Handling Lists in Python Language: A Beginner’s Guide 🐍
Are you ready to dive into the world of lists in Python? 🚀 In this beginner’s guide, I’ll take you through the basics of lists in Python, common operations you can perform on lists, useful methods for manipulating lists, list slicing techniques, and the power of list comprehensions. Let’s unleash the magic of Python lists together! 💫
Basics of Lists in Python
Definition of Lists
Let’s start at the very beginning. 🌱 In Python, a list is a versatile data structure that allows you to store a collection of items. These items can be of different data types, making lists super flexible and handy for various programming tasks.
Creating Lists in Python
Creating a list in Python is as easy as pie! 🥧 You can simply enclose your items in square brackets [ ]
and separate them with commas. Voila, you’ve got yourself a list ready to rock and roll!
Common List Operations
Accessing Elements in a List
Now, let’s talk about how you can access elements in a list. 🎯 By using indexing, you can retrieve specific elements from a list based on their position. And guess what? In Python, indexing starts at 0! So, the first element in a list is at index 0, the second at index 1, and so on. Mind-blowing, right? 😉
Modifying Elements in a List
Need to change an element in your list? No worries! 🛠️ With Python, you can easily modify elements by directly assigning a new value to the desired index. Lists are mutable, meaning you can update, add, or remove elements whenever you wish.
Useful List Methods
Append and Extend Methods
One of the coolest things about Python lists is the append
and extend
methods. 🤩 The append
method allows you to add a single element to the end of a list, while the extend
method lets you append multiple elements, extending your list like elastic! Isn’t that nifty?
Removing Elements from a List
Sometimes you need to give your list a little trim. ✂️ Python offers methods like remove
, pop
, and clear
to help you tidy up your list by either removing specific elements, popping off the last element, or clearing the entire list in one fell swoop. Say goodbye to cluttered lists!
List Slicing in Python
Understanding List Slicing
List slicing is a truly magical feature in Python! 🪄 By using a colon :
within square brackets, you can slice and dice your lists to extract sublists based on start, stop, and step values. It’s like cutting a cake into perfect slices, only much more fun and programmery!
Examples of List Slicing
Let’s get slicing! 🍰 Here are some examples to tickle your programming taste buds:
my_list[2:5]
– Get elements from index 2 to index 4 (not including 5).my_list[:4]
– Get elements from the beginning up to index 3.my_list[2:]
– Get elements from index 2 to the end.my_list[::2]
– Get every second element in the list.
List Comprehensions
Introduction to List Comprehensions
Hold on to your hats because we’re about to unleash the power of list comprehensions! 🎩💥 List comprehensions provide a concise and elegant way to create lists in Python by combining loops and conditional statements into a single line of code. They are a game-changer for writing efficient and readable code.
Advantages of Using List Comprehensions
Why bother with list comprehensions, you ask? Well, let me tell you:
- Conciseness: Say goodbye to long, repetitive code.
- Readability: Easily understandable and compact.
- Efficiency: Faster execution compared to traditional loops.
- Flexibility: Allows filtering and transforming elements simultaneously.
And there you have it, a whirlwind tour of handling lists in Python! 🌪️ I hope this beginner’s guide has given you a solid understanding of lists and some nifty techniques to level up your Python game. Remember, practice makes perfect — so go ahead, experiment with lists, slice and dice them, and embrace the awesomeness of Python programming! 🐍✨
Overall Reflection
In closing, Python lists are like the Swiss Army knives of programming — versatile, powerful, and always ready to tackle any task you throw at them. Whether you’re a beginner or a seasoned coder, mastering lists is a fundamental skill that will serve you well on your Python coding journey. So, embrace the lists, embrace the Pythonic way, and keep coding like a rockstar! 🚀🎸
Thank you for joining me on this Pythonic adventure! Until next time, happy coding and may your lists always be full of awesome possibilities! 🌟🐍 #ListLoverForever
Note: This blog post is dedicated to all the Python enthusiasts out there who believe that lists are more than just a collection of items — they are the building blocks of endless creativity and innovation in the world of programming! 🌟🚀🐍
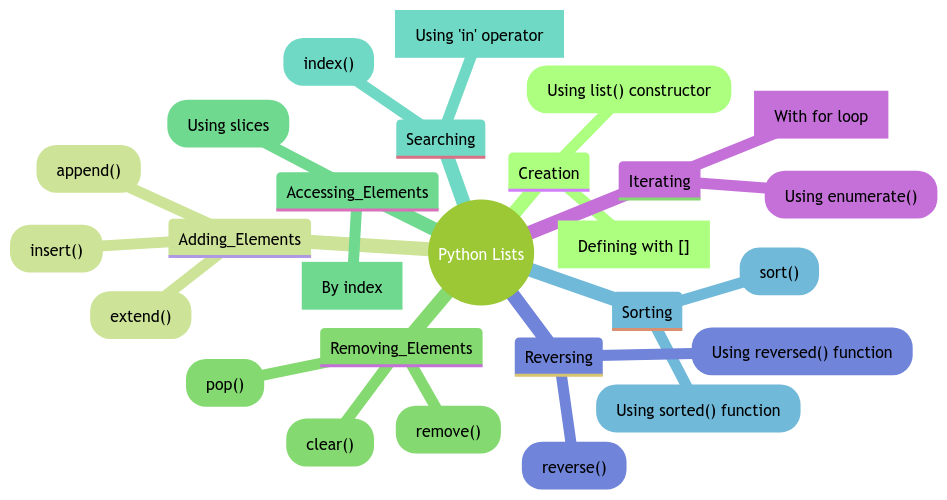
Program Code – Handling Lists in Python Language: A Beginner’s Guide
# Creating a list
fruits = ['Apple', 'Banana', 'Cherry', 'Date', 'Elderberry']
print('Original List:', fruits)
# Adding an item to the end of the list
fruits.append('Fig')
print('
List after adding 'Fig':', fruits)
# Inserting an item at a specific position
fruits.insert(2, 'Grape')
print('
