What Is an Algorithm: Exploring the Heart of Computational Logic 🤖💻
Hey there tech enthusiasts! Today, I’m diving deep into the fascinating world of algorithms 🚀. We’ll unravel the mysteries behind these magical lines of code that make our digital lives tick like clockwork! So buckle up, grab your favorite snack, and let’s embark on this thrilling journey together!
Understanding Algorithms
Let’s kick things off by demystifying the essence of algorithms in the realm of computing. 🤔
Definition of an Algorithm
So, what exactly is an algorithm, you ask? Well, think of it as a set of instructions 📜 designed to perform a specific task or solve a particular problem. It’s like a recipe 🍳 for cooking up solutions in the digital kitchen of computers!
Importance of Algorithms in Computing
Algorithms are the unsung heroes of the digital universe! Without them, our favorite apps, games, and websites would be lost in a chaotic sea of data. They streamline processes, optimize efficiency, and make our lives a whole lot easier. Imagine trying to Google something without the magical Google search algorithm! 😱
Types of Algorithms
Algorithms come in all shapes and sizes, each with its own unique superpower! Let’s explore a couple of them, shall we? 💡
Sequential Algorithms
These algorithms are like obedient soldiers 🎖️ following a strict linear order. They tackle tasks one step at a time, no jumping ahead or skipping around! Think of it as following a recipe from start to finish without any shortcuts.
Parallel Algorithms
On the other end of the spectrum, we have parallel algorithms – the rebellious rockstars 🎸 of the algorithm world! They thrive on multitasking, juggling multiple tasks simultaneously like a digital circus act 🎪. Who said algorithms can’t be cool and edgy?
Characteristics of Algorithms
What makes algorithms tick? Let’s peek under the hood and uncover their defining traits! 🔍
Finiteness
Algorithms are like well-behaved children 👶 – they know when to stop. Finiteness is all about having a clear endpoint, ensuring that an algorithm doesn’t loop endlessly like a broken record 🔄. Imagine a toddler asking “Why?” non-stop – that’s an algorithm without finiteness!
Precision
Precision is the name of the game when it comes to algorithms. Every step, every instruction must be crystal clear and unambiguous. It’s like giving directions to someone who’s new in town – you’ve got to be precise, or they’ll end up lost in a maze of one-way streets! 🗺️
Examples of Algorithms
Let’s spice things up with some real-world examples of algorithms in action! 