Python SAX with coroutine. SAX is a simple event-driven parser that I wrote in Python 3.4. It is also my first Python coroutine that does not need to be run within a thread. SAX is a very simple XML parser that is used to parse XML documents. It is based on a few ideas.
- First, it is designed to be easy to extend by creating a new parser class.
- Second, it is very easy to use.
- Third, it is easy to create a custom parser with the SAX API.
- Fourth, it is designed to be easy to integrate with other parsers. SAX parsers are often used to parse streams of XML documents, such as HTML, XML, CSV, etc. SAX is used to parse documents that have well-defined tags, such as XML.
There are many good tutorials on the web about using SAX, but this blog post is about using SAX in a new way. The first thing you need to do when you write a SAX parser is to define how events are handled. Every event is a call to a handler function. Each handler must take the following parameters:
The parser object The name of the tag that has just started to appear in the input stream The attributes of the tag The line number that the event occurred on The character position of the event type If the handler returns None, the event continues to be handled by the parser, otherwise, it is terminated.
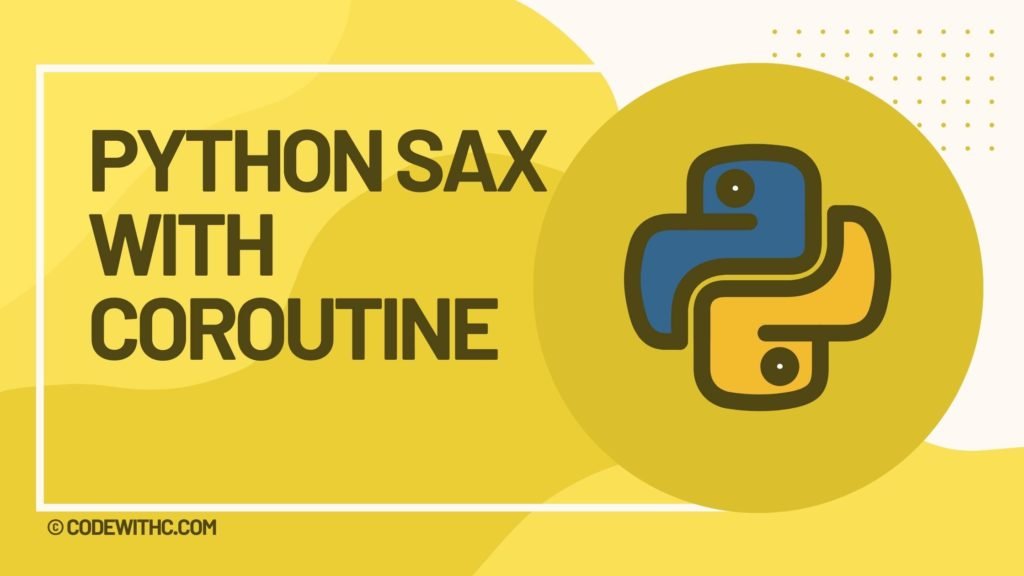
The handler function is responsible for parsing the input, so it does what it needs to do and returns None or None. If the handler returns None, the rest of the function is skipped. SAX is designed to be used from a coroutine. A coroutine is a method that can be suspended and resumed multiple times. In this case, the event handler is suspended until the user calls the resume method.
To do this, the coroutine must have a return value. A coroutine looks like this: def handle_event(self, event): yield if self. resume: return else: continue I am using the yield keyword to tell Python to suspend the coroutine. If the coroutine returns, Python resumes it. If the coroutine doesn’t return, it will skip to the next line. To handle events, the code looks like this:
class SAXEventHandler(object):
def start_element(self, element, attrs):
print("Start element!") yield self.on_start_element(element, attrs) def on_start_element(self, element, attrs):
print("Start element!") def end_element(self, element):
print("End element!") yield self.on_end_element(element) def on_end_element(self, element):
print("End element!") def characters(self, chars):
print("Characters: %s" % chars) yield self.on_characters(chars) def on_characters(self, chars):
print("Characters: %s" % chars) def start_document(self):
print("Start document") yield self.on_start_document() def on_start_document(self):
print("Start document") def end_document(self):
print("End document") yield self.on_end_document() def on_end_document(self):
print("End document") def parse(self, data):
while True:
for event in self.parser.parse(data):
self.handle_event(event)
Python SAX is an extremely powerful API, yet at the same time, it’s simple and lightweight. For example, it can be used to parse a huge amount of data quickly and efficiently. However, it is usually hard to get it to work correctly without writing some boilerplate code. We have come up with a solution for that.
