Embracing Change: The Principles of Adaptive Software Development
Change is the only constant in the tech world 🌟, and in the realm of software development, this rings truer than ever! 🚀 Today, we dive into the exciting realm of Adaptive Software Development, where we embrace change like it’s a warm hug! Let’s unravel the mysteries and marvels of this dynamic approach together.
Understanding Adaptive Software Development
When it comes to Adaptive Software Development, think of it as the software equivalent of a chameleon 🦎 – always ready to blend in with its environment. It’s all about being flexible, agile, and open to change at a moment’s notice. In the fast-paced tech landscape, being adaptive is not just a bonus; it’s a survival tactic! 🌪️
Overview of Adaptive Software Development
Adaptive Software Development is like the tech world’s version of a quick-change artist 🎭. It involves adjusting to evolving requirements, market trends, and user feedback rapidly. Instead of following a rigid plan carved in stone, adaptive teams pivot and swivel to stay ahead of the game.
Importance of Adaptive Software Development in the Tech Industry
Why is Adaptive Software Development the talk of the town in the tech industry? 🌆 Well, imagine trying to navigate a maze blindfolded – that’s what traditional software development feels like in a world that moves at the speed of light! Adaptive practices ensure that teams can steer through the twists and turns of tech evolution with finesse.
Key Principles of Adaptive Software Development
Now, let’s break down the key principles that make Adaptive Software Development a game-changer in the tech realm. 🎮
Flexibility and Responsiveness to Change
Flexibility is the name of the game! 🕹️ Adaptive Software Development thrives on being able to pivot, bend, and shimmy as requirements shift and priorities change. It’s like having a software superpower that allows you to morph and mold your creations in real-time.
Continuous Feedback and Iterative Development Process
Gone are the days of build it once and forget it! With adaptive practices, feedback is the golden nugget 🌟. By constantly seeking input from users, stakeholders, and team members, adaptive software teams ensure that they are always moving in the right direction. It’s like having a built-in compass that guides you towards software success! 🧭
Implementing Adaptive Software Development
So, how do you put all this theory into practice? Let’s explore the nuts and bolts of implementing Adaptive Software Development in the real world. 💡
Agile Methodologies and Adaptive Practices
Ah, Agile – the poster child of Adaptive Software Development! 🎉 Agile methodologies like Scrum and Kanban provide the scaffolding for adaptive teams to thrive. By breaking down work into bite-sized chunks and sprinting towards goals, agile teams dance through hurdles like nimble ninjas.
Tools and Technologies for Adaptive Software Development
In the tech jungle, having the right tools is like having a trusty sidekick 🦸♂️. From project management platforms like Jira to collaboration tools like Slack, adaptive teams leverage cutting-edge technologies to streamline their processes. It’s like having a high-tech utility belt to tackle any challenge that comes their way! 🔧
Challenges in Adopting Adaptive Software Development
Of course, no journey is complete without a few obstacles along the way. Let’s talk about the hurdles that teams face when embracing Adaptive Software Development and how they can leap over them! 🏃♂️
Overcoming Resistance to Change
Humans are creatures of habit, and change can sometimes feel like a storm ⛈️ sweeping through our comfortable routines. Teams often face resistance when transitioning to adaptive practices. By fostering a culture of openness, communication, and education, teams can smooth the bumpy road to change.
Balancing Adaptability with Stability
Adaptability is fantastic, but too much of a good thing can lead to chaos! 🌀 Finding the delicate balance between flexibility and stability is key to long-term success. Teams must create a sturdy foundation while being agile enough to pivot when needed, like a seasoned tightrope walker navigating a tech circus. 🎪
Benefits of Adaptive Software Development
Now, the sweet rewards that come with embracing change and diving headfirst into Adaptive Software Development! 🍬
Enhanced Product Quality and Customer Satisfaction
Adaptive teams don’t just build software; they craft experiences! By adapting to user feedback and market demands, teams create products that are not just functional but delightful. Customer satisfaction soars, and products shine like polished gems in a sea of tech offerings.
