How To Declare Simple Variables in Java?
You can declare a variable in Java without using the new keyword, just like any other variable.
Java is a language that supports object-oriented programming. One of the ways in which it supports this is that it has the concept of objects. An object is a self-contained unit of code that contains data. This data can be referred to as fields or attributes, and it can be manipulated and changed as needed.
When declaring a variable, the new keyword is used. However, there is no requirement to use the new keyword. Instead, you can create an object using the Object class. You will need to add code to the constructor method to set values within the object.
Declaring a Variable in Java
To declare a variable, you will first need to create a variable name and an initial value. The following example shows how to declare and assign a variable named age.
public class VariableDeclaration {
private int age;
public void variableDeclaration() {
// This is a variable declaration.
// We declare a variable named age and initialize it to 42.
age = 42;
}
}
The age variable will be initialized to 42 when the variable declaration method is called.
The following example shows how to declare and assign a variable named height.
public class VariableDeclaration {
private double height;
public void variableDeclaration() {
// This is a variable declaration.
// We declare a variable named height and initialize it to 160.
height = 160;
}
}
The height variable will be initialized to 160 when the variable declaration method is called.
In the previous examples, both variables were assigned to a numeric type, double. A different type of variable may be declared. The following example shows how to declare and assign a variable named type.
public class VariableDeclaration {
private String type;
public void variableDeclaration() {
// This is a variable declaration.
// We declare a variable named type and initialize it to Student.
type = Student.class.getName();
}
}
In the previous example, the type variable was assigned to a String.
There are two types of variables in Java, primitive and reference. Primitive variables have a fixed size. For example, int has a fixed size of 32 bits. Integer has a fixed size of 64 bits. In addition, the size of a reference variable is determined by the reference type that it holds. The reference type may be char, byte, short, int, long, float, double, or boolean.
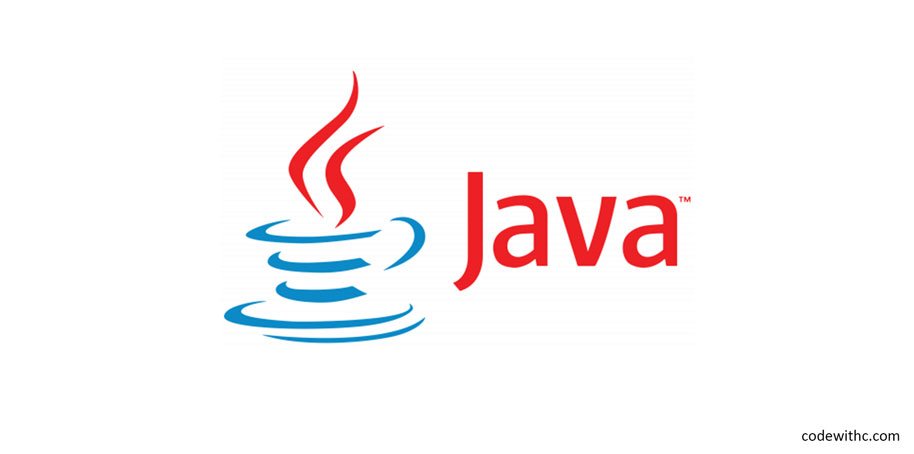
Object Reference in Java
An object reference is created using the new operator. This is the most common way to create objects in Java. The following example shows how to declare and assign a variable named animal.
public class AnimalReference
{
public static void main(String[] args)
{
// This is an animal reference.
// We create an animal and assign it to a dog reference.
Animal dog = new Animal("Jimmy");
// The dog reference is now an object.
// It contains data about the dog.
// In this case, it contains the dog's name.
AnimalReference ref = new AnimalReference();
ref.animal.name = "Jimmy";
System.out.println(ref.animal.name);
}
}
Here, we create an object named dog, and assign it to a dog reference. The ref variable contains the data about the dog. The ref variable is also an object.
Constructor in Java
The constructor is the method that is called when you create an object. It is called automatically, but it may be used to initialize any data that you wish to have with the object. For example, it is possible to set the name of the dog in the previous example using the constructor.
public class AnimalReference {
public static void main(String[] args) {
// This is an animal reference.
// We create an animal and assign it to a dog reference.
Animal dog = new Animal("Jimmy");
// The dog reference is now an object.
// It contains data about the dog.
// In this case, it contains the dog's name.
AnimalReference ref = new AnimalReference();
ref.animal.name = "Jimmy";
System.out.println(ref.animal.name);
}
public Animal animal;
public AnimalReference() {
// Here, we are creating an instance of the Animal class.
// The dog reference is created and initialized to an instance of the Dog class.
animal = new Dog("Doe");
}
}
In the previous example, we create a new object using the new operator and assign it to the ref variable. The ref variable contains data about the dog. The ref variable is also an object. When the ref variable is initialized, the animal variable is also initialized.
Object Initialization
To initialize a variable, we call the variable’s constructor. For example, if we want to initialize the height of the dog, we will call the AnimalReference constructor, as shown in the previous example.
Declarations for Primitive and String Types
public class DeclarationsExample {
public static void main (String[] args) {
boolean BooleanVal = true; /* Default is false */
char charval = 'G'; /* Unicode UTF-16 */
charval = '\u0490'; /* Ukrainian letter Ghe(Ґ) */
byte byteval; /* 8 bits, -127 to 127 */
short shortval; /* 16 bits, -32,768 to 32,768 */
int intval; /* 32 bits, -2147483648 to 2147483647 */
long longval; /* 64 bits, -(2^64) to 2^64 - 1 */
float floatval = 10.123456F; /* 32-bit IEEE 754 */
double doubleval = 10.12345678987654; /* 64-bit IEEE 754 */
String message = "Darken the corner where you are!";
message = message.replace("Darken", "Brighten");
}
}
The concept of visibility applies to Variables. After they have been created, they are visible from the main method and then dealt with when the method ends. They are not accessible from outside of the main method.
Conclusion:
A variable can be defined by the name of the data type. There are two types of variables that are used in this regard, Integer and Boolean. Integer is the simplest type of data, which can be defined as an integer or a whole number. The other type of variable is Boolean. Boolean is the type of data that indicates whether a condition is true or false.