π The Hilarious Guide to Data Structures π
Ah, data structures β the unsung heroes of the programming world! π€ Today, weβre diving headfirst into the amusement park of Data Structures. Hold onto your hats (or should I say, your coding hats) as we navigate through the exhilarating world of organizing information for efficient access and modification.
Overview of Data Structures
Alright, folks, letβs start this rollercoaster ride with a quick overview of data structures. Imagine data structures as the magical wardrobes that programmers use to organize and store their data efficiently. They are like the Marie Kondo of programming β sparking joy by keeping everything neat and tidy! π§Ή
Importance of Data Structures
Now, why should we care about these fancy-schmancy data structures? Well, buckle up because hereβs the deal β efficient data structures can make or break your code performance. They are the secret sauce behind fast algorithms, ensuring your programs run smoother than a jazz pianistβs fingers on the keys. π
Common Types of Data Structures
Hold onto your butts, itβs time to explore the common types of data structures that will make your programming heart skip a beat!
- Array Data Structure
- Linked List Data Structure
- Tree Data Structure
- Hash Table Data Structure
Array Data Structure
Letβs kick things off with the notorious Array Data Structure. Picture an array like a gigantic buffet table where you can neatly line up your data. Itβs like organizing your sock drawer β but for numbers and strings! π§¦
Definition and Characteristics
An array is like a treasure chest where you can stuff all your goodies in one place. Itβs a simple yet powerful data structure that lets you store elements of the same data type in contiguous memory locations. Itβs like having a row of lockers β each holding a different item!
Operations on Arrays
Now, how do we play with these arrays? Well, you can do all sorts of fun stuff β adding elements, removing elements, or even shuffling them around. Itβs like being a magician, waving your wand (or should I say, your keyboard) and making the elements dance to your tune! β¨
Linked List Data Structure
Next stop β the Linked List Data Structure! Imagine a train with carriages holding your data β thatβs a Linked List for you! π
Singly Linked List
In a Singly Linked List, each element points to the next one, forming a chain of data. Itβs like a conga line at a party β each person holding hands with the next, moving as one cohesive unit! π
Doubly Linked List
Now, letβs introduce the Doubly Linked List β the fancy cousin of the Singly Linked List. In this version, each element points both to the next and the previous element, creating a bidirectional data dance floor! Itβs like having eyes in the back of your head β you can see in both directions! π
Tree Data Structure
Branching out now to the Tree Data Structure β natureβs way of organizing information in a hierarchy. Imagine a family tree, but for your data! π³
Binary Tree
In a Binary Tree, each node can have at most two children β a left child and a right child. Itβs like playing a game of 20 questions β making binary decisions at each step to find the treasure at the end! π
Binary Search Tree
Now, the Binary Search Tree takes it up a notch! Itβs like a well-organized library where each element knows exactly where it belongs. No more searching through stacks of books β just follow the tree branches to your data destination! π
Hash Table Data Structure
Last but not least, we have the Hash Table Data Structure β the Sherlock Holmes of data retrieval! π΅οΈββοΈ
Hashing Function
Hashing functions are like secret codes that transform your data into unique fingerprints. Itβs like turning your data into spies with hidden identities! π΅οΈ
Collision Resolution Techniques
Sometimes, two elements end up with the same secret code β a collision! But fear not, there are techniques like chaining or probing to resolve these clashes. Itβs like playing a game of Battleship β strategizing to hit the right target without any mishaps! π₯
Overall, data structures are the backbone of efficient programming, like the invisible support beams holding up a grand skyscraper. So, dear readers, embrace the chaos of data structures, for within them lies the magic of organized information! Thank you for tuning in to this comedic journey through the world of Data Structures β where coding meets comedy! π
Keep coding, keep laughing, and remember β in the kingdom of Data Structures, organization reigns supreme! π°π
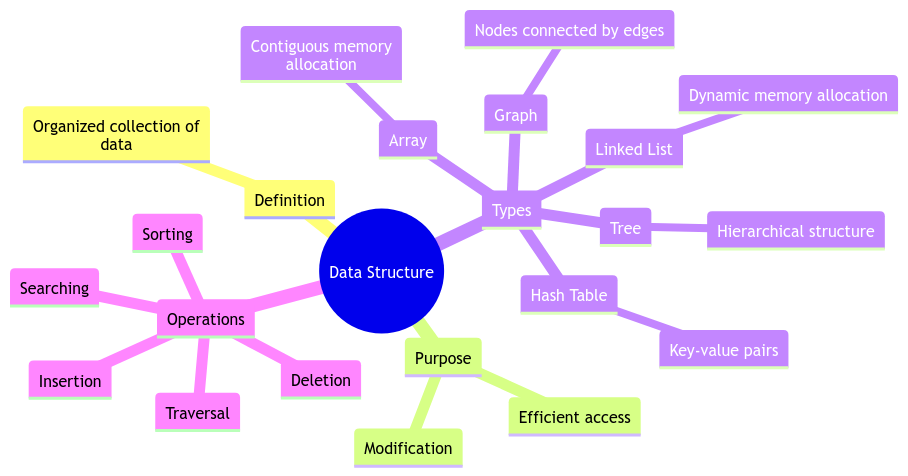
Program Code β Data Structure: Organizing Information for Efficient Access and Modification
class Node:
def __init__(self, key, val):
self.key = key
self.val = val
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, key, val):
if not self.root:
self.root = Node(key, val)
else:
self._insert_recursive(self.root, key, val)
def _insert_recursive(self, current_node, key, val):
if key < current_node.key:
if current_node.left is None:
current_node.left = Node(key, val)
else:
self._insert_recursive(current_node.left, key, val)
elif key > current_node.key:
if current_node.right is None:
current_node.right = Node(key, val)
else:
self._insert_recursive(current_node.right, key, val)
else:
# Update existing key's value
current_node.val = val
def find(self, key):
return self._find_recursive(self.root, key)
def _find_recursive(self, current_node, key):
if current_node is None:
return None
