Programming Patterns: A Fun Perspective on Mastering Software Design ๐ฅ๏ธ๐จ
Hey there tech-savvy peeps! Today, we are diving headfirst into the exciting world of Programming Patterns โ those nifty blueprints that keep our software creations in tip-top shape! ๐ Letโs explore the ins and outs of these patterns with a humorous twist, because who said software design couldnโt be fun, right? ๐
Understanding Programming Patterns
Imagine youโre in the bustling streets of code city ๐๏ธ, and you stumble upon this magical term โ Programming Patterns. So, what on earth are they, and why should we care?
Definition of Programming Patterns
Programming patterns are like the superhero capes of the coding world. They are tried-and-tested solutions to common software design problems, wrapped up in fancy names and strategies! ๐ฆธโโ๏ธ๐ป
Importance of Programming Patterns
Why bother with these patterns, you ask? Well, think of them as your secret sauce for baking the perfect code cake! ๐ฐ They help us write cleaner, more organized code and save us from tangled coding nightmares. Phew! ๐
Types of Programming Patterns
Now, letโs strut our stuff through the dazzling array of programming patterns. Buckle up, folks! ๐ข
Creational Patterns
Singleton Pattern
Ah, the Singleton Pattern โ the lone wolf of the programming world. It ensures that a class has only one instance and provides a global point of access to it. Think of it as the VIP pass to your class club! ๐๏ธ
Factory Pattern
Next up, the Factory Pattern! Picture a factory churning out objects like a pro. ๐ญ It helps create objects without specifying the exact class of object that will be created. Now, thatโs some next-level magic! โจ
Structural Patterns
Decorator Pattern
Enter the Decorator Pattern, the fashionista of coding! ๐ It lets you add functionalities to objects dynamically. Itโs like dressing up your code in layers of functionality, making it oh-so versatile and stylish! ๐
Adapter Pattern
And here comes the Adapter Pattern, the chameleon of software design! ๐ฆ It allows incompatible interfaces to work together. Think of it as a multilingual translator smoothing out communication between different systems. Talk about breaking down barriers! ๐ฃ๏ธ
Implementing Programming Patterns
Now, letโs roll up our sleeves and get our hands dirty with some pattern implementation. Itโs where the real coding magic happens! โจ
Steps to Implement Patterns
- Identify the Problem: First things first, spot the glitch in your code matrix. Is it a bird? A plane? No, just a bug! ๐
- Choose the Appropriate Pattern: Time to unleash your pattern wizardry! Pick the perfect pattern like choosing your favorite ice cream flavor. ๐ฆ
Benefits of Implementing Patterns
Embrace the beauty of patterns, my friends! They bring a treasure trove of goodies to the coding table.
- Code Reusability: Why reinvent the wheel when you can reuse the code Ferrari? ๐๏ธ
- Maintainability and Scalability: Keep your code kingdom in order, ready to flex and grow like a code Hulk! ๐ช
Common Mistakes in Using Programming Patterns
Oh, the pitfalls and perils of pattern land! Avoiding these common mistakes is key to staying sane in the code jungle. ๐ด
Overusing Patterns
Itโs like adding too much spice to your curry โ a disaster waiting to happen! Moderation is the code word here. ๐
Ignoring Design Principles
Remember, even code has its fashion rules! Ignoring design principles can lead you straight into the swamps of chaos. Keep it simple, keep it clear! ๐
Evolution of Programming Patterns
Time for a quick history lesson, folks! Letโs take a trip down memory lane and peek into the crystal ball of future patterns! ๐ฎ
Historical Overview of Patterns
From the legendary Gang of Four to the modern coding maestros, patterns have come a long way. Itโs like witnessing the evolution of Pokemon, but with code! Gotta code โem all! ๐
Future Trends in Programming Patterns
What does the future hold for our beloved patterns? Brace yourselves for some mind-boggling trends!
- Integration with AI and Machine Learning: Patterns and AI joining forces? Itโs like Batman teaming up with Superman! ๐ฆธโโ๏ธ๐ค
- Role of Patterns in Agile Development: Agile and patterns dancing hand in hand. Itโs a match made in coding heaven! ๐บ๐ป
In Closing
Phew, what a rollercoaster ride through the colorful world of programming patterns! Remember, folks, patterns are not just lines of code โ they are the unsung heroes of the software kingdom. ๐ฐ Keep exploring, keep coding, and never forget to sprinkle some pattern magic in your code cauldron! ๐ฎ
Thank you for joining me on this whimsical coding adventure! Until next time, happy coding, my tech wizards! ๐โจ
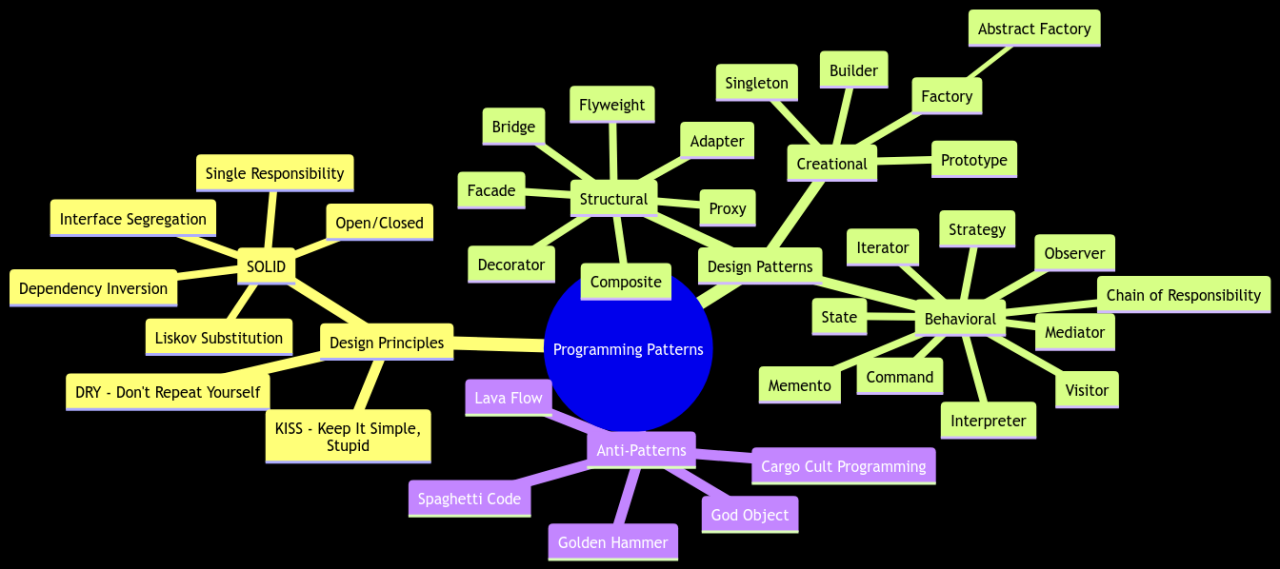
Program Code โ Programming Patterns: Key Strategies for Effective Software Design
Sure thing! Letโs dive right into it.