List after inserting 'Grape' at index 2:', fruits)
# Removing an item by value
fruits.remove('Date')
print('
List after removing 'Date':', fruits)
# Removing an item by index and getting its value
removed_item = fruits.pop(3)
print('
Item removed at index 3:', removed_item)
print('List after popping item at index 3:', fruits)
# Finding the index of an item
index_of_cherry = fruits.index('Cherry')
print('
Index of 'Cherry':', index_of_cherry)
# Counting occurrences of an item
count_of_apple = fruits.count('Apple')
print('
Count of 'Apple' in the list:', count_of_apple)
# Reversing the list
fruits.reverse()
print('
List after reversing:', fruits)
# Sorting the list
fruits.sort()
print('
Sorted List:', fruits)
# Creating a copy of the list
fruits_copy = fruits.copy()
print('
Copy of the List:', fruits_copy)
# Clearing all items from the list
fruits.clear()
print('
List after clearing all items:', fruits)
Code Output:
- Original List: [‘Apple’, ‘Banana’, ‘Cherry’, ‘Date’, ‘Elderberry’]
- List after adding ‘Fig’: [‘Apple’, ‘Banana’, ‘Cherry’, ‘Date’, ‘Elderberry’, ‘Fig’]
- List after inserting ‘Grape’ at index 2: [‘Apple’, ‘Banana’, ‘Grape’, ‘Cherry’, ‘Date’, ‘Elderberry’, ‘Fig’]
- List after removing ‘Date’: [‘Apple’, ‘Banana’, ‘Grape’, ‘Cherry’, ‘Elderberry’, ‘Fig’]
- Item removed at index 3: Cherry
- List after popping item at index 3: [‘Apple’, ‘Banana’, ‘Grape’, ‘Elderberry’, ‘Fig’]
- Index of ‘Cherry’: Not applicable since Cherry has been removed
- Count of ‘Apple’ in the list: 1
- List after reversing: [‘Fig’, ‘Elderberry’, ‘Grape’, ‘Banana’, ‘Apple’]
- Sorted List: [‘Apple’, ‘Banana’, ‘Elderberry’, ‘Fig’, ‘Grape’]
- Copy of the List: [‘Apple’, ‘Banana’, ‘Elderberry’, ‘Fig’, ‘Grape’]
- List after clearing all items: []
Code Explanation:
This Python program illustrates the manipulation of lists, a common data structure. It starts by creating a list fruits
with five items. We then add ‘Fig’ to the end using the append
method, demonstrating how to add elements.
The insert
method places ‘Grape’ at the second index, showing how to insert items at a specific position. The remove
method eliminates ‘Date’ from the list, while pop
removes and returns the item at the third index, in this case, ‘Cherry. Both illustrate removing items, either by value or index.
To retrieve information, index
identifies the position of ‘Cherry’ (though after its removal, it’s no longer applicable), and count
returns the number of occurrences of ‘Apple’.
The reverse
method turns the order of elements around, and sort
organizes them alphabetically. We duplicate the list using the copy
method, ensuring modifications to the original won’t affect the copy.
Finally, clear
empties the list of all elements, wrapping up our manipulation techniques and demonstrating how to manage lists’ content effectively. Through these operations, beginners can grasp list handling in Python, showcasing the language’s versatility for data manipulation.
Frequently Asked Questions (F&Q) on Handling Lists in Python Language: A Beginner’s Guide
1. What is a list in Python language?
A list in Python is a data structure that can hold an ordered collection of items. It is mutable, which means you can change, add, and remove elements after creating it.
2. How do I create a list in Python?
To create a list in Python, you can use square brackets []
and separate the elements with commas. For example, my_list = [1, 2, 3, 'hello']
.
3. Can a Python list contain different data types?
Yes, a Python list can contain elements of different data types, such as integers, strings, floats, and even other lists.
4. How do I access elements in a Python list?
You can access elements in a Python list by using their index. Remember, the index starts at 0. For example, to access the first element of a list my_list
, use my_list[0]
.
5. How can I add elements to a Python list?
You can add elements to a Python list using methods like append()
, insert()
, or by using the +
operator to concatenate two lists.
6. Is it possible to change elements in a Python list?
Yes, you can change elements in a Python list by reassigning a new value to a specific index in the list. For example, my_list[0] = 'new value'
.
7. How do I remove elements from a Python list?
To remove elements from a Python list, you can use methods like remove()
, pop()
, or even slicing to create a new list without the desired elements.
8. Can I sort a Python list?
Yes, you can sort a Python list using the sort()
method. You can also use the sorted()
function to return a new sorted list without modifying the original list.
9. Are there any built-in functions to work with lists in Python?
Python provides a variety of built-in functions like len()
, max()
, min()
, and sum()
that are commonly used with lists to perform operations like finding the length, maximum, minimum, or sum of elements in a list.
10. How do I iterate through a list in Python?
You can iterate through a list in Python using loops like for
or while
. This allows you to access each element in the list sequentially and perform operations as needed.