How to Find Out Which Class an Object (Instance) Belongs to in Phyton Programming. Python is one of the most popular and powerful languages used by programmers to code applications. Python is an object-oriented language where you can easily write codes using classes. So, in this tutorial, we will learn how to find out which class an object belongs to in python programming.
As the name suggests, you will learn about the basics of python programming, then you will learn to identify the type of objects, and finally, you will learn how to find out which class an object belongs to.
Let us start with the introduction of python
Python is a high-level programming language that allows you to write simple and readable codes. It is designed to be very powerful and to allow you to work with the machine directly without going through the OS. It supports object-oriented programming which means that you can easily define the classes and methods.
Object-oriented programming is nothing but creating a group of data and functions that will perform specific tasks. For example, let us take a class called class1 and we will create two variables x and y. Now, if you create an object of class1, you will get x and y.
So, the basic idea of object-oriented programming is that the classes can be used to define the types of objects. So, class1 will be used to define the objects of class1, class2 will be used to define the objects of class2, and so on.
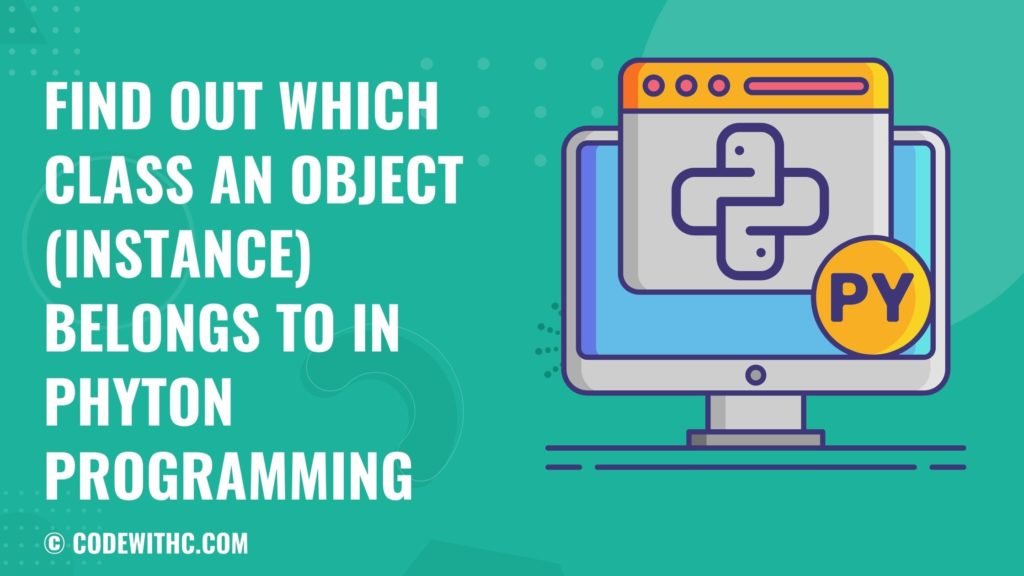
Objects in python programming
Objects are used everywhere in programming, we use objects to store data and information and it is very common in programming. An instance of an object is a single copy of the same object, which means that it has all the properties of the parent object and can do whatever it wants.
Every object has a type and there is only one instance of that type in the whole program. All objects that belong to the same type share the same properties and methods. A class is a template for creating objects of the same type. A class contains a set of properties and functions that describe the object.
We can create different classes for different types of objects and each class has a set of properties and methods that define what that particular object is. For example, we can create a car class and make different instances of this class for different cars.
So, how can we find out which class an object (instance) belongs to in phyton programming?
What is Class?
In the Python language, an object is a collection of information. Every Python object has a class that defines the structure of the object. An object of a class has attributes, methods, and instance variables.
For example, consider the following code:
import sys
class Student(object):
pass
sys.stdout = open('output.txt','w')
Student.name = 'Isha'
print(Student.name)
Here, the Student is the class and the name is the attribute of the class. Now, when we print the value of the variable name, we get the output as follows:
Isha
The variable name is an instance variable of the class student.
Now, as the name suggests, you will use python to write codes that will help you to solve the problems. The most common problem faced by the developers is finding out which class an object belongs to.
Example: 1
Let us assume that we have created two classes called Car and Motorcycle. We can create the object of either of the classes, and the instance of the class is not fixed to any one of the objects. For example, if we create an object of class Car, then it is a car object. However, if we create an object of the class Motorcycle, then it is a motorcycle object.
Here is the code for the above scenario:
class Car {
private int speed;
public Car(int speed) {
this.speed = speed;
}
public int getSpeed() {
return this.speed;
}
}
class Motorcycle {
private int speed;
public Motorcycle(int speed) {
this.speed = speed;
}
public int getSpeed() {
return this.speed;
}
}
class Driver {
public static void main(String[] args) {
Car car1 = new Car(100);
Motorcycle motorcycle1 = new Motorcycle(90);
System.out.println("Car Speed = "+car1.getSpeed());
System.out.println("Motorcycle Speed = "+motorcycle1.getSpeed());
}
}
In the above program, we have created two classes, one for cars and another for motorcycles. The constructor of the class defines the name and the speed of the object. The getSpeed method returns the speed. The driver class shows how to find out which class an object belongs to.
In the driver class, we have created two objects car1 and motorcycle1. The car object is of class Car and the motorcycle object is of class Motorcycle. If you run the driver class, you will get the following output:
Car Speed = 100
Motorcycle Speed = 90
You can also find out which class an object belongs to by using the getClass() method.
Example: 2
import random
class1 = {}
x=0
y=0
def set():
global x
global y
x=random.randint(10,20)
y=random.randint(10,20)
return x,y
class1.__init__=set
print(class1.__init__)
for i in range(100):
print(i)
Example: 3
import re
a = 2
b = "3"
c = 2.3
m = re.search(r'\d', str(c))
print(a. class ) # <type 'int'>
print(b. class ) # <type 'str'>
print(c. class ) # <type 'float'>
print(type(a)) # <type 'int'>
print(type(b)) # <type 'str'>
print(type(c)) # <type 'float'>
print(a. class . name ) # int
print(b. class . name ) # str
print(c. class . name ) # float
print(re. class . name ) # module
print(m. class . name ) # SRE_Match or Match
In this tutorial, we discussed the concepts of finding out which Class and Object (Instance) Belong in Phyton Programming. If you want to know more about Python programming then please read the tutorial on python basics.