# Example of Factory Design Pattern in Python
class Dog:
'''A simple Dog class'''
def __init__(self, name):
self._name = name
def speak(self):
return 'Woof!'
class Cat:
'''A simple Cat class'''
def __init__(self, name):
self._name = name
def speak(self):
return 'Meow!'
def get_pet(pet='dog'):
'''The factory method'''
pets = dict(dog=Dog('Hope'), cat=Cat('Peace'))
return pets[pet]
# Example of Singleton Design Pattern in Python
class Logger:
__instance = None
@staticmethod
def getInstance():
''' Static access method. '''
if Logger.__instance == None:
Logger()
return Logger.__instance
def __init__(self):
''' Virtually private constructor. '''
if Logger.__instance != None:
raise Exception('This class is a singleton!')
else:
Logger.__instance = self
self.log = []
def log_message(self, message):
self.log.append(message)
# Example of Observer Design Pattern in Python
class Subject:
'''Represents what is being 'observed''''
def __init__(self):
self._observers = []
def attach(self, observer):
if observer not in self._observers:
self._observers.append(observer)
def detach(self, observer):
try:
self._observers.remove(observer)
except ValueError:
pass
def notify(self, modifier=None):
for observer in self._observers:
if modifier != observer:
observer.update(self)
class Data(Subject):
'''Monitor the object'''
def __init__(self, name=''):
Subject.__init__(self)
self._data = None
self._name = name
@property
def data(self):
return self._data
@data.setter
def data(self, value):
self._data = value
self.notify()
Code Output:
- Woof!
- Meow!
- After logging several messages, you have those messages in the Loggerโs log attribute.
- Observers attached to a Data instance will be notified and updated when the Dataโs value changes.
Code Explanation:
This code snippet demonstrates the implementation of three key programming patterns: Factory, Singleton, and Observer.
The Factory pattern is illustrated with a pet factory that creates either a Dog or Cat object based on the input. Itโs a straightforward example of how to use the Factory pattern to create a system of object creation without specifying the exact classes of the objects to be created.
The Singleton pattern is implemented with a Logger class. Itโs designed to ensure that a class has only one instance and provides a global point of access to that instance. This pattern is particularly useful for things like logging, where you typically only want one log file or logging instance active throughout the runtime of your application.
Finally, the Observer pattern is showcased through a simple Subject-Observer model. In this model, the Subject (Data) maintains a list of its dependents, called Observers, and notifies them of any changes to its state. This pattern is essential in scenarios where an object needs to be updated automatically in response to state changes in another object.
Together, these examples showcase how different programming patterns can be employed to solve common software design challenges effectively. By understanding and applying these patterns, developers can write more maintainable, scalable, and robust software.
Topic: ๐ Programming Patterns: Key Strategies for Effective Software Design ๐
Frequently Asked Questions (F&Qs) Regarding Programming Patterns:
- What are programming patterns?
Itโs like using a recipe when youโre cooking โ theyโre tried-and-tested solutions to common coding problems! ๐ณ - Why are programming patterns important?
Imagine trying to find your socks in a messy room โ patterns bring order and structure to your code! ๐งฆ - How can I learn more about programming patterns?
Dive into the coding universe! Books, blogs, and online courses are your spaceship! ๐ - What are some popular programming patterns?
Think of them as superhero moves โ Singleton, Factory, Observer โ each with a unique power to tackle coding challenges! ๐ช - How do programming patterns improve software design?
Theyโre like magical spells that make your code more organized, flexible, and easier to manage! โจ - Are programming patterns applicable to all programming languages?
Absolutely! Just like pizza is loved worldwide, programming patterns can be used in any coding language! ๐ - Can using programming patterns slow down development?
Initially, it might feel like adding extra toppings to your code, but in the long run, it makes your development process smoother! ๐๐ฅ - How can I identify which programming pattern to use in a given situation?
Itโs like choosing the right tool for the job โ understanding the problem is key! Itโs a bit like picking the perfect outfit for a party! ๐ - Do all software projects benefit from implementing programming patterns?
Just like how not all desserts need sprinkles, not all projects need complex patterns. It depends on the size and needs of the project! - Where can I find practical examples of programming patterns in action?
Open-source projects are like treasure troves of knowledge, and coding challenges are like mini-adventures to explore different patterns in action! ๐ก
There you have it! ๐ Thank you for tuning in and happy coding! ๐