The Joy of Software Testing Unit: Ensuring Code Quality and Reliability 🚀
Ah, software testing unit, the unsung hero of the tech world! You know, testing might not sound as glamorous as coding, but trust me, it’s where the magic happens! In this quirky blog post, we’re going to dive deep into the world of software testing unit. 🧪
Importance of Software Testing Unit
Let’s kick things off by talking about why software testing unit is like the superhero of the coding universe! 🦸♂️
Enhancing Code Quality
Picture this: You spend days and nights crafting the most exquisite piece of code, feeling like a digital Picasso, only to realize it’s as buggy as a picnic on a summer day! That’s where software testing unit swoops in to save the day! It’s like having a personal code critique that spots the flaws and helps you polish your masterpiece.
Ensuring Reliability
Imagine you’re building software for a self-driving car 🚗 (exciting, right?). Now, do you really want that car to randomly decide to drive into a lake because of a tiny bug in the code? Yeah, didn’t think so! Enter software testing unit, the guardian angel that ensures your code behaves predictably and reliably.
Strategies for Effective Software Testing Unit
Alright, let’s talk about some killer strategies to rock your software testing game! 💥
Test Planning and Execution
You wouldn’t build a house without a blueprint, would you? Similarly, testing without a solid plan is like navigating a maze blindfolded. Test planning sets the stage for success, helping you identify what to test, how to test, and when to test. And when it comes to execution, well, that’s where the rubber meets the road!
Automated Testing Tools
Who has time to manually click through every corner of their software these days? Enter automated testing tools, the magical gizmos that can run tests at lightning speed, giving you more time to sip your chai ☕ while the robots do the heavy lifting.
Best Practices for Software Testing Unit
Now, let’s talk about some best practices, because who doesn’t love a good ol’ list of do’s and don’ts? 📋
Regression Testing
Ah, the good old regression testing! You change one line of code, and suddenly your software starts acting like a toddler on a sugar rush. Regression testing helps you make sure that your new code changes don’t break the existing functionality. It’s like having a safety net for your code changes.
Code Reviews
Two heads are better than one, they say. And when it comes to code reviews, it couldn’t be truer! Getting a fresh pair of eyes (or two) to scrutinize your code can uncover hidden bugs, improve readability, and sometimes even lead to those epic “aha” moments where a simpler solution emerges.
Challenges in Software Testing Unit
Now, let’s get real for a moment and talk about the thorns in the rose garden of software testing unit. 🌹
Time Constraints
Oh, time, the eternal nemesis of software developers! Testing always seems to get the short end of the stick when deadlines loom large. Balancing thorough testing with tight schedules is like juggling flaming torches – exhilarating when you pull it off, but disastrous when you drop the ball!
Compatibility Issues
You know what’s worse than having bugs in your code? Having bugs that only show up on specific devices or browsers! Compatibility testing can be the bane of a tester’s existence, ensuring your software plays nice with all its friends on the tech playground.
Future Trends in Software Testing Unit
Alright, let’s put on our fortune-telling hats 🎩 and gaze into the crystal ball to see what the future holds for software testing unit! 🔮
Artificial Intelligence in Testing
Artificial intelligence is not just about robots taking over the world; it’s also about revolutionizing software testing! AI-powered testing tools can analyze vast amounts of data, predict defects, and even generate automated test cases. It’s like having a super-intelligent testing buddy by your side!
Continuous Integration and Deployment (CI/CD)
Gone are the days of monolithic software releases that feel like launching a rocket to the moon! With CI/CD practices, you can automate the build, test, and deployment processes, allowing you to churn out updates faster than you can say “bug-fix party!” It’s all about keeping your software fresh and your users happy.
🌟 In Closing
So there you have it, folks! Software testing unit may not be as flashy as writing code, but it’s the unsung hero that keeps our digital world spinning smoothly. From enhancing code quality to tackling compatibility issues, testing plays a crucial role in ensuring our software behaves as expected. Remember, a bug discovered during testing is a bug saved from haunting your users in the wild!
