Gaussian Filter is a 2D convolution operator which is extensively used in Image Processing to reduce the noises and details in digital images. As Gaussian Filter has the property of having no overshoot to step function, it carries a great significance in electronics and image processing.
In this post, we are going to generate a 2D Gaussian Kernel in C++ programming language, along with its algorithm, source code, and sample output.
Gaussian Filter Theory:
Gaussian Filter is based on Gaussian distribution which is non-zero everywhere and requires large convolution kernel. During image processing, the collected discrete pixels of the stored image need to be produced as discrete approximation to Gaussian Function before convolution.
The 2D Gaussian Kernel follows the Gaussian distribution as given below:
Where, x is the distance along horizontal axis measured from the origin, y is the distance along vertical axis measured from the origin and σ is the standard deviation of the distribution.
During the Gaussian Filtering process, the mean of the distribution is assumed to be at origin O(0, 0). The graphical representation of a 2D Gaussian distribution with mean at origin and standard deviation equal to 1 is as follows:
Algorithm:
- Start
- Declare the function to generate Gaussian Filter
- Declare variable as double data type
- Call the function named filter with gk i.e Gaussian kernel argument
- Display the generated Gaussian filter using loop
- Define the function with following sub steps:
- Declare variables to represent standard deviation, mean, horizontal distance, vertical distance etc.
- Assign the value of standard deviation to 1
- Initialize the sum for normalization process
- Generate a Kernel of desired order using loop (in the program below, a 5×5 kernel has been generated)
- Normalize the generated kernel
- Return generated filter to the calling function
- Stop
Gaussian Filter Generation in C++:
#include <iostream>
#include <cmath>
#include <iomanip>
using namespace std;
void filter(double gk[][5]); // Declaration of function to create Gaussian filter
int main()
{
double gk[5][5];
filter(gk); // Function call to create a filter
for(int i = 0; i < 5; ++i) // loop to display the generated 5 x 5 Gaussian filter
{
for (int j = 0; j < 5; ++j)
cout<<gk[i][j]<<"\t";
cout<<endl;
}
}
void filter(double gk[][5])
{
double stdv = 1.0;
double r, s = 2.0 * stdv * stdv; // Assigning standard deviation to 1.0
double sum = 0.0; // Initialization of sun for normalization
for (int x = -2; x <= 2; x++) // Loop to generate 5x5 kernel
{
for(int y = -2; y <= 2; y++)
{
r = sqrt(x*x + y*y);
gk[x + 2][y + 2] = (exp(-(r*r)/s))/(M_PI * s);
sum += gk[x + 2][y + 2];
}
}
for(int i = 0; i < 5; ++i) // Loop to normalize the kernel
for(int j = 0; j < 5; ++j)
gk[i][j] /= sum;
}
The given program doesn’t need any value as input; all the data are already defined within the source code. When this C++ program for Gaussian Filter Generation is executed, it displays a 5×5 kernel. A sample output screenshot is shown below:
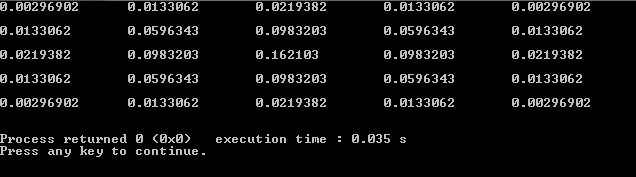
The given source code is to be compiled in Code::Blocks. It is well tested and there are no errors in the program code. This Gaussian Filter Generation program presented here is designed to generate a 5×5 kernel but you can modify it to generate kernel of any desired order.
Hello,
Does anybody know that why this isn’t working for 7X7 or 9X9 kernel? After 11 value all value becomes zero.