Looping Made Simple: Python Programming for Loop Explained 👩🏽💻
In the vast world of Python programming, loops are like the trusty sidekicks that help you navigate through repetitive tasks with ease. Whether you’re a coding newbie or a seasoned developer, understanding loops in Python is crucial for writing efficient and clean code. Today, we’re diving deep into the realm of loops to unravel the mysteries behind ‘for’ and ‘while’ loops while having a barrel of laughs along the way! 🚀
Basics of Python Programming for Loop
Before we embark on our loop-drenched journey, let’s shake hands with the basics of Python loops and get cozy with the concept of repetitive execution. Imagine you have to do something over and over again in your code – that’s where loops shine! They save you from the dreary task of writing the same lines of code repeatedly. So, let’s roll up our sleeves and dig deeper!
Introduction to Python Loops
Python loops are like the energizer bunnies of programming; they keep going and going until a specific condition is met. With loops, you can iterate over a sequence of elements, be it a list, tuple, or even a string. No more manual tedium – let Python do the heavy lifting for you!
Different types of loops in Python
In Python, you have two main loop buddies – ‘for’ and ‘while’. Each comes with its quirks and perks, ready to assist you in your coding quests. Let’s grab our magnifying glass and take a closer look at these dynamic duo of loops!
Understanding Python ‘for’ Loop
Ah, the ‘for’ loop – a loyal companion that marches through each item in a sequence, be it a list, tuple, or range. Its syntax is as sweet as a piece of cake, making it a favorite among Pythonistas.
Syntax of ‘for’ loop
The syntax of the ‘for’ loop is as simple as ABC – no rocket science here! You just need to define your iterator variable and the sequence you want to iterate over. Let’s cook up some Python magic with the ‘for’ loop!
Examples of ‘for’ loop in Python
Let’s sprinkle some Python fairy dust with a couple of examples:
- Looping through a list of fruits 🍎🍌
- Iterating over a range of numbers 🔢
Mastering Python ‘while’ Loop
Now, say hello to the ‘while’ loop – the wild child of loops that keeps executing until a specified condition is met. It’s like having a persistent friend who won’t leave until you say the magic word! 😉
Syntax of ‘while’ loop
The ‘while’ loop’s syntax might seem a bit rebellious, but trust me, it’s as charming as a mischievous puppy. With a condition to check and a block of code to execute, the ‘while’ loop keeps the party going until you pull the plug.
Examples of ‘while’ loop in Python
Get ready for some Python acrobatics with these examples:
- Countdown to blast off 🚀
- Keep looping until a specific condition is met 🎯
Common Mistakes to Avoid in Python Loops
As you dance your way through Python loops, watch out for these common pitfalls that can trip you up along the coding path. Let’s dodge those banana peels like a pro!
- Infinite loops: Beware of loops that never end, sucking your program into a black hole of eternal execution. Remember to include a break condition to escape the loop’s clutches!
- Forgetting to update loop variables: Don’t get stuck in a Groundhog Day scenario by neglecting to update your loop variables. Keep them fresh and kicking to avoid stagnation!
Tips and Tricks for Efficient Looping in Python
Now that you’ve tamed the loop dragons, it’s time to level up your Python loop game with some nifty tips and tricks. These secrets will turbocharge your loop efficiency and make your code sparkle like a unicorn!
- Using list comprehensions: Unleash the power of list comprehensions to condense your loops into elegant one-liners. It’s like turning a bulky caterpillar into a sleek butterfly!
- Leveraging built-in functions like ‘enumerate’ and ‘zip’: Dive into the toolbox of Python’s built-in functions like ‘enumerate’ and ‘zip’ to supercharge your loop maneuvers. They’ll make your loops sing like a symphony!
