Merge Sort: Breaking Down the Sorting Algorithm for Efficiency 💡
Hey there, tech enthusiasts! Today, we’re diving into the fascinating world of sorting algorithms, focusing on the ever-efficient Merge Sort. 🚀 Let’s unravel this algorithm piece by piece with a sprinkle of humor and a dash of knowledge!
Understanding Merge Sort
Definition of Merge Sort 📚
Imagine you have a messy room and you need to organize it. Merge Sort is like having a magical sorting wand that helps you neatly arrange all the clutter in ascending or descending order. It’s a divide-and-conquer algorithm that recursively divides the unsorted list until each sublist contains only one element. Then, it conquers by merging those sublists back together in a sorted manner. Voilà! 🪄
How Merge Sort works 🛠️
Merge Sort operates in three simple steps:
- Divide: The unsorted list is divided into two equal halves until individual elements are reached.
- Conquer: Each divided sublist is sorted.
- Merge: The sorted sublists are merged back together in the correct order.
Advantages of Merge Sort
Stable Sorting Algorithm 🦄
One of the magical qualities of Merge Sort is its stability. Unlike some algorithms that might shuffle equal elements during sorting, Merge Sort maintains the relative order of equal elements, making it a reliable choice for various applications.
Efficiency of Merge Sort 💪
Merge Sort shines bright in the efficiency department! It boasts a time complexity of O(n log n), making it a top contender for sorting large datasets swiftly and effectively. Who says sorting has to be a slow and tedious affair? Merge Sort says, "Not on my watch!" 🕒
Implementing Merge Sort
Divide and Conquer Strategy 🤖
Merge Sort follows the age-old wisdom of "divide and conquer." By breaking down the problem into smaller, more manageable chunks, Merge Sort tackles the sorting puzzle with finesse and precision. It’s like solving a jigsaw puzzle—piece by piece until the bigger picture emerges! 🧩
Merging Process in Merge Sort 🌀
The merging step in Merge Sort is where the real magic unfolds. It’s like orchestrating a symphony where each element finds its perfect place in the sorted array. The merging phase elegantly combines the sorted sublists to create a harmonious melody of order. Bravo! 🎻
Comparing Merge Sort with Other Sorting Algorithms
Merge Sort vs. Quick Sort 🐇🐢
Quick Sort might be speedy like the hare, but Merge Sort is the steady tortoise of sorting algorithms. While Quick Sort boasts an average-case time complexity of O(n log n), its worst-case scenario can be a bit unpredictable. Merge Sort, on the other hand, maintains consistent efficiency in all cases.
Merge Sort vs. Bubble Sort 💭💬
Ah, Bubble Sort—the algorithm we’ve all encountered as coding newbies. Bubble Sort’s charm lies in its simplicity, but Merge Sort takes the crown for efficiency and speed. Bubble Sort’s time complexity of O(n^2) might leave you waiting for ages, while Merge Sort zips through the sorting process like a pro! 🎩
Applications of Merge Sort
Real-life Examples 🌟
Merge Sort isn’t just a theoretical concept—it’s a real-world hero! From organizing vast libraries of books to sorting massive databases, Merge Sort plays a crucial role in various applications where efficiency and stability are paramount.
Where Merge Sort is commonly used 🌐
Merge Sort finds its way into diverse fields, including:
- External Sorting: Sorting huge datasets that don’t fit into memory.
- Parallel Computing: Leveraging multiple processors for lightning-fast sorting.
- Optimizing Merge Operations: Enhancing efficiency in database operations for improved performance.
In closing, Merge Sort’s efficiency, stability, and elegance make it a standout player in the world of sorting algorithms. So, next time you need to sort a chaotic mess of data, remember the magic of Merge Sort! ✨ Thanks for tuning in, techies—stay curious, stay coding! 🚀🌈
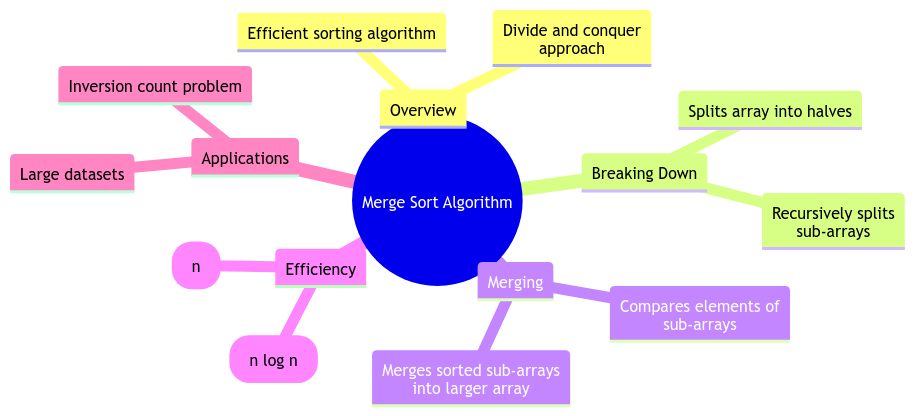
Program Code – Merge Sort: Breaking Down the Sorting Algorithm for Efficiency
def merge_sort(arr):
'''
This function implements the merge sort algorithm.
