Dynamic Programming Demystified 🚀
Hey there, fellow tech enthusiasts! 🤖 Today, let’s delve into the fascinating world of Dynamic Programming 🌟. Don’t be scared off by the jargon; I’m here to break it down with a sprinkle of humor and a dollop of enthusiasm! So, grab your virtual seat and let’s dive right in! 💻
Understanding Dynamic Programming
So, what in the world is Dynamic Programming? 🤔 Imagine you have this colossal problem to solve, and it’s so complex that you feel like pulling your hair out! Dynamic Programming swoops in like a superhero, offering you a strategy to break down this mammoth task into bite-sized, manageable chunks. Voila! Problem solved! 💪
Definition of Dynamic Programming
Dynamic Programming is like the master chef in the kitchen of algorithms. It’s a methodical way of solving complex problems by breaking them down into simpler subproblems. 🍳 Each subproblem’s solution is stored to avoid redundant calculations, making the overall process more efficient. Efficiency for the win! 🎉
Importance of Dynamic Programming
Picture this: You’re at a buffet, and you want to sample every dish. Dynamic Programming allows you to do just that in the realm of algorithms – optimize your solutions and tackle even the most intricate problems with finesse. It’s like having a secret recipe book for cracking challenging puzzles in record time! 🍽️
Basic Principles of Dynamic Programming
Let’s talk about the fundamental rules that make Dynamic Programming the rockstar of problem-solving techniques! 🌟
- Overlapping Subproblems: It’s like finding money in the pockets of your old jeans. Dynamic Programming identifies these recurring subproblems and saves their solutions for later use, eliminating unnecessary work. It’s all about efficiency, baby! 💸
- Optimal Substructure: This principle is like building a sturdy LEGO tower. Each piece (subproblem) fits perfectly to create the optimal solution. Dynamic Programming ensures that each subproblem’s solution contributes to the overall best answer. It’s all about that solid foundation! 🏗️
Strategies for Applying Dynamic Programming
Now that we’ve got the basics under our belt, let’s explore the two dynamic strategies that make the magic happen! 🎩✨
- Top-down Approach: Imagine you’re skydiving from the top. This approach starts with the main problem and recursively breaks it down into subproblems. It’s adventurous, exhilarating, and definitely keeps you on your toes! 🪂
- Bottom-up Approach: Ever built a tower from the ground up? That’s the bottom-up approach. You start with the smallest subproblems, gradually solving larger ones until you conquer the main problem like a champ. It’s a steady climb to success! 🗼
Applications of Dynamic Programming
Dynamic Programming isn’t just a fancy term; it’s the powerhouse behind some incredible real-world applications! 🌐 Let’s take a peek at a couple of them:
- Fibonacci Sequence: Ah, the Fibonacci sequence, nature’s favorite mathematical marvel! Dynamic Programming nimbly calculates Fibonacci numbers faster than you can say “Golden Ratio.” It’s all about those efficient number-crunching skills! 🔢