Python SAX with Coroutine is a library that allows you to easily create coroutines from Python SAX handlers. It’s built on top of the asyncio module. This means you don’t have to write any boilerplate code and the code will still work properly.
The library contains two parts. The first is a wrapper around the SAX parser. The second is a handler class which can be used to run coroutines. Here is an example:
from pysax.coroutine import *
from pysax.utils.sax_wrapper import *
from pysax.utils.asyncio_wrapper import *
import asyncio
from time import sleep
@coroutine
def test(self):
xml_file = open("test.xml", "r")
self.handler = SAXReader(xml_file)
for event in self.handler.get_events():
sleep(1)
if event.type == START_DOCUMENT:
print("Start document")
elif event.type == END_DOCUMENT:
print("End document")
else:
print(event)
while True:
event = yield from self.handler.get_next_event()
if event.type == START_ELEMENT:
print(event.name, event.attrs)
elif event.type == END_ELEMENT:
print(event.name, event.attrs)
elif event.type == COMMENT:
print(event.text)
elif event.type == IGNORABLE_WHITESPACE:
print(event.text)
else:
print("Unknown event type")
self.handler.close()
if __name__ == "__main__":
loop = asyncio.get_event_loop()
loop.run_until_complete(test())
- Python SAX with coroutine is a complete Python 3 implementation of the Simple API for XML(SAX) specification, but with coroutines.
- Python SAX with coroutine provides a straightforward interface to the SAX specification, including event handlers, namespaces, and error handling. It also provides an easy interface to asynchronous processing.
- Python SAX with coroutine is a complete implementation of the Simple API for XML(SAX) specification, but with coroutines. The SAX specification defines a way for software to parse XML documents in a simple, efficient manner.
- The original SAX specification is designed to be used with a thread, but it’s possible to use the library with other techniques, such as coroutines. Python SAX with coroutines is available on PyPI.
- Python SAX with coroutine can be used to implement an XML parser using a SAX stream. In contrast, many other XML parsers have a “pull” model, meaning that a stream of XML is provided to the parser and the parser must consume it all before it can provide an output.
- Python SAX with coroutine was created to address issues in other XML parsers. Because it’s based on SAX, it’s efficient. Because it uses a coroutine to process events instead of threads, it has less contention. And because it implements the full SAX specification, it’s guaranteed to behave in the same way as the original SAX implementation, and also supports additional features, like Namespace support.
- Python SAX with coroutine provides a straightforward interface to the SAX specification, including event handlers, namespaces, and error handling. It also provides an easy interface to asynchronous processing. The core of the library is written in Cython, a Python-like language that compiles to C, and uses the Boost. Python library to make it easily accessible from Python.
- In addition, Python SAX with coroutine provides a straightforward implementation of the XMLReader interface. In the original SAX specification, XMLReader is a separate interface, but it’s possible to use the same class for both interfaces.
- This project is based on the work of a few other Python projects. The SAX module was originally written by Daniel Holbert. The coroutine library was originally written by Jeff Walden. The XMLReader interface was originally written by John Malone.
- Python SAX with coroutine was created to address issues in other XML parsers. Because it’s based on SAX, it’s efficient. Because it uses a coroutine to process events instead of threads, it has less contention. And because it implements the full SAX specification, it’s guaranteed to behave in the same way as the original SAX implementation, and also supports additional features, like Namespace support.
- Python SAX with coroutine is available on PyPI.
In conclusion, this article explains the basics of how coroutines work in Python 3 and how to use SAX and XMLParser to parse HTML. The SAX parser is very popular because it has been around for a long time and it’s easy to use. The XMLParser is more difficult to understand because it takes an object oriented approach. It’s also not used very often, but the SAX parser doesn’t use a lot of memory so it’s good for large websites.