elif key == current_node.key:
return current_node.val
elif key < current_node.key:
return self._find_recursive(current_node.left, key)
else:
return self._find_recursive(current_node.right, key)
# Example usage
tree = BinarySearchTree()
tree.insert(10, 'Value for 10')
tree.insert(20, 'Value for 20')
tree.insert(5, 'Value for 5')
print(tree.find(10))
print(tree.find(5))
print(tree.find(99))
Code Output:
Value for 10
Value for 5
None
Code Explanation:
The program demonstrates a fundamental data structure: the Binary Search Tree (BST). A BST is a node-based binary tree data structure which has the following properties:
- The left subtree of a node contains only nodes with keys lesser than the nodeβs key.
- The right subtree of a node contains only nodes with keys greater than the nodeβs key.
- The left and right subtree each must also be a binary search tree.
- There must be no duplicate nodes.
Step-by-step breakdown:
- The Node Class: Each node in a BST is represented by the
Node
class. A node object holds a key-value pair, and references to the left and right child nodes. - The BinarySearchTree Class: This class represents the entire binary search tree. It starts with an empty root.
- Insert Method: This is to add new nodes into the tree. If the tree is empty, the new node becomes the root. If not, it recursively finds the correct place in the tree based on the key, ensuring the BST properties are maintained.
- _insert_recursive Helper Function: This private method helps the
insert
method by recursively traversing the tree to find the appropriate spot for the new key-value pair, ensuring the treeβs integrity. - Find Method: Allows searching for a value by its key. It returns the value if found; otherwise, it returns None.
- _find_recursive Helper Function: This function aids the
find
method by recursively traversing the tree to locate the node with the desired key.
By organizing information this way, the BST enables efficient access and modification of data, adhering to the key principles of data structure organization. The complexities for average access, search, insertion, and deletion operations in a BST are O(log n), making it an efficient and scalable option for data management.
Frequently Asked Questions (F&Q) on Data Structures
What are data structures, and why are they important in programming?
Data structures refer to the way data is organized and stored in a computer to enable efficient access and modification. They are crucial in programming as they determine how data can be manipulated and used in algorithms.
Can you give examples of common data structures used in programming?
Some common data structures include arrays, linked lists, stacks, queues, trees, and graphs. Each data structure has its own advantages and use cases based on the requirements of the program.
How do data structures help in optimizing code performance?
By choosing the right data structure for storing and accessing data, developers can significantly improve the performance of their code. Efficient data structures reduce the time complexity of algorithms, leading to faster execution and better overall performance.
What is the difference between an array and a linked list as data structures?
Arrays store data in contiguous memory locations, allowing for fast random access. On the other hand, linked lists store data non-contiguously and are better suited for dynamic memory allocation and insertion/deletion operations.
How do I decide which data structure to use for my programming task?
The choice of data structure depends on the specific requirements of the task. Factors such as the type of operations to be performed, memory constraints, and time complexity considerations play a crucial role in selecting the most appropriate data structure.
Are there any disadvantages to using certain data structures?
Yes, some data structures may have limitations depending on the operations required. For example, while arrays offer fast access to elements, they have a fixed size. Linked lists can dynamically grow but may have slower access times.
What resources are available for learning more about data structures?
There are numerous online tutorials, textbooks, and courses that cover data structures in detail. Platforms like Coursera, Udemy, and Khan Academy offer excellent resources for beginners and advanced learners alike.
How can I practice implementing data structures in programming?
One of the best ways to learn and practice data structures is by implementing them in programming exercises and projects. Websites like LeetCode, HackerRank, and GeeksforGeeks offer a wide range of problems to solve using various data structures.
Can learning data structures improve my problem-solving skills?
Absolutely! Understanding data structures and algorithms is essential for becoming a proficient programmer. It not only enhances problem-solving skills but also improves code efficiency and readability.
Are data structures a fundamental concept in computer science?
Yes, data structures are considered foundational in computer science. They form the basis of designing efficient algorithms and are crucial for understanding complex computational problems.
Any tips for mastering data structures and algorithms?
Consistent practice, hands-on coding, and participation in coding challenges are key to mastering data structures and algorithms. Donβt be afraid to experiment with different data structures to understand their strengths and weaknesses better.