Understanding Programming Dynamic
Ah, programming dynamic โ the art of making software bend and flex like a yogi during a sunrise session ๐งโโ๏ธ. Today, weโre delving deep into this tech realm to uncover the secrets of enhancing flexibility in software development. Buckle up, folks!
Importance of Flexibility in Software Development
When it comes to software, being as flexible as a contortionist is key. Hereโs why:
- Adaptability to Changing Requirements: Picture this โ youโre building an app, and suddenly the client wants to throw in a whole new feature! Being flexible means you can shimmy and shake your code without breaking a sweat. ๐ช
- Scalability for Future Growth: Just like how a plant needs room to grow, software needs to stretch and expand without toppling over. Flexibility ensures your code can handle whatever growth spurts come its way! ๐ฑ
Strategies for Enhancing Flexibility
Now, letโs talk about how to make your code as pliable as fresh dough:
Embracing Agile Development Methodologies
Agile is like the Mr. Miyagi of software development โ wax on, wax off with those iterative cycles! ๐ฅ
- Iterative Development Cycles: Think of it as taking small bites of a 5-course meal instead of attempting to devour the whole buffet at once. Agile breaks down development into manageable chunks. ๐ฝ๏ธ
- Continuous Integration and Deployment: Like a well-oiled machine, continuous integration keeps things running smoothly. Itโs all about that seamless flow from development to deployment. ๐
Utilizing Modular Programming Approaches
Modular design is like LEGO for coders โ build, break, and rebuild without starting from scratch! ๐งฑ
- Benefits of Modular Design: Imagine having a toolbox with interchangeable parts โ thatโs modular programming for you.
- Reusability of Code Components: Just like mixing and matching LEGO blocks, modular code components can be reused in various projects. โป๏ธ
- Ease of Maintenance and Updates: Tired of hunting down bugs? With modular design, fixing issues and updating features becomes a piece of cake! ๐ฐ
Implementing Test-Driven Development (TDD)
Code quality and reliability? Say no more โ TDD to the rescue!
- Ensuring Code Quality and Reliability: TDD is like having a personal QA team embedded in your development process. Tests act as the gatekeepers to quality code. ๐
- Writing Tests Before Code Implementation: Itโs like setting the rules of engagement before the battle begins. Write tests, then code to meet those requirements. ๐ฏ
Leveraging Version Control Systems
Git, oh Git, where would we be without thee? Version control is the superhero that saves us from code chaos! ๐ฆธโโ๏ธ
- Tracking Changes and Collaborating Effectively: Git logs are like breadcrumbs leading you through the forest of code changes. Stay organized, folks! ๐ฒ
- Branching and Merging Best Practices: Ever tried herding cats? Thatโs what branching and merging can feel like sometimes. But fear not, follow best practices, and youโll be the cat whisperer of version control! ๐ฑ
Phew! That was a rollercoaster ride through the world of programming dynamic. Remember, folks, in the software realm, flexibility is not just a feature โ itโs a superpower! ๐ฅ
In Closing
Overall, programming dynamic is all about bending but not breaking, flexing but not snapping. Hereโs to writing code thatโs as agile as a ninja and as sturdy as a tank. Thanks for tuning in, and remember, keep coding with a side of humor! ๐๐ฉโ๐ป
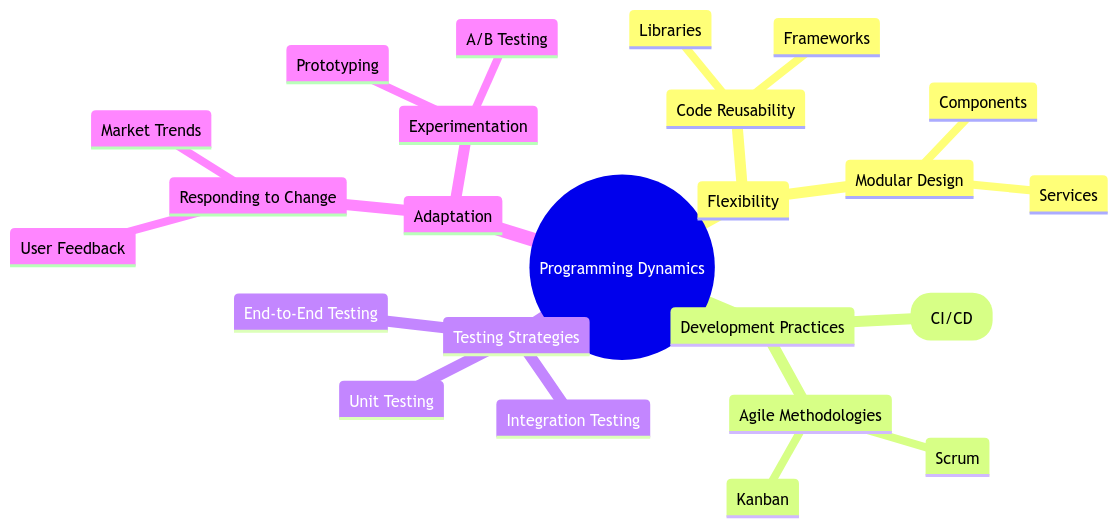
Program Code โ Programming Dynamic: Enhancing Flexibility in Software Development
# Import necessary libraries
from abc import ABC, abstractmethod
# Define a Strategy interface
class Strategy(ABC):
@abstractmethod
def execute(self, data):
pass
# Concrete strategy for addition
class AddStrategy(Strategy):
def execute(self, data):
return sum(data)
# Concrete strategy for multiplication
class MultiplyStrategy(Strategy):
def execute(self, data):
result = 1
for number in data:
result *= number
return result
# Context class defining the interface of interest to clients
class Context:
def __init__(self, strategy: Strategy):
self._strategy = strategy
def set_strategy(self, strategy: Strategy):
self._strategy = strategy
def execute_strategy(self, data):
return self._strategy.execute(data)