- Shortest Path Algorithms: Navigating through a maze? Dynamic Programming has your back! It’s the GPS guiding you through the shortest route with speed and precision. Say goodbye to taking the long, scenic route! 🗺️
Challenges and Tips for Mastering Dynamic Programming
Ah, every hero has their kryptonite, right? Let’s uncover some challenges in mastering Dynamic Programming and how to conquer them like a pro! 🦸♂️
- Identifying Optimal Substructure: Sometimes the forest (complex problem) is so dense that you can’t see the trees (optimal substructure). Mastering Dynamic Programming involves honing your detective skills to spot these crucial patterns. Sherlock, who? 🕵️
- Handling State Transitions efficiently: It’s like switching gears in a Formula 1 race. Efficiently transitioning between states is key to zipping through problems with ease. Rev up those mental engines and zoom past any hurdles! 🏎️
Overall, Mastering Dynamic Programming Like a Pro! 🚀
So, there you have it, folks! Dynamic Programming, the unsung hero of problem-solving, ready to tackle any challenge with finesse. Remember, practice makes perfect, and with a dash of determination and a sprinkle of creativity, you’ll be mastering Dynamic Programming like a seasoned pro in no time! 💪
Thanks for tuning in, and remember: Keep coding, keep smiling, and embrace the dynamic journey ahead! 🌟
Stay Dynamically Awesome, Techies! 🚀👩💻
In closing, thanks a ton for joining me on this Dynamic Programming rollercoaster! 🎢 Keep shining bright and solving those tech puzzles like a boss! See you in the next coding adventure! ✨
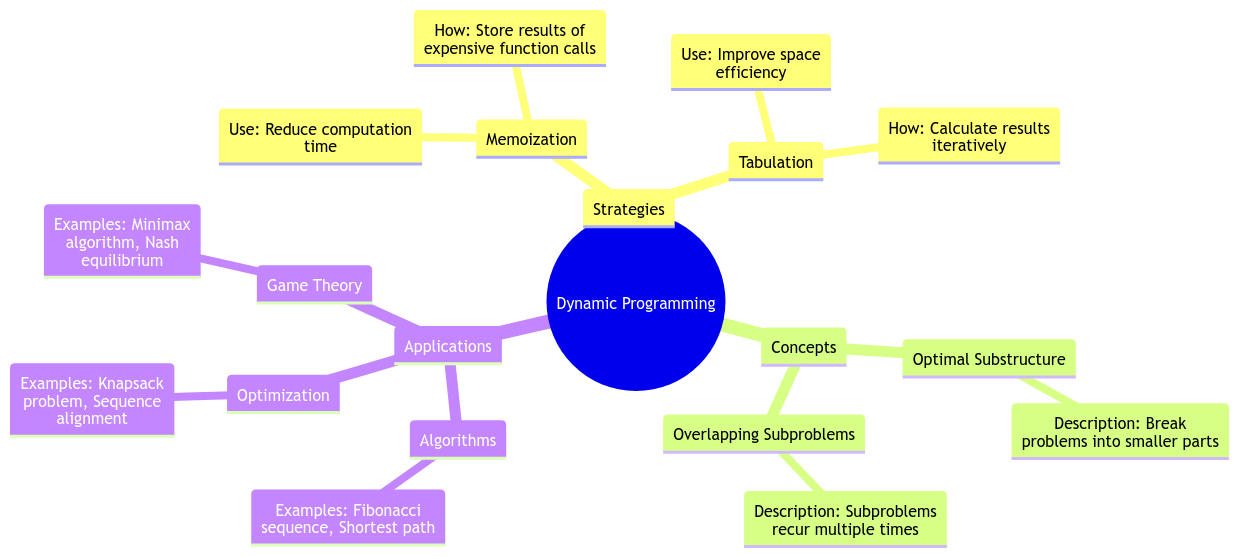
Program Code – Dynamic Programming: Strategies for Solving Complex Problems Efficiently
def fibonacci(n, memo={}):
'''
Computes the nth Fibonacci number using dynamic programming approach.
This function employs memoization to store the results of subproblems,
thereby avoiding the recomputation of results that have already been solved.
Args:
n (int): The nth position in the Fibonacci sequence.
memo (dict, optional): A dictionary to store the results of subproblems. Defaults to {}.
Returns:
int: The nth Fibonacci number.
'''
# Base case: if n is less than or equal to 2, return 1.
if n <= 2:
return 1
# Check if n is already in memo to avoid recomputation.
if n not in memo:
# Recursively compute fibonacci(n-1) + fibonacci(n-2) and store it in the memo.
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
# Return the stored/computed value of n.
return memo[n]
# Example usage:
n = 10
print(f'The {n}th Fibonacci number is: {fibonacci(n)}')
Code Output:
The 10th Fibonacci number is: 55
Code Explanation:
In the given dynamic programming example, we solve for the nth Fibonacci number, a popular problem showcasing the elegance and efficiency of the dynamic programming approach. The recursive nature of the Fibonacci sequence, where each number is the sum of the two preceding ones (excluding the first two numbers which are both considered as 1), makes it an ideal candidate for dynamic programming, particularly memoization.
