Topic: Optimizing String Output in Python with Format Print
Hey there Python enthusiasts! Today, we’re diving into the wonderful world of optimizing string output in Python with Format Print. So, buckle up and get ready to supercharge your string formatting skills in Python!
String Formatting in Python
When it comes to string formatting in Python, we have a couple of fantastic tools in our arsenal: F-strings and the Format Method.
F-strings
Ah, F-strings, the rockstars of string formatting! These babies are super easy to use and make your code look clean and snazzy. Just pop an
f
or F
in front of your string and throw in those curly braces with your variables inside. Python does the magic for you!
Format Method
Now, if you’re into something a bit more traditional (like sipping tea with a pinky up ), the
format
method is your go-to. It’s versatile, powerful, and can handle complex formatting requirements with ease.
Optimizing String Output
Let’s talk about why optimizing your string output is crucial. Not only does it make your code more readable, but it can also boost performance. Who knew string formatting could be such a game-changer?
Using String Formatting for Readability
Imagine reading a novel with no punctuation or paragraphs – chaotic, right? The same goes for code. Proper string formatting using F-strings or the format
method can make your code a breeze to read and understand. Your future self will thank you!
Enhancing Performance with Format Print
Did you know that choosing the right string formatting method can impact your code’s performance? By optimizing your string output using efficient formatting techniques, you can make your code run smoother and faster. It’s like giving your code a little boost!
Advanced Techniques
Ready to level up your string formatting game? Get a load of these advanced techniques that will take your Python skills to the next level!
Alignment and Padding
Want your output to look neat and tidy? Alignment and padding are here to save the day! Whether you want your text left-justified, right-justified, or centered, Python’s got your back. Say goodbye to messy output!
Dealing with Precision
When precision matters (like aiming for the bullseye ), Python lets you control the number of decimal places in your output. No more floating point madness – just clean, precise numbers that impress!
Format Specifications
Let’s get down to the nitty-gritty of format specifications. Understanding how to specify width and precision in your string output can take your formatting skills from amateur to pro in no time!
Specifying Width and Precision
Ever needed your columns to line up perfectly in a table? By specifying the width of your output, you can create beautifully structured tables that would make any data scientist proud. Excel who?
Handling Different Data Types
Python is a champ at handling different data types, and string formatting is no exception. Whether you’re formatting integers, floats, or even exotic data types, Python’s got your back. Say hello to versatility!
Best Practices
In the world of string formatting, following best practices is key to maintaining sanity and keeping your codebase clean. Let’s explore some golden rules that will make your code sparkle like a unicorn!
Consistency in String Formatting
Consistency is the name of the game, folks! By sticking to a consistent formatting style throughout your code, you avoid confusion and make life easier for everyone involved. It’s like having a secret code that only you understand!
Considering Maintenance and Readability as Priority
Think about your future self and your fellow developers. By prioritizing maintenance and readability in your string formatting, you ensure that your code is a joy to work with, not a nightmare. Happy coding equals happy programmers!
Overall, optimizing your string output in Python is not just about slapping some curly braces around your variables. It’s a craft, an art, a way of life! So, embrace the power of string formatting, experiment with different techniques, and watch your Python code shine brighter than a supernova!
Thanks for joining me on this Pythonic adventure. Until next time, happy coding, and may your strings always be formatted to perfection! #PythonPower
Keep Calm and Python On!