In closing, looping in Python isn’t just about repeating yourself; it’s about dancing through code with finesse and flair. Embrace the loop symphony, conduct it with confidence, and watch your programs come alive in a dazzling performance! Thanks for joining me on this loop-de-loop adventure, and remember, keep coding and keep smiling! 🌟
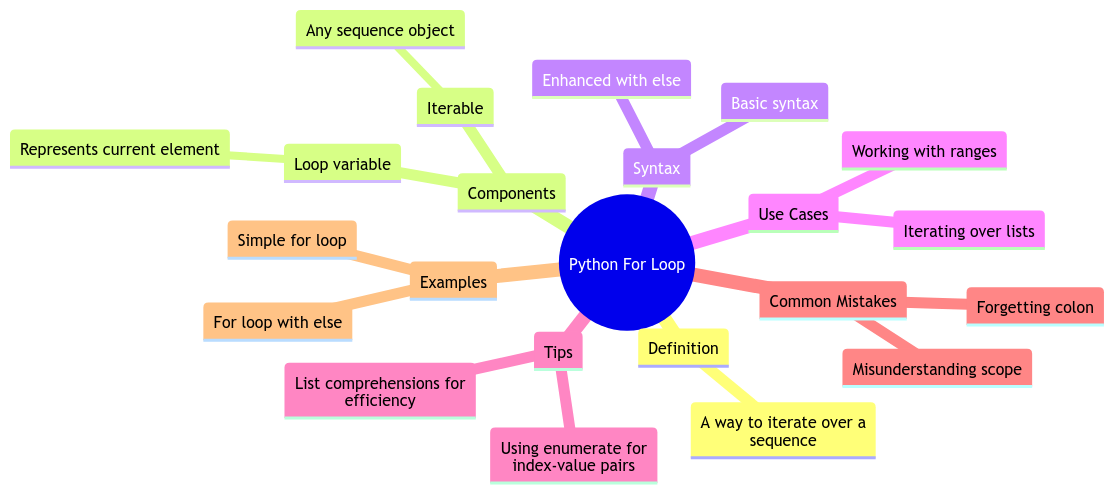
Program Code – Looping Made Simple: Python Programming for Loop Explained
# Python Program Demonstrating Various Uses of For Loop
# 1. Simple for loop to print numbers from 0 to 4
print('Simple for loop:')
for i in range(5):
print(i, end=' ')
print('
----------')
# 2. For loop with else
print('For loop with else:')
for i in range(5):
print(i, end=' ')
else:
print('
Loop completed successfully.')
print('----------')
# 3. Nested for loop to generate a pattern
print('Nested for loop for pattern generation:')
rows = 3
for i in range(1, rows + 1):
for j in range(1, i + 1):
print('*', end=' ')
print('')
print('----------')
# 4. For loop with enumerate to track list indices and values
print('For loop with enumerate for indices and values:')
names = ['John', 'Jane', 'Doe']
for index, name in enumerate(names):
print(f'Index: {index}, Name: {name}')
print('----------')
# 5. For loop with list comprehension
print('For loop with list comprehension:')
squares = [x**2 for x in range(10)]
print('Squares of numbers from 0 to 9:', squares)
Code Output:
Simple for loop:
0 1 2 3 4
----------
For loop with else:
0 1 2 3 4
Loop completed successfully.
----------
Nested for loop for pattern generation:
*
* *
* * *
----------
For loop with enumerate for indices and values:
Index: 0, Name: John
Index: 1, Name: Jane
Index: 2, Name: Doe
----------
For loop with list comprehension:
Squares of numbers from 0 to 9: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
Code Explanation:
The given Python program showcases various applications of ‘for loop’ in Python programming, elucidating its adaptability and simplicity.
- Simple for Loop: The first part prints numbers from 0 to 4 using a straightforward for loop, incorporating
range(5)
which generates numbers from 0 up to (but not including) 5. - For Loop with Else: This segment demonstrates an often underused feature of for loops – the ‘else’ block. This block executes after the for loop concludes naturally (not broken by a ‘break’ statement), signifying the loop’s successful completion.
- Nested For Loop: Illustrated here is a nested for loop creating a simple triangle pattern with asterisks. The outer loop manages the row number, while the inner loop handles the printing of asterisks per row.
- For Loop with Enumerate: This example uses ‘enumerate’ to loop over a list while keeping track of the index and the item. This is particularly useful for situations where both the item and its index are needed.
- For Loop within List Comprehension: The final example showcases the power of list comprehension for creating lists in a concise manner. A for loop inside the list comprehension calculates squares of numbers from 0 to 9, illustrating both efficiency and elegance.
This program demonstrates the versatile nature of for loops in Python programming, illustrating its potential in simplifying tasks, optimizing code, and enhancing readability.
Frequently Asked Questions (F&Q)
What is a for loop in Python programming?
A for loop in Python is used to iterate over a sequence of elements, such as a list, tuple, or string. It allows you to execute a block of code multiple times without having to write the same code for each iteration.
How does a for loop work in Python?
In Python, a for loop iterates over each item in a sequence, executing the block of code within the loop for each item. The loop continues until all items in the sequence have been processed.
Can you provide an example of a for loop in Python?
Sure! Here’s an example of a simple for loop in Python that prints each element of a list:
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
What is the difference between a for loop and a while loop in Python?
The main difference between a for loop and a while loop in Python is that a for loop is used for iterating over a sequence of elements, while a while loop is used for executing a block of code repeatedly as long as a certain condition is met.
How do you use the range() function with a for loop in Python?
The range() function in Python generates a sequence of numbers that can be used with a for loop. You can specify the start, stop, and step size of the sequence within the range() function to control the iteration.
Can you nest for loops in Python?
Yes, you can nest for loops in Python by placing one or more for loops inside another for loop. This allows you to iterate over multiple sequences or create more complex iteration patterns.
Are there any best practices for using for loops in Python programming?
It’s recommended to use descriptive variable names in for loops to improve code readability. Additionally, it’s important to avoid modifying the sequence you are iterating over within the loop to prevent unexpected behavior.