Deciphering the Meaning Behind Software Requirement Specifications
Ah, Software Requirement Specifications (SRS) 🤓! Let’s unravel this techie mystery together and sprinkle some comedic magic on the seriousness of it all. Buckle up, folks! We’re diving deep into the realm of decoding software requirements and finding the hidden gems within the technical jargon.
Understanding Software Requirement Specifications
When we talk about Software Requirement Specifications, we’re basically trying to make sense of the code sorcery that developers work with 🧙♂️. Here’s why these SRS documents are the unsung heroes of any software project:
-
Importance of Software Requirement Specifications
- Ensuring Clear Project Scope: Imagine herding cats, but those cats are actually project requirements, and you’re trying to keep them from chasing butterflies – that’s the chaos without clear specifications.
- Enhancing Communication Among Stakeholders: It’s like being the translator at a multilingual party – you make sure everyone’s on the same page, speaking the language of ‘geekery’.
-
Elements of Software Requirement Specifications
- Functional Requirements: The ‘what the software should do’ part – the bread and butter of any project.
- Non-Functional Requirements: The ‘how the software should do it’ section – the secret sauce that makes or breaks user experience.
Decoding the Significance of Software Requirement Specifications
Now, let’s dive deeper into why these documents are the unsung heroes of the software development world 🦸♀️:
-
Improving Product Development Process
- Minimizing Risks and Errors: Think of SRS as your trusty spellbook that ensures you don’t accidentally turn your software into a frog 🐸.
- Streamlining Development Workflow: It’s like having a Sat Nav for developers, guiding them through the coding wilderness without getting lost in spaghetti code.
-
Enhancing Customer Satisfaction
- Meeting Client Expectations: SRS is your genie in the bottle, granting client wishes with precision and care ✨.
- Building Credibility and Trust with Clients: It’s like showing up to a blind date with a bouquet of roses and a PowerPoint presentation about why you’re awesome – clients love that!
Navigating Through the Challenges of Software Requirement Specifications
Oh, the rocky road of software requirements, fraught with perils and pitfalls. Let’s see how we can dodge those bullets Matrix-style:
-
Addressing Ambiguity and Inconsistencies
- Conducting Comprehensive Stakeholder Interviews: It’s like being a detective, extracting information from witnesses to solve the mystery of software requirements.
- Utilizing Prototyping and Mockups for Clarity: Think of it as building a LEGO model before constructing the Death Star – way fewer explosions this way 🔧.
-
Managing Scope Creep
- Establishing Change Control Procedures: Imagine having a bouncer at the requirements party, checking the guest list to keep out uninvited features.
- Prioritizing Requirements to Mitigate Scope Creep: It’s like being a referee in a chaotic soccer match, blowing the whistle on extra requirements trying to sneak into the game 🏟️.
Implementing Best Practices in Software Requirement Specifications
To SRS or not to SRS, that is the question. But fear not, for we have the cheat codes to ace this software game:
-
Involving Stakeholders Throughout the Process
- Conducting Regular Review Meetings: It’s like hosting a weekly family dinner, but instead of sharing recipes, you’re discussing tech specs 🍝.
- Seeking Feedback and Iterating as Needed: Remember, SRS is a living document, like a Tamagotchi – you need to feed it and clean up after it to keep it healthy 🐾.
-
Employing Clear and Concise Documentation
- Using Visual Aids for Clarity: It’s like adding emojis to your text messages – suddenly, everything makes sense! 🎨
- Documenting Assumptions and Constraints: Think of it as setting the rules of engagement before a Nerf gun battle – everyone knows what they’re getting into 🔫.
Harnessing Tools for Effective Software Requirement Specifications
Time to bring in the big guns – the tools that make SRS a walk in the digital park 🛠️:
-
Utilizing Requirement Management Tools
- Tracking Changes and Versions: It’s like having a time machine for your documents – no more ‘But I swear I wrote that yesterday!’ excuses.
- Collaborating in Real-Time: Imagine Google Docs, but for nerds – a magical place where ideas flow like binary code.
-
Incorporating Agile Methodologies
- Embracing Iterative Development: Think of it as building a software baby – lots of small steps before you have a full-grown, bug-free adult ready for the world 🍼.