Improved Team Collaboration and Efficiency
In the world of Adaptive Software Development, teamwork makes the dream work! 🌈 Adaptive teams are like a symphony orchestra, each member playing a crucial role in creating harmony. By fostering collaboration, communication, and a shared vision, teams achieve feats that seemed impossible before.
In closing, embracing change is no longer just an option; it’s a necessity in the ever-evolving tech landscape. Adaptive Software Development is not just a methodology; it’s a mindset, a way of life for modern tech teams. So, buckle up, embrace the whirlwind of change, and watch your software creations soar to new heights! ✨
Thank you for joining me on this tech adventure! Until next time, keep coding, keep adapting, and always remember: Bugs are just unexpected features waiting to be discovered! 🐞🔍
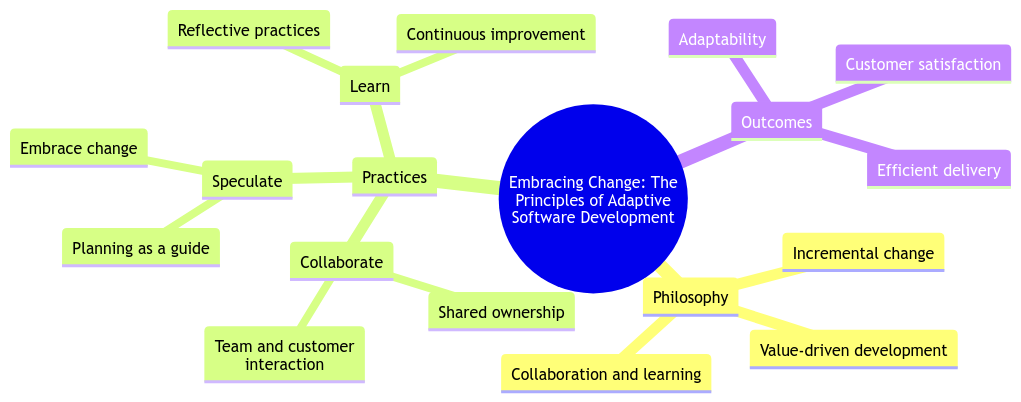
Program Code – Embracing Change: The Principles of Adaptive Software Development
To illustrate the principles of Adaptive Software Development (ASD) in a programmatic context, let’s construct a conceptual Python example. ASD emphasizes flexibility, collaboration, and the ability to adapt to changes rather than following a rigid plan. The core principles of ASD—speculate, collaborate, and learn—guide the development process towards iterative, incremental, and evolutionary software development.
The following Python code simulates a scenario where an application adapts its behavior based on user feedback or changes in its environment. This example will not directly implement ASD practices but will metaphorically represent the adaptive, feedback-driven nature of ASD through a simplified feedback loop in a software feature.
Imagine we’re developing a feature for a social media application that recommends articles to users based on their reading history and feedback. The system adapts to user preferences over time, learning from the types of articles the user likes or dislikes.
