Understanding the Core: An Algorithm Definition for Beginners
Hey there, code enthusiasts! Today, I’m diving into the fascinating world of algorithms 🤓. We’re going to unravel the mysteries behind these magical sets of instructions that make our digital world go round. So buckle up, grab some popcorn (or your favorite coding snack), and let’s embark on this algorithmic adventure together! 🚀
Fundamentals of Algorithms
Let’s kick things off with the basics. What exactly are algorithms, and why are they so essential in the realm of computing? Let’s find out! 🧐
What are Algorithms?
Alright, picture this: you’re baking a cake, following a recipe step by step. Well, in the tech realm, an algorithm is like a recipe for computers! It’s a precise set of instructions designed to perform a specific task or solve a problem. Think of it as a roadmap guiding the computer on what to do. 🍰
Algorithms are everywhere in the digital universe, from the apps on your phone to the algorithms that power your favorite social media platforms. They’re the secret sauce behind all the magic you see on your screens! ✨
Importance of Algorithms in Computing
Now, why should we care about algorithms? Let me tell you, they are the unsung heroes of the tech world! Algorithms are the key to efficiency and effectiveness in computing. They help optimize processes, solve complex problems, and make our lives easier (most of the time, at least). Without algorithms, our digital lives would be a chaotic mess! 🤯
Characteristics of Algorithms
What makes an algorithm tick? Let’s explore its defining features:
- Well-defined Instructions: Algorithms are like bossy chefs; they know exactly what needs to be done at every step. No room for confusion here! 🍳
- Finite Steps to Complete a Task: Just like a good book, every algorithm has a well-defined ending. It’s not an endless loop of instructions; there’s a clear finish line! 🏁
Types of Algorithms
Algorithms come in various flavors, each with its unique twist. Here are a couple of popular ones:
- Sequential Algorithms: These algorithms follow a step-by-step approach, like a neat queue at your favorite food joint. First come, first served! 🍔
- Parallel Algorithms: Picture a multitasking wizard; that’s what parallel algorithms are all about! They juggle multiple tasks simultaneously, like a master of efficiency. 🧙♂️
Algorithm Complexity Analysis
Now, let’s peek into the complexities of algorithms. It’s not all rainbows and unicorns; there’s some serious analysis going on behind the scenes!
Time Complexity
Time is money, even in the world of algorithms! Time complexity analyzes how the algorithm’s execution time grows as the input size increases. The faster, the better—no one likes a slowpoke algorithm! 🐌⏱️
Space Complexity
Ah, space—the final frontier of algorithms! Space complexity delves into how much memory an algorithm needs to do its thing. Efficient use of memory is key to a well-behaved algorithm! 🚀🧠
Practical Applications of Algorithms
Alright, enough theory—let’s talk real-world applications! Algorithms aren’t just theoretical mumbo jumbo; they actually do some pretty cool stuff:
- Sorting and Searching Algorithms: Ever wondered how Google finds that one webpage out of billions in a fraction of a second? That’s the magic of sorting and searching algorithms at work! 🔍
- Machine Learning Algorithms: Ah, the darling of tech enthusiasts everywhere! Machine learning algorithms power everything from recommendation systems to self-driving cars. They’re like digital wizards making sense of heaps of data! 🤖📊
Overall, It’s Algorithm Magic!
In closing, algorithms are the beating heart of the digital world. They power the apps we love, the tech we rely on, and the innovations that shape our future. So next time you marvel at a nifty app or a mind-blowing tech gadget, remember: algorithms are the unsung heroes making it all possible! 💪
Thanks for joining me on this algorithmic rollercoaster ride! Until next time, happy coding and may your algorithms always run smoothly! 🌟🚀
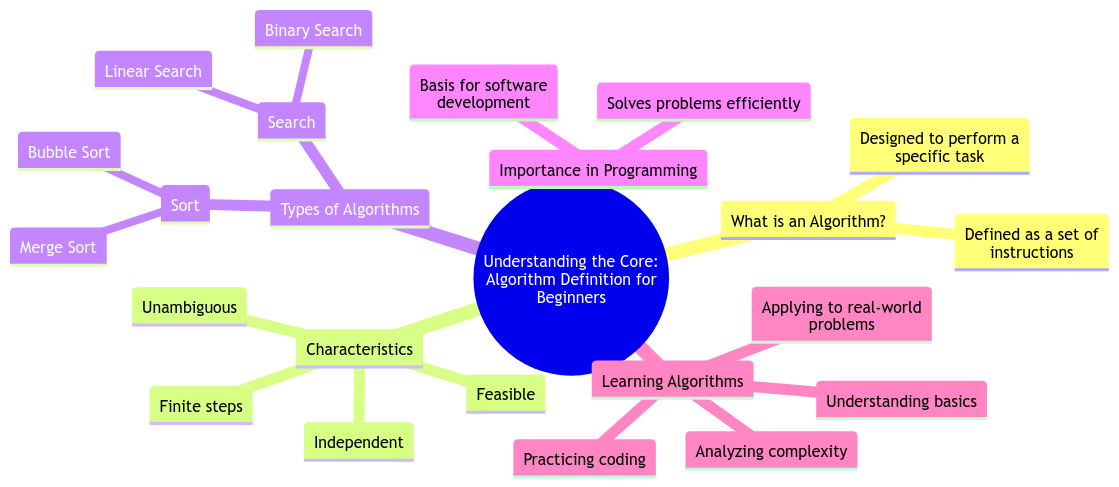
Program Code – Understanding the Core: An Algorithm Definition for Beginners
def bubble_sort(arr):
'''
A simple implementation of bubble sort.
