Programming is a skill that can never be mastered. There are always new ways of writing programs and there are always new ways to learn to program. But one thing is for sure, that programming is a skill that will always stay with you. It is the only way to express our ideas and our thoughts in a different language.
Literal expressions are the simplest form of expression in programming languages. They are used to express something that is a fixed value. They are used to create constants, and they can be used to represent numbers, dates, times, and other types of data.
But there are a lot of different ways that you can use them, and not all of them are the same. So, this tutorial will cover the basics of literal expressions in C++ programming.
Constant expressions
Constant expressions can be defined as an expression that represents a fixed value. There are two types of constant expressions in C++: literal and compile-time constant expressions.
Literal Expression
A literal is a kind of expression that contains a single value. If you are familiar with algebra, then you will know what I am saying. A literal is written as ‘(1+2)*3’. A literal can be an integer, a character, a string, a floating-point number, etc. For example, 82 is a literal, whereas X isn’t always because one needs to see its declaration to understand its type and read previous lines of code to know its price.
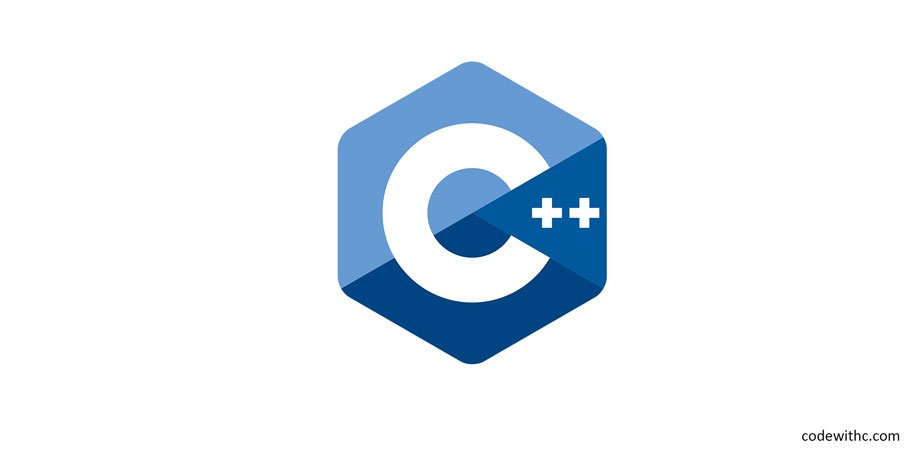
Why Use Literals?
Literal expressions are useful because they can be used to create constants.
This means that you can store a value in a variable, and then use that value later. But you can also use literal expressions to create constants.
And if you want to create a constant, then you can use a literal expression. For example, you could create a constant named “CITY_NAME” that represents the name of your city.
You can use literal expressions to create constants because they can be used to represent different types of data.
For example, you could use a literal expression to create a constant named “DATE” that represents the date of the last day of the month.
You could also use a literal expression to create a constant named “TIME” that represents the current time.
What is Literal octal?
Literal octal is the use of octal digits in literals. The octal number system is used for representing integers. Literal octal is also called octal literal. Octal literal is a kind of literal that represents the integer value using octal digits. Literal octal is useful when you need to specify a large number of octal digits.
For example, suppose we want to specify a large number of octal digits.
We can write the literal octal as shown below.
int main()
{
int x = 077;
}
How to create Literal octal?
In the above example we have specified the literal octal as 077. To create a literal octal, we need to use the following format.
0b
0b means binary.
So we can use binary literal to create octal literal.
For example, if we want to create a literal octal of 7 digits, we can write the following code.
int main()
{
int x = 0b01111111;
}
If we want to create a literal octal of 8 digits, we can write the following code.
int main()
{
int x = 0b011111110001;
}
How to convert Literal octal to Decimal?
To convert a literal octal to decimal, we need to first understand the conversion process. Let’s see how it works. First we need to divide the literal octal into two parts. Then we need to multiply the second part by eight. Finally we add the result to the first part.
int main()
{
int x = 077;
int y = x/8;
int z = y*8;
int w = z + x;
}
Here is the result.
x = 077
y = 5
z = 5*8 = 40
w = 40 + 077 = 407
As you can see, the literal octal is converted to decimal as shown above.
How to convert Literal octal to Hexadecimal?
To convert a literal octal to hexadecimal, we need to use the following format.
0o
0o means octal.
For example, if we want to create a literal octal of 8 digits, we can write the following code.
int main()
{
int x = 0o77;
}
If we want to create a literal octal of 9 digits, we can write the following code.
int main()
{
int x = 0o7777;
}
How to convert Literal octal to Binary?
To convert a literal octal to binary, we need to use the following format.
0b
0b means binary.
For example, if we want to create a literal octal of 8 digits, we can write the following code.
int main()
{
int x = 0b01111111;
}
If we want to create a literal octal of 9 digits, we can write the following code.
int main()
{
int x = 0b011111110001;
}
How to Convert Literal Octal to Decimal?
To convert a literal octal to decimal, we need to first understand the conversion process. Let’s see how it works. First we need to divide the literal octal into two parts. Then we need to multiply the second part by eight. Finally we add the result to the first part.
int main()
{
int x = 077;
int y = x/8;
int z = y*8;
int w = z + x;
}
Here is the result.
x = 077
y = 5
z = 5*8 = 40
w = 40 + 077 = 407
As you can see, the literal octal is converted to decimal as shown above.
How to Convert Literal Octal to Hexadecimal?
To convert a literal octal to hexadecimal, we need to use the following format.
0o
0o means octal.
For example, if we want to create a literal octal of 8 digits, we can write the following code.
int main()
{
int x = 0o77;
}
If we want to create a literal octal of 9 digits, we can write the following code.
int main()
{
int x = 0o7777;
}
How to Convert Literal Octal to Binary?
To convert a literal octal to binary, we need to use the following format.
0b
0b means binary
For example, if we want to create a literal octal of 8 digits, we can write the following code.
int main()
{
int x = 0b01111111;
}
If we want to create a literal octal of 9 digits, we can write the following code.
int main()
{
int x = 0b011111110001;
}