It recursively divides the array into halves, sorts each half, and merges them.
'''
if len(arr) > 1:
mid = len(arr) // 2 # Find the midpoint of the array.
L = arr[:mid] # Dividing the array into 2 halves.
R = arr[mid:]
merge_sort(L) # Sorting the first half.
merge_sort(R) # Sorting the second half.
i = j = k = 0
# Copy data to temporary arrays L[] and R[].
while i < len(L) and j < len(R):
if L[i] < R[j]:
arr[k] = L[i]
i += 1
else:
arr[k] = R[j]
j += 1
k += 1
# Checking if any element was left.
while i < len(L):
arr[k] = L[i]
i += 1
k += 1
while j < len(R):
arr[k] = R[j]
j += 1
k += 1
# Example usage
arr = [12, 11, 13, 5, 6, 7]
merge_sort(arr)
print('Sorted array is: ', arr)
Code Output:
Sorted array is: [5, 6, 7, 11, 12, 13]
Code Explanation:
The Merge Sort algorithm is a perfect example when it comes to showing the might of divide-and-conquer strategies in programming. Starting off, let’s break down the essence of this program:
-
Divide and Conquer: The first order of business for merge sort is diving the problem into smaller chunks. This is achieved by finding the middle of the array to divide the array into left and right halves (L, R). This is a recursive process until each piece is a manageable unit of one element.
-
Recursive Sorting: With both halves identified (L and R), the function calls itself for each half. This recursion happens until the base condition of the array length being greater than one fails. It’s like hitting the atom at its core – once we’re at the smallest unit, it’s time to start building up.
-
Merging: This is where the ‘conquer’ part of ‘divide and conquer’ comes in. Both halves have been independently sorted. Picture this: you’ve got two piles of books sorted by author’s last name; now you need to merge these piles while keeping the overall order intact. This is done through a series of comparisons and temporary arrays to hold the sorted elements until they can be moved back into the original array.
-
Copying Remaining Elements: In a perfect world, both halves would run out of items at the exact same time. However, in reality, one half might finish first. This leftover scenario is accounted for by simply iterating through the remaining elements and adding them back to the main array.
The beauty of merge sort is its time complexity of O(n log n), making it disastrously efficient for large datasets. Plus, the way it intricately weaves together splitting, sorting, and merging, much like knitting a sweater from a single thread, is nothing short of programming poetry.
Overall, the merge sort algorithm, with its robust yet elegant approach to sorting, perfectly showcases the power of recursive functions and the divide-and-conquer strategy. It’s no wonder coding aficionados and data scientists alike swoon over its efficiency and reliability in taming even the most unruly of arrays. 🚀
Thanks for tagging along on this code-filled adventure. Remember, in a world full of sorting dilemmas, be the merge sort! 😉
Frequently Asked Questions about Merge Sort: Breaking Down the Sorting Algorithm for Efficiency
What is Merge Sort and how does it work?
Merge Sort is a popular sorting algorithm that follows the divide-and-conquer strategy. It works by dividing the unsorted list into smaller sublists, sorting those sublists, and then merging them back together in the correct order.
Why is Merge Sort considered efficient?
Merge Sort is considered efficient because it has a time complexity of O(n log n), where n is the number of elements in the list. This means that it can handle large datasets without a significant increase in time complexity.
Are there any drawbacks to using Merge Sort?
While Merge Sort is efficient in terms of time complexity, it does require additional space to store the temporary sublists during the sorting process. This can be a drawback when dealing with limited memory resources.
How does Merge Sort compare to other sorting algorithms like Quick Sort or Bubble Sort?
Merge Sort outperforms algorithms like Bubble Sort in terms of efficiency and scalability. It is often compared to Quick Sort, which is also efficient but may have a higher worst-case time complexity compared to Merge Sort.
Can Merge Sort be used to sort different types of data structures?
Yes, Merge Sort can be used to sort various data structures, including arrays, linked lists, and trees. Its versatility makes it a popular choice for sorting algorithms.
Is Merge Sort a stable sorting algorithm?
Yes, Merge Sort is a stable sorting algorithm, which means that it maintains the relative order of equal elements in the sorted list. This makes it suitable for scenarios where maintaining the original order of equal elements is important.
Are there any real-world applications where Merge Sort is commonly used?
Merge Sort is commonly used in scenarios where a stable sorting algorithm with a predictable time complexity is required. It is often used in programming languages for sorting large datasets efficiently.