
emp[0].age = 30;
emp[1].age = 21;
function(&emp[0],30);
return 0;
}
Output:
Enter name: Brandi
Brandi30
Brandi18
We have already learned how to declare a function in a standalone program. So, this is not a new concept. But if you want to use this concept inside the structure, then you need to write the function before defining the structure.
Conclusion:
I hope you liked this post about how to declare a function inside the structure of C. As you can see, the function is declared before the structure, because you can’t define the function inside the structure.