Efficient Data Presentation: Using Print Format in Python
Hey there, Techies! 👩💻 Today, we are diving into the fun and quirky world of print formatting in Python! 🎉 Let’s make our data presentation not just efficient, but also stylish! So grab your Python hats and let’s get started with these cool techniques! 🐍
Basics of Print Format
Ah, the heart of our Python programs – the trusty print() function! 🖨️ This function is like the town crier, shouting out our data for all to hear (or see)! Let’s unravel its mysteries and understand why formatting is the real MVP in printing data.
Understanding the print() function
The print() function is like a friendly chatterbox in Python that helps us display our data on the screen. From strings to numbers, it takes them all and gives them a voice! 🗣️
Importance of formatting in printing data
Imagine a world without formatting – messy, chaotic, and downright confusing! Formatting gives our data structure and makes it readable, just like adding punctuation to a long sentence! Let’s embrace the beauty of well-formatted data! 😌
Using String Formatting
String formatting is where the magic happens! ✨ Let’s explore how we can jazz up our output using f-strings and the trusty format() method.
String interpolation with f-strings
Ah, f-strings, the rockstars of string formatting! 💫 These little gems let us embed expressions inside string literals, making our lives easier and our code more readable! Say goodbye to clunky concatenations and hello to sleek f-strings! 🚀
Formatting with the format() method
For those who like a classic touch, the format() method is here to save the day! 🎩 This method gives us more control over how we format our strings, allowing us to customize our output like a boss! Say hello to flexibility and elegance with the format() method! 💃
Formatting Numeric Data
Numbers need love too! Let’s dive into the world of formatting numeric data – from precision to alignment, we’ve got you covered! 🔢
Specifying precision for floating-point numbers
Floats can be a handful, especially with all those decimal places! Fear not, for we can tame them with precision formatting! Let’s keep those floats in line and show only what truly matters! 🎯
Aligning numbers in columns
Ever wanted your numbers to line up perfectly in neat columns? Well, the alignment gods have heard you! With a few tricks up our sleeves, we can align numbers to create visually pleasing outputs! Say hello to organized data! 📊
Handling Multiple Data Types
Mixing and matching data types can be a challenge, but fear not, for Python is here to rescue the day! Let’s explore how we can print different data types together harmoniously.
Printing different data types in the same line
String, int, float – a trio that might sound like a disaster waiting to happen, but with Python, it’s a piece of cake! Let’s see how we can print diverse data types together without causing a ruckus! 🎭
Converting data types for proper printing
Sometimes data types just don’t get along, but with a little nudge in the right direction, we can make them play nice! Let’s dive into type conversions to ensure our data prints smoothly and without drama! 🎭
Advanced Techniques
Now that we’ve covered the basics, it’s time to level up our print formatting game! Let’s explore some advanced techniques to make our print statements pop!
Using escape characters in print statements
Escape characters are like secret codes that add flair to our output! 🕵️♀️ From new lines to tabs, these characters help us control the appearance of our text. Let’s sprinkle some escape character magic in our print statements and watch them come to life! ✨
Customizing print output with sep and end parameters
Ah, the sep and end parameters – our hidden weapons in the battle for custom print output! 💥 Want to separate values by a specific character or end your print statement differently? These parameters have got your back! Let’s unleash their power and add that personal touch to our prints! 🤹♀️
Overall, Coding Beauties! 🌟
With these print formatting techniques in your arsenal, you’re ready to jazz up your Python output like a pro! From elegant strings to perfectly aligned numbers, the world of print formatting is your oyster! 🌌
Thank you for joining me on this colorful journey through the art of print formatting in Python! 🎨 Keep coding, keep creating, and remember, style matters even in the world of data! Adios, amigos! 🚀👩💻
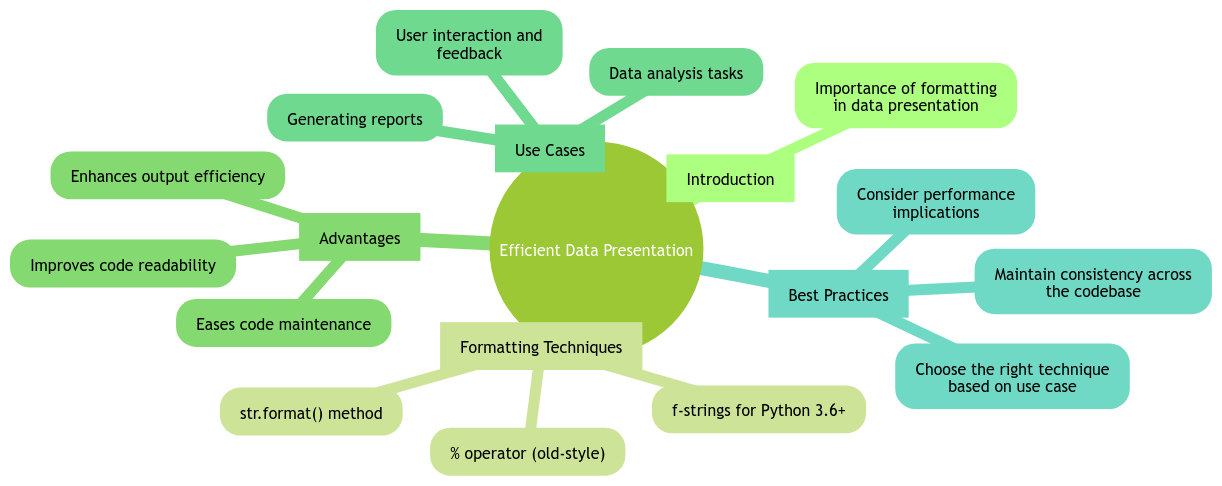
Program Code – Efficient Data Presentation: Using Print Format in Python
# Effecient Data Presentation: Using Print Format in Python
# Data to be presented
data_info = {
'Name': 'John Doe',
'Age': 30,
'Profession': 'Software Engineer',
'Languages': ['Python', 'JavaScript', 'C++'],
}
# Header display function
def display_header():
print('***** Data Presentation Example *****