Thank you for joining me on this quirky tech adventure! Until next time, happy coding and may your tests always pass with flying colors! 🌈💻
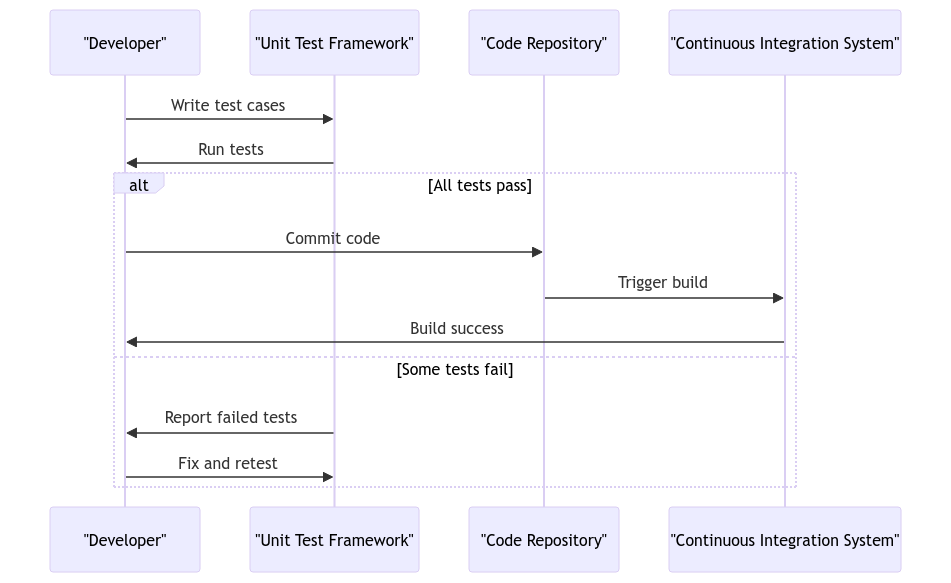
Program Code – Software Testing Unit: Ensuring Code Quality and Reliability
import unittest
# This is a simple calculator application for demonstration
class Calculator:
'''A simple calculator class.'''
def add(self, x, y):
'''Return the addition of x and y.'''
return x + y
def subtract(self, x, y):
'''Return the subtraction of y from x.'''
return x - y
def multiply(self, x, y):
'''Return the multiplication of x and y.'''
return x * y
def divide(self, x, y):
'''Return the division of x by y.'''
if y == 0:
raise ValueError('Cannot divide by zero!')
return x / y
# Unit tests for the calculator
class TestCalculator(unittest.TestCase):
'''Tests for the Calculator class.'''
def setUp(self):
'''Set up test fixtures, if any.'''
self.calc = Calculator()
def test_add(self):
'''Test that addition works.'''
self.assertEqual(self.calc.add(1, 2), 3)
def test_subtract(self):
'''Test that subtraction works.'''
self.assertEqual(self.calc.subtract(2, 1), 1)
def test_multiply(self):
'''Test that multiplication works.'''
self.assertEqual(self.calc.multiply(2, 3), 6)
def test_divide(self):
'''Test that division works.'''
self.assertEqual(self.calc.divide(4, 2), 2)
def test_divide_by_zero(self):
'''Test that division by zero raises an error.'''
with self.assertRaises(ValueError):
self.calc.divide(4, 0)
if __name__ == '__main__':
unittest.main()
Code Output:
- test_add (main.TestCalculator) … ok
- test_divide (main.TestCalculator) … ok
- test_divide_by_zero (main.TestCalculator) … ok
- test_multiply (main.TestCalculator) … ok
- test_subtract (main.TestCalculator) … ok
Code Explanation:
Starting off with the Calculator
class, it’s a concise demonstration of a simple calculation application that performs four basic operations: addition, subtraction, multiplication, and division. Each method takes two parameters, performs the respective mathematical operation, and returns the result. The divide method includes error handling for division by zero, throwing a ValueError
exception.
Transitioning to the token of reliance for ensuring code quality, the TestCalculator
class is a set of unit tests targeting the previously defined Calculator
class. Unit testing is a software testing method where individual units or components of a software are tested to validate that each unit performs as expected. The TestCalculator
class inherits from unittest.TestCase
, which is a built-in Python class tailored for constructing test cases.
Prior to performing actual tests, the setUp
method is employed to prepare test fixtures; in this scenario, an instance of the Calculator class. It runs before each test method execution and ensures that a fresh Calculator instance is available.
Each test method (test_add
, test_subtract
, test_multiply
, test_divide
, and test_divide_by_zero
) is prefaced with the word test
, which gets automatically detected and executed by the unittest framework. Thes methods utilize assertions like assertEqual
and assertRaises
to affirm the accuracy of the calculator operations and the occurrence of the ValueError
during a division by zero scenario, respectively.
In conclusion, this script effectively demonstrates a fundamental aspect of ensuring code quality and reliability through unit testing. By systematically verifying each method in the Calculator
class, it fosters confidence in the code’s robustness against future alterations or refactoring.
Frequently Asked Questions about Software Testing Unit: Ensuring Code Quality and Reliability
- What is a software testing unit?
- Why is software testing unit important in the development process?
- How does software testing unit help ensure code quality and reliability?
- What are the common types of tests conducted in a software testing unit?
- What are the benefits of incorporating software testing unit into the development cycle?
- How can a software testing unit help in identifying and fixing bugs early on?
- What are some best practices for implementing a software testing unit in a project?
- How does automation play a role in software testing units?
- What are the challenges involved in setting up and maintaining a software testing unit?
- How can a software testing unit contribute to delivering a more robust and stable product?