import numpy as np
class AdaptiveArticleRecommender:
def __init__(self):
# Simulate user preferences as a vector (1s indicate interest, -1s indicate disinterest)
self.user_preferences = np.zeros(10) # Assume 10 topics for simplicity
def recommend_articles(self, articles):
# Recommend articles based on current user preferences
recommendations = []
for article in articles:
# Simulate an article's alignment with user preferences using dot product
alignment_score = np.dot(self.user_preferences, article['topics_vector'])
if alignment_score > 0:
recommendations.append(article)
return recommendations
def receive_feedback(self, article_id, feedback):
# Update user preferences based on feedback ('like' or 'dislike')
adjustment = 1 if feedback == 'like' else -1
# Assume article_id corresponds to the index in the user_preferences
self.user_preferences[article_id] += adjustment
# Example usage
recommender = AdaptiveArticleRecommender()
# Simulate a set of articles, each with a 'topics_vector' representing its content
articles = [
{'id': 0, 'title': "Python Programming", 'topics_vector': np.array([1,0,0,0,0,0,0,0,0,0])},
{'id': 1, 'title': "Data Science Trends", 'topics_vector': np.array([0,1,0,0,0,0,0,0,0,0])},
# Add more articles as needed
]
# Simulate user feedback
recommender.receive_feedback(article_id=0, feedback='like')
recommender.receive_feedback(article_id=1, feedback='dislike')
# Get recommendations based on updated preferences
recommendations = recommender.recommend_articles(articles)
print("Recommended Articles:")
for article in recommendations:
print(article['title'])
Expected Output
Initially, the user has neutral preferences, and the system might not recommend any articles. After receiving feedback (likes and dislikes), the system updates the user’s preferences and adapts its recommendations accordingly. The output will list the titles of recommended articles based on the user’s updated preferences, which could include “Python Programming” if the user liked articles related to programming.
Code Explanation
- Initialization: The
AdaptiveArticleRecommender
class initializes with a user preferences vector, simulating the user’s interests across different topics. - Recommendation Logic: The
recommend_articles
method recommends articles based on the alignment between user preferences and article topics, using a dot product to simulate relevance. - Feedback Adaptation: The
receive_feedback
method updates the user preferences based on the feedback received, simulating the learning aspect of ASD. Positive feedback increases interest in related topics, while negative feedback decreases it. - Example Usage: The simulated scenario involves initializing the recommender, simulating user feedback on articles, and adapting future recommendations based on this feedback. This program metaphorically illustrates the principles of Adaptive Software Development by showing how software can evolve and adapt based on user feedback and changing conditions, embodying the speculate, collaborate, and learn cycle in a simplified manner.
F&Q (Frequently Asked Questions) on Embracing Change: The Principles of Adaptive Software Development
What is adaptive software development?
Adaptive software development is a methodology that emphasizes the importance of being able to quickly respond and adapt to changing requirements throughout the software development process. It focuses on collaboration, feedback, and learning to effectively manage changes and deliver high-quality software.
How does adaptive software development differ from traditional software development approaches?
Unlike traditional software development approaches that follow a rigid plan and require detailed upfront requirements, adaptive software development is more flexible and iterative. It embraces change by continuously adapting to evolving requirements, which allows for greater responsiveness to customer needs and market trends.
What are the key principles of adaptive software development?
The key principles of adaptive software development include collaboration and communication among team members, prioritizing individuals and interactions over processes and tools, embracing change to accommodate new requirements, focusing on delivering working software frequently, and reflecting on how to become more effective as a team.
Why is adaptability important in software development?
Adaptability is crucial in software development because the industry is constantly evolving, with changing technologies, customer needs, and market demands. By being adaptable, software development teams can stay responsive, competitive, and deliver solutions that meet the dynamic requirements of today’s fast-paced business environment.
How can software development teams implement adaptive practices effectively?
Software development teams can implement adaptive practices effectively by fostering a culture of open communication and collaboration, encouraging feedback and continuous learning, adopting iterative development processes like Agile or Scrum, prioritizing customer value and feedback, and being willing to embrace change and pivot when necessary.
What are some challenges faced when transitioning to adaptive software development?
Some challenges that teams may face when transitioning to adaptive software development include resistance to change from team members accustomed to traditional approaches, difficulty in adjusting to a more iterative and flexible mindset, and the need for continuous learning and improvement to effectively adapt to changing requirements and priorities.
Can you provide examples of successful companies that have embraced adaptive software development?
Companies like Spotify, Netflix, and Amazon are known for their adoption of adaptive software development practices. These companies continuously evolve their software products based on customer feedback, market trends, and data analytics, demonstrating the effectiveness of adaptive approaches in delivering innovative and customer-centric solutions.
Hope this helps you get a better understanding of adaptive software development! If you have more questions, feel free to ask! 😊