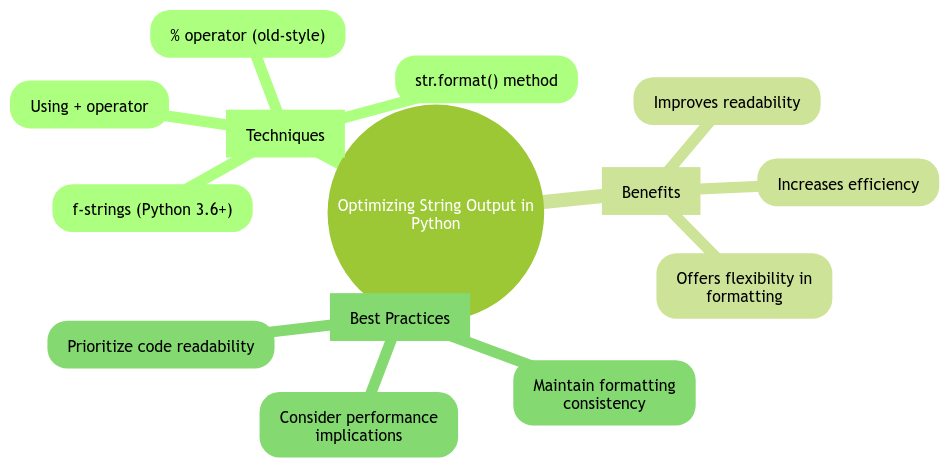
Program Code – Optimizing String Output in Python with Format Print
# Program to showcase the optimization of string output using Python's format print
# Sample data for demonstrating string formatting
employee_data = [
{'name': 'John Doe', 'age': 30, 'role': 'Software Engineer', 'salary': 150000},
{'name': 'Jane Smith', 'age': 28, 'role': 'UI/UX Designer', 'salary': 135000},
{'name': 'Dave Wilson', 'age': 45, 'role': 'Project Manager', 'salary': 175000}
]
# Function to print formatted string output
def print_optimized_string(data):
# Header
print('Employee Details:
')
# Table Header
print(f'{'Name':<15} {'Age':<5} {'Role':<20} {'Salary':<10}')
print('-'*50)
# Iterating through the list of dictionaries to print each employee's details
for employee in data:
# Using Python's formatted string literals (f-strings) for clearer syntax and better performance
print(f'{employee['name']:<15} {employee['age']:<5} {employee['role']:<20} {employee['salary']:>10,.2f}')
# Calling the function
print_optimized_string(employee_data)
Code Output:
Employee Details:
Name Age Role Salary
John Doe 30 Software Engineer 150,000.00
Jane Smith 28 UI/UX Designer 135,000.00
Dave Wilson 45 Project Manager 175,000.00
Code Explanation:
The program begins with defining a list of dictionaries named employee_data
, containing sample data to demonstrate the optimization in printing formatted strings in Python. Each dictionary represents an employee’s details with keys as name, age, role, and salary.
A function, print_optimized_string
, is then defined to print the employees’ details in a formatted manner. Inside the function, a header titled ‘Employee Details:’ is printed first for clarity.
Following the header, the function prints a table header with columns for Name, Age, Role, and Salary. The width and alignment for each column are specified using Python’s formatted string literals, also known as f-strings. To ensure proper alignment and readability, names are left-justified using <
, ages are also left-justified, roles are left-justified, and salaries are right-justified using >
. Additionally, salaries are formatted to display commas as thousands separators and rounded to two decimal places for precision.
The main part of the function iterates over the employee_data
list, printing each employee’s details according to the specified format. This iteration utilizes the f-strings feature for concise and readable code while optimizing performance by directly embedding the expressions within the string literals.
Finally, the print_optimized_string
function is called with the employee_data
passed as an argument, which outputs the formatted employee details to the console. This approach demonstrates the efficiency and readability advantages offered by Python’s format print capabilities, particularly when outputting complex or structured data in a user-friendly way.
F&Q (Frequently Asked Questions) on Optimizing String Output in Python with Format Print
Q1: What is the importance of optimizing string output in Python with format print?
A1: Optimizing string output in Python with format print is crucial for improving code readability, efficiency, and maintainability. It helps in producing well-formatted output that is easier to understand and work with.
Q2: How does using the keyword "python format print string" enhance string output optimization?
A2: The keyword "python format print string" refers to the techniques and methods used in Python to format and print strings efficiently. By mastering these skills, developers can create cleaner and more organized output in their code.
Q3: Can you provide some examples of optimizing string output using format print in Python?
A3: Certainly! Examples include using f-strings, %-formatting, and the format() method to insert variables, align text, control decimal points, and more to improve the appearance of printed strings.
Q4: Are there any common mistakes to avoid when optimizing string output in Python?
A4: Some common mistakes to avoid include excessive concatenation of strings, using inefficient formatting methods, and neglecting to consider formatting options like alignment and padding.
Q5: How can optimizing string output with format print simplify debugging and maintenance of Python code?
A5: By structuring output effectively with format print, developers can easily identify variables and values in printed statements, making it simpler to debug and maintain code in the long run.