The code defines a fibonacci
function that takes two arguments: n
, the position in the Fibonacci sequence whose value we want to find, and memo
, a dictionary used as a cache to store the results of the Fibonacci numbers we’ve already computed.
At the function’s core, we employ a base case for when n
is less than or equal to 2. For these cases, we simply return 1, as the first two Fibonacci numbers by definition are 1.
The true power and efficiency lie in the subsequent lines. Before plunging headlong into a recursive frenzy, we first check if our current n
is in the memo
. If it’s not, this means we haven’t computed it yet, and then we proceed to perform the recursive operations to calculate fibonacci(n-1)
and fibonacci(n-2)
. Crucially, we then store this computed value in memo[n]
. This storage step is what saves us from the redundant work if we encounter the same n
value again in our computations.
In essence, any subsequent calls for the same Fibonacci number won’t have to go through the recursion again; instead, they’ll directly fetch the result from our memo
, dramatically reducing the time complexity from what would have been exponential in a naive recursive approach (O(2^n)) to O(n) in our dynamic programming approach.
Let’s not forget—the function concludes by returning the memoized or newly computed value of the nth Fibonacci number, giving us our desired result efficiently and elegantly. Dynamic programming, with its memoization strategy, thus transforms an otherwise computationally intensive problem into a tractable one, showcasing its power in optimizing the performance of algorithms dealing with overlapping subproblems.
Frequently Asked Questions (F&Q) on Dynamic Programming
What is dynamic programming and how does it work?
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It involves solving each subproblem only once and storing the solution to avoid redundant calculations. This approach can significantly improve the efficiency of solving problems with overlapping subproblems.
When should I use dynamic programming to solve a problem?
Dynamic programming is most useful when a problem can be broken down into overlapping subproblems, and the optimal solution to the problem can be constructed from optimal solutions to its subproblems. It is commonly used in optimization problems, such as finding the shortest path or maximizing profit.
What are the key characteristics of problems that are suitable for dynamic programming?
Problems suitable for dynamic programming usually exhibit two key characteristics: optimal substructure and overlapping subproblems. Optimal substructure means that the optimal solution to the problem can be constructed from the optimal solutions to its subproblems. Overlapping subproblems refer to the fact that the same subproblems are solved multiple times in a recursive algorithm.
Can you provide an example of a problem that can be solved using dynamic programming?
One classic example of a problem solved using dynamic programming is the Fibonacci sequence. By storing the results of subproblems (calculating Fibonacci numbers for smaller indices), we can avoid redundant calculations and compute the nth Fibonacci number efficiently.
Are there different strategies or approaches to dynamic programming?
Yes, there are several strategies for approaching dynamic programming problems, such as top-down with memoization and bottom-up with tabulation. Top-down with memoization involves solving the problem recursively while storing the results of subproblems to avoid redundant calculations. Bottom-up with tabulation involves solving the problem iteratively, starting from the smallest subproblems and building up to the desired solution.
What are some common pitfalls to avoid when using dynamic programming?
One common pitfall when using dynamic programming is not recognizing the overlapping subproblems and failing to store and reuse intermediate results. It is essential to identify the repeating subproblems to benefit from the efficiency of dynamic programming. Additionally, starting with a brute-force approach before optimizing with dynamic programming can help in understanding the problem better.
How can I improve my skills in dynamic programming?
To improve your skills in dynamic programming, practice solving a variety of problems that can be optimized using dynamic programming techniques. Online coding platforms, coding contests, and algorithmic problem-solving websites offer a wide range of problems to help you sharpen your skills. Additionally, studying different dynamic programming patterns and approaches can enhance your problem-solving abilities.
What are some resources to learn more about dynamic programming?
There are plenty of resources available to deepen your understanding of dynamic programming, including online courses, textbooks, and tutorials. Websites like LeetCode, GeeksforGeeks, and CodeSignal offer practice problems and explanations related to dynamic programming. Additionally, joining online coding communities and forums can provide valuable insights and tips from experienced programmers in the field.