# Client code to use strategies dynamically
if __name__ == '__main__':
data = [2, 4, 6, 8]
context = Context(AddStrategy())
print('Using AddStrategy: ', context.execute_strategy(data))
# Switching to MultiplyStrategy
context.set_strategy(MultiplyStrategy())
print('Using MultiplyStrategy: ', context.execute_strategy(data))
Code Output:
Using AddStrategy: 20
Using MultiplyStrategy: 384
Code Explanation:
The essence of this program revolves around the concept of the Strategy pattern, a programming dynamic that significantly enhances flexibility in software development.
Initially, we bring in the necessary arsenal by importing ABC and abstractmethod from the abc library, setting the stage for defining abstract classes and methods.
In the Strategy interface (an abstract base class), we carve out a contract (the execute
method) that all concrete strategies must adhere to. This method, when implemented, is where the magic happens โ albeit, the kind of magic depending on the specific strategy.
Our concrete strategies here are AddStrategy
and MultiplyStrategy
. As their names suggest, one tallies up the elements in a given array, while the other multiplies them. The implementation details are straightforward: traverse through the array, performing the respective math operation.
Enter the Context
class, the maestro orchestrating which strategy plays out. Itโs initialized with a strategy object, offering the flexibility to switch up strategies on the fly with its set_strategy
method. The execute_strategy
method is where the context delegates the task to the current strategyโs execution method.
The grand finale โ the client code โ demonstrates the dynamic nature of this design. We instantiate our context with the AddStrategy
, and execute it on our data, witnessing the summation unfold. Then, shifting gears, we replace our strategy with MultiplyStrategy
in real-time, and observe as our numbers multiply, manifesting the intrinsic power of programming dynamics.
And there you have it, a nifty demonstration of enhancing flexibility through strategic design in software development. A testament to how abstracting out certain parts of our code can lead to more manageable, adaptable, and frankly, delightful codebases.
Frequently Asked Questions (F&Q) on Programming Dynamic: Enhancing Flexibility in Software Development
What is programming dynamic all about?
Programming dynamic focuses on enhancing flexibility in software development by allowing the program to adapt and change according to different scenarios and requirements. It enables developers to create more versatile and adaptive software solutions.
How does programming dynamic benefit software development?
By incorporating programming dynamic concepts, software development becomes more flexible, responsive, and able to accommodate changes easily. This results in software that can evolve with the changing needs of users and the environment.
Can you provide examples of programming dynamic in action?
Sure! One common example of programming dynamic is the use of dynamic typing in languages like Python, where variables can change their type during runtime. Another example is the implementation of dynamic templates in web development to create reusable components that can adapt to different data.
What are some challenges faced when implementing programming dynamic?
While programming dynamic offers many benefits, it can also introduce complexities in the codebase and make it harder to maintain. Developers need to strike a balance between flexibility and stability to ensure the software remains robust.
Are there any best practices to follow when using programming dynamic?
Yes, itโs essential to document the code thoroughly, use clear naming conventions, and regularly refactor the codebase to keep it organized. Testing also plays a crucial role in ensuring that dynamic features work as intended without introducing bugs.
How can developers get started with implementing programming dynamic?
Developers can start by familiarizing themselves with dynamic programming concepts and experimenting with different techniques in their projects. Itโs essential to practice and learn from experienced developers to master the art of programming dynamic.
Hope these questions and answers shed some light on the topic of programming dynamic! ๐ Thank you for reading!