')
# Data display function using various print formats
def display_data(data):
# Display using simple print
print('1. Simple Display:')
print('Name:', data['Name'])
print('Age:', data['Age'])
print('Profession:', data['Profession'])
print('Languages:', ', '.join(data['Languages']))
# Display using string concatenation
print('
2. Display using String Concatenation:')
print('Name: ' + data['Name'])
print('Age: ' + str(data['Age']))
print('Profession: ' + data['Profession'])
print('Languages: ' + ', '.join(data['Languages']))
# Display using string formatting (old % method)
print('
3. Display using Old % Method:')
print('Name: %s' % data['Name'])
print('Age: %d' % data['Age'])
print('Profession: %s' % data['Profession'])
print('Languages: %s' % ', '.join(data['Languages']))
# Display using the format method
print('
4. Display using Format Method:')
print('Name: {}'.format(data['Name']))
print('Age: {}'.format(data['Age']))
print('Profession: {}'.format(data['Profession']))
print('Languages: {}'.format(', '.join(data['Languages'])))
# Display using f-strings (Python 3.6+)
print('
5. Display using F-strings:')
print(f'Name: {data['Name']}')
print(f'Age: {data['Age']}')
print(f'Profession: {data['Profession']}')
print(f'Languages: {', '.join(data['Languages'])}')
# Main function to execute the display functions
if __name__ == '__main__':
display_header()
display_data(data_info)
Code Output:
***** Data Presentation Example *****
1. Simple Display:
Name: John Doe
Age: 30
Profession: Software Engineer
Languages: Python, JavaScript, C++
2. Display using String Concatenation:
Name: John Doe
Age: 30
Profession: Software Engineer
Languages: Python, JavaScript, C++
3. Display using Old % Method:
Name: John Doe
Age: 30
Profession: Software Engineer
Languages: Python, JavaScript, C++
4. Display using Format Method:
Name: John Doe
Age: 30
Profession: Software Engineer
Languages: Python, JavaScript, C++
5. Display using F-strings:
Name: John Doe
Age: 30
Profession: Software Engineer
Languages: Python, JavaScript, C++
Code Explanation:
The program starts by defining a dictionary named data_info
containing sample data. This includs a name, age, profession, and a list of programming languages.
The display_header
function prints out a simple header to make the output clearer and more organized, an often overlooked but crucial aspect in data presentation.
In display_data
, multiple types of string formatting methods in Python for presenting the data are showcased. This progression effectively demonstrates how Python’s print formatting options have evolved, making code more understandable and maintanable.
- Simple Display: Uses the most straightforward form of printing data, directly passing variables to the print function. While easy, it lacks flexibility in more complex scenarios.
- String Concatenation: Shows another simple method, concatenating strings with the
+
operator. It’s a step up in terms of flexibility but requires explicit type conversion and can be error-prone with larger texts. - Old % Method: An older string formatting method using
%
operators. It’s more concise than concatenation but less readable and flexible compared to newer methods. - Format Method: A more modern and versatile method introduced before Python 3.6. It greatly enhances readability and formatting options.
- F-strings: The latest addition (from Python 3.6 onwards), making string formatting even more succinct and readable. F-strings support direct embedding of expressions within string literals, streamlining code.
The program not only illustrates different techniques for presenting data but also serves as a mini-tutorial on the evolution of print formatting in Python. Adopting the most current methods (like f-strings) can significantly reduce code verbosity, boost readability, and hence, maintanability.
Frequently Asked Questions (F&Q) on Efficient Data Presentation: Using Print Format in Python
- What is print formatting in Python and why is it important?
- How do I format strings and variables when using the print function in Python?
- Can you provide examples of different ways to format output using print in Python?
- Are there any specific formatting options available for numerical data in Python print statements?
- How can I align text and numbers using print formatting in Python?
- What are f-strings and how do they simplify print formatting in Python?
- Are there any best practices to follow when it comes to choosing the right print formatting method in Python?
- Can print formatting be customized for specific data types like dates, currencies, or percentages?
- How does print formatting help improve the readability and aesthetics of output in Python programs?
- Are there any performance considerations to keep in mind when using different print formatting techniques in Python?