The stack trace is the best way to understand the errors that occur in the python program. To get the details of the error and how the error occurred. Let’s discuss the stack trace.
The stack trace is used to analyze the errors that occur while running the program. The stack trace is the complete list of statements that were executed by the program when the error occurred.
There are two types of stack traces, the top stack trace, and the bottom stack trace. The top stack trace shows the steps followed by the program to execute the error. The bottom stack trace shows the steps followed by the program to execute the error.
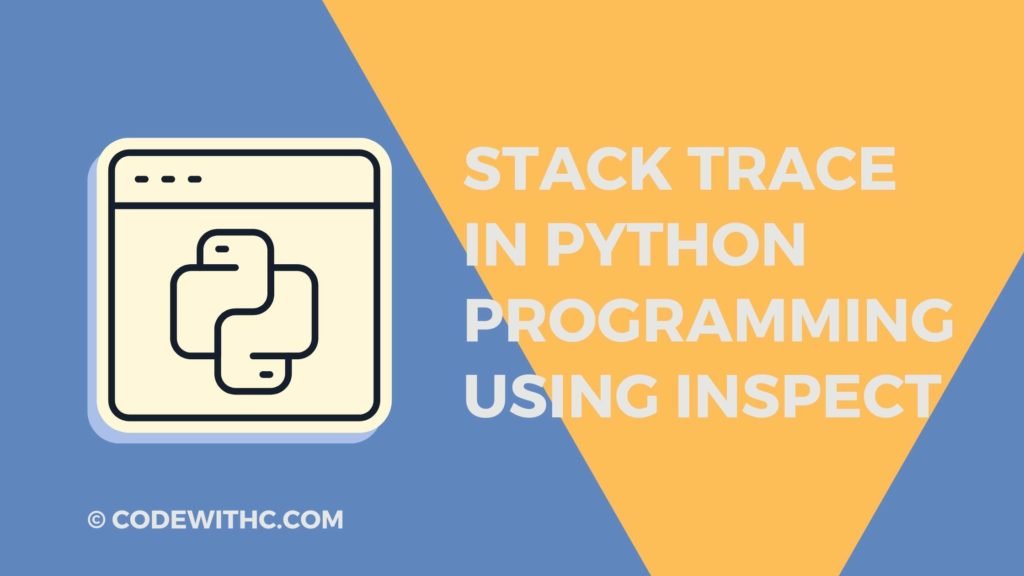
Let’s understand the stack trace in detail.
Stack Trace In Python
Stack trace in Python is a method that is used to show the source code of the program. It will be very helpful to developers and they will be able to find the reason behind the error. So, let’s see how to stack trace in Python works.
Let’s imagine that you have a python program, that contains a function named myfunc(). When you run this program, it will give an error. So, now you want to find out what is wrong with your program.
To find out what is wrong with your program you can use the inspect module. It will provide you with the information that you want to find.
Let’s assume that you have a python file named main.py. And in this file you have written the following lines.
def myfunc():
print("Hi")
if __name__ == '__main__':
myfunc()
Here, you can see that the program is running inside the main.py file. But the problem is that the program is not working as expected.
Now, you can run this program using the following command:
python3 main.py
If the program runs successfully then you will be able to see the output in the console.
If you get the error then you will not be able to find out what is wrong with your program. To find out the error you can use the inspect. stack().
In this case, the output will be as follows:
Traceback (most recent call last):
File "main.py", line 9, in
myfunc()
File "main.py", line 1, in myfunc
print("Hi")
NameError: name'myfunc' is not defined
Now, you can understand that if you get the output of inspecting.stack(), then it will help you to find out what is wrong with your program.
You can also use this module to find out the source code of a Python program.
The stack trace can be divided into two parts, the source code and the line number where the error occurred. Let’s see the example of the stack trace.
Example: 1
Stack trace example:
def main():
print(‘Hello World’)
main()
Here the program is trying to print the message ‘Hello World’. But the message is not printed.
This means that there was an error and the message couldn’t be printed. So, let’s find out what happened.
- This method main() contains the code to execute the program.
- The main method contains the print() statement.
- The print() statement contains the message that needs to be printed.
- The message wasn’t printed because of an error. So, the program stopped at the print statement and the error is the reason for the stop of the program.
- Let’s check the source code, the error occurs.
source code:
def main():
print(‘Hello World’)
main()
It is clear from the source code that the program contains the print statement and the main method.
6) Now let’s see the bottom stack trace.
def main():
print(‘Hello World’)
main()
Here the program starts from line no. 1. The print statement is executed and then the program stops and the error occurs.
7) Let’s see the source code of line no. 1.
source code:
def main():
print(‘Hello World’)
main()
The print statement is contained in the source code.
8) Let’s see the bottom stack trace.
def main():
print(‘Hello World’)
main()
def main():
print(‘Hello World’)
main()
Here the error occurred at line no. 1, the same as in the top stack trace.
9) Let’s see the source code of line no. 1.
source code:
def main():
print(‘Hello World’)
main()
Example: 2
Let’s take an example where a user entered an incorrect password and we want to find out which line of code is causing the issue.
The process of finding the line of code is shown below:
- Create a new file and save it as “bug_fix.py”.
- Import the inspect module and import the print module.
- Run the program.
- The stack trace is displayed in the terminal.
Here is the example code:
import inspect
import pprint
pprint.pprint(inspect.stack())
from bug_fix import *
def main():
password = "12345"
login()
def login():
print("Login")
main()
As the above code is running, we will get the output as follows:
Traceback (most recent call last):
File “bug_fix.py”, line 10, in
main()
File “bug_fix.py”, line 8, in main
login()
File “bug_fix.py”, line 9, in login
print("Login")
UnboundLocalError: local variable ‘login’ referenced before assignment
We can understand the error by looking at the stack trace. It says that line 9 which is the line number of the login function is the culprit.
Example: 3
Let’s assume that you have written a program that will calculate the square root of a number. The program will prompt the user to enter a number and once the user enters the correct number, it will calculate the square root of that number and display the answer.
The below code will show the program that is used to calculate the square root of a number.
def square_root(x):
if x < 0:
print("Sorry, no negative numbers.")
else:
return int(x**0.5)
print("Enter a number to calculate its square root:")
x = input()
print(square_root(x))
Now, if you run this program and enter a number less than zero, it will display the error message as shown below.
Enter a number to calculate its square root: 1
Traceback (most recent call last):
File “C:\test\stacktrace.py”, line 9, in square_root return int(x**0.5)
TypeError: unsupported operand type(s) for ** or pow(): ‘int’ and ‘NoneType’
If you look at the stack trace, it is showing the exact line of the code where the error occurred. It also shows the function name, the file name, and the line of code.
In this blog, I have explained how you can get a stack trace in Python using the inspect module. You can also find out the source code of a Python program using the this module.