:param arr: A list of elements that needs to be sorted.
:return: The same list, but sorted in ascending order.
'''
n = len(arr)
# Traverse through all array elements
for i in range(n):
swapped = False
# Last i elements are already in place
for j in range(0, n-i-1):
# traverse the array from 0 to n-i-1
# Swap if the element found is greater
# than the next element
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
swapped = True
# IF no two elements were swapped by inner loop, then break
if not swapped:
break
return arr
# Sample array
arr = [64, 34, 25, 12, 22, 11, 90]
# Function call
sorted_arr = bubble_sort(arr)
print('Sorted array is:', sorted_arr)
Code Output:
Sorted array is: [11, 12, 22, 25, 34, 64, 90]
Code Explanation:
The algorithm defined in the code is a basic implementation of the bubble sort algorithm, a fundamental concept often encountered when learning about sorting algorithms. The purpose here is to sort a list of elements in ascending order, showcasing a vivid example of an algorithm definition for beginners.
How the algorithm works:
- Initialization: We start with a function
bubble_sort
that takes a listarr
as its parameter. - Traversal and Comparison: The list is traversed from the first element to the last. For each element, it is compared with the next one. This part is crucial because it determines the order of elements.
- Swapping: If the current element is greater than the one following it, their positions are swapped. This is the core action that moves larger elements towards the end of the list, hence the name ‘bubble’ as larger elements ‘bubble’ up to the end of the list.
- Optimization Check: After each traversal, we check if any swaps occurred. If not, we break out of the loop early. This is an optimization to avoid unnecessary passes once the list is already sorted.
Mechanism Behind the Scenes:
- Two nested loops bring the complexity, where the outer loop represents each pass through the list, and the inner loop focuses on comparing adjacent elements.
- A boolean flag
swapped
is used to track if any swap occurred in the inner loop, enhancing the efficiency by potentially cutting down the number of passes.
Conclusion:
Through this bubble sort example, the idea of an algorithm operating systematically to achieve a specifically defined objective—here, sorting—is illustrated. An algorithm, by definition, is a set of rules or steps designed to perform a task or solve a problem. This program effectively simplifies the algorithm definition, portraying the essence of algorithmic thinking which is pivotal in programming and computer science.
F&Q (Frequently Asked Questions) on Understanding the Core: An Algorithm Definition for Beginners
What is an algorithm definition?
An algorithm definition refers to a set of well-defined instructions or rules designed to solve a specific problem. In simpler terms, it is a step-by-step procedure followed to carry out a particular task or calculation.
Why is understanding algorithm definitions important for beginners?
Understanding algorithm definitions is vital for beginners as it forms the foundation of programming and problem-solving skills. It helps in developing logical thinking, improving efficiency in coding, and enhancing the ability to tackle complex scenarios.
How can beginners grasp the concept of algorithm definitions effectively?
Beginners can grasp the concept of algorithm definitions effectively by practicing regularly, breaking down problems into smaller steps, seeking guidance from experienced programmers, and exploring various resources such as online tutorials and courses.
Are algorithm definitions limited to a specific programming language?
No, algorithm definitions are not bound to a specific programming language. They are universal concepts that can be applied across different programming languages and platforms. The focus is on the logic and steps involved in solving a problem rather than the syntax of a particular language.
Can you provide an example of an algorithm definition for better understanding?
Sure! An example of an algorithm definition is the “Bubble Sort” algorithm used to sort a list of elements in ascending or descending order. It involves comparing adjacent elements and swapping them if they are in the wrong order, iterating through the list until it is sorted.
How can practicing algorithm definitions benefit aspiring programmers?
Practicing algorithm definitions can benefit aspiring programmers by enhancing their problem-solving abilities, improving coding efficiency, preparing them for technical interviews, and laying a strong foundation for advanced topics in computer science.
Where can beginners find resources to learn more about algorithm definitions?
Beginners can find resources to learn more about algorithm definitions through online coding platforms like LeetCode, Codecademy, and Khan Academy, as well as by referring to books such as “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein.
Remember, understanding algorithm definitions is like mastering a recipe – it takes practice, patience, and a sprinkle of creativity! 🌟