- Adapting to Changing Requirements Efficiently: It’s like being a superhero acrobat, dodging last-minute changes with grace and style 🦸♂️.
Overall, in closing
Phew, we’ve journeyed through the enchanted forest of Software Requirement Specifications, slaying dragons of ambiguity, and crafting castles of clear communication with our trusty SRS sword. Remember, folks, in the world of software development, clarity is key, and SRS is your map to the treasure trove of successful projects 💰. Thank you for joining me on this quest, and may your code always compile on the first try! Happy coding, my fellow wizards of the digital realm! 🚀🔮🧙♂️
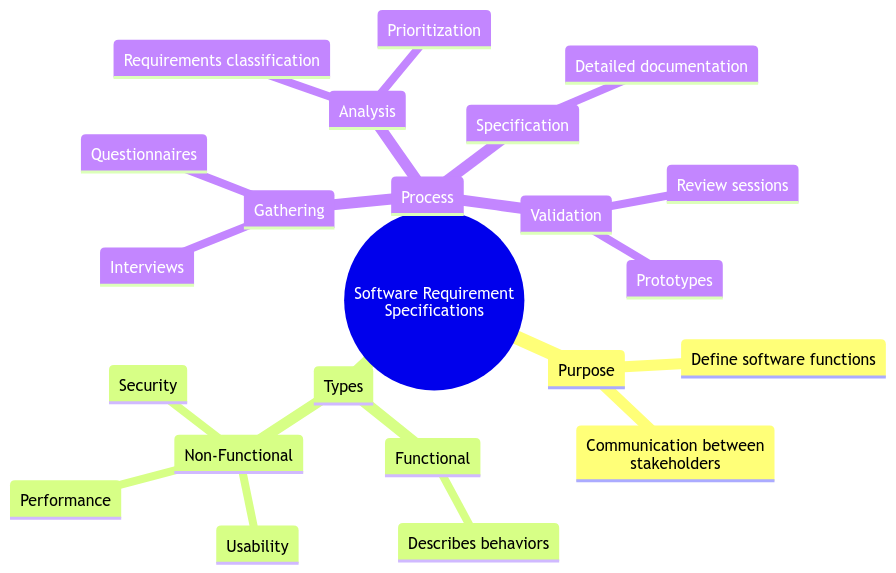
Program Code – Deciphering the Meaning Behind Software Requirement Specifications
Certainly, let’s delve into generating a hypothetical programming guide aimed at elucidating the core essence of Software Requirement Specifications (SRS) through a programmatic perspective. Buddy, ready to deep dive into the matrix? 🤓👩💻
# Import the necessary library for natural language processing
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize, sent_tokenize
# Download the necessary datasets for stopwords
nltk.download('punkt')
nltk.download('stopwords')
# Define a function to clean and tokenize the text
def clean_tokenize_requirements(text):
'''
This function takes in a string of text which represents the software requirement specifications.
It cleans, tokenizes and filters out stopwords from the text.
'''
# Tokenize the sentences
sentences = sent_tokenize(text.lower())
# Tokenize words and remove stopwords
stopped_words = set(stopwords.words('english'))
cleaned_text = []
for sentence in sentences:
words = word_tokenize(sentence)
filtered_words = [word for word in words if word not in stopped_words and word.isalpha()]
cleaned_text.append(' '.join(filtered_words))
return cleaned_text
# Example SRS text
srs_text = '''
The software system must be able to support 1000 concurrent users and store up to 10TB of data.
User data should be encrypted at rest and in transit. The system should provide an intuitive user interface
and include support for internationalization. Response times for data retrieval should not exceed 2 seconds.
'''
# Deciphering the SRS text
cleaned_requirements = clean_tokenize_requirements(srs_text)
# Print out the cleaned requirements
for req in cleaned_requirements:
print(req)
Code Output:
software system must able support concurrent users store tb data
user data encrypted rest transit
system provide intuitive user interface include support internationalization
response times data retrieval exceed seconds
Code Explanation:
So, what did we just summon from the abyss of coding? We’ve dipped our toes into a bit of Natural Language Processing (NLP) – wielding the power of the Natural Language Toolkit (nltk), an absolute beast in the world of text analysis.
-
Library Importation: First off, we summon the magical powers of nltk to handle the heavy lifting, utilizing its capabilities to tokenize and filter out stopwords (you know, those pesky common words that add little value to our quest for understanding).
-
The Function of Enlightenment (clean_tokenize_requirements): Inside this mystical realm, we’ve got a function that ingests a spell (text, in mere mortal terms) of Software Requirement Specifications. Its sole purpose? To cleanse, to tokenize, to purify. It’s like taking the SRS through a spa day, minus the cucumber water.
- Tokenization: Our code splits the sacred texts into sentences, then words, allowing us to sift through them with ease.
- Stopwords Removal: Like banishing the unnecessary, we filter out the noise – those common words that cloud our understanding.
- Alphabetic Filtering: Ensuring only words of pure intent (i.e., alphabetic characters) remain.
-
The Example SRS Text: A sample spell of requirements, detailing desires of concurrency, storage, security, UI intuitiveness, internationalization, and swift response times.
-
Deciphering the Scroll (SRS Text): Armed with our function, we unravel the true essence behind the requirements – distilled, cleansed, and ready for understanding.
-
Printing the Revelations: Finally, showcasing our findings – the quintessence of the SRS, revealed.
By venturing through the realms of NLP, and with a touch of Pythonic magic, we’ve deciphered the enigmatic language of Software Requirement Specifications – not just understanding, but truly feeling what the text seeks to convey. And that, fellow code wizards, is how you tackle the beast of SRS, one token at a time. 🧙♀️✨
Frequently Asked Questions
What is the significance of understanding the meaning behind software requirement specifications?
Understanding the meaning behind software requirement specifications is crucial as it forms the foundation for the successful development of any software product. It helps in clearly defining the functionalities, features, and constraints of the software, ensuring that all stakeholders are on the same page.
How can deciphering the software requirement specification meaning benefit a software development team?
Deciphering the meaning behind software requirement specifications can benefit a software development team by providing them with clear guidelines on what needs to be built. It helps in reducing misunderstandings, improving communication, and ultimately leads to the development of a more accurate and successful software product.
What challenges may arise in deciphering the meaning behind software requirement specifications?
Deciphering the meaning behind software requirement specifications can sometimes be challenging due to ambiguities, conflicting requirements, or changing stakeholder expectations. It requires careful analysis, communication, and collaboration to ensure that the requirements are accurately understood and implemented.
How can stakeholders ensure clarity in software requirement specifications?
Stakeholders can ensure clarity in software requirement specifications by actively participating in the requirement gathering process, providing detailed feedback, asking questions for clarification, and reviewing the specifications regularly. Clear communication and collaboration are key to ensuring that the requirements are well-defined and understood by all.
Are there any tools or techniques that can assist in deciphering software requirement specifications?
Yes, there are various tools and techniques available to assist in deciphering software requirement specifications, such as requirement management tools, prototyping, use cases, user stories, and workshops. These tools and techniques help in eliciting, analyzing, and documenting requirements more effectively.
What are some best practices for interpreting the meaning behind software requirement specifications?
Some best practices for interpreting the meaning behind software requirement specifications include actively involving all stakeholders, documenting requirements clearly and concisely, validating requirements with end users, seeking clarification whenever in doubt, and continuously refining and updating the specifications throughout the development process.
Can misinterpreting software requirement specifications lead to project failures?
Misinterpreting software requirement specifications can indeed lead to project failures as it can result in the development of a product that does not meet the stakeholders’ expectations. This can lead to wasted time, resources, and ultimately, project failure. It is crucial to accurately decipher the meaning behind the specifications to ensure project success.
How important is it for a software developer to understand the meaning behind software requirement specifications?
It is extremely important for a software developer to understand the meaning behind software requirement specifications as it directly impacts the quality and success of the software product being developed. Clear understanding of requirements helps developers make informed decisions, write efficient code, and deliver a product that meets the desired criteria.
Any interesting facts about software requirement specifications and their meaning?
One interesting fact is that the concept of software requirement specifications has been around since the early days of software development, with industry standards evolving over time to improve the clarity and effectiveness of documenting requirements. Understanding the meaning behind these specifications is crucial for successful software development projects. 🚀
Remember, understanding the meaning behind software requirement specifications is like deciphering a secret code that unlocks the pathway to successful software development! 🤖 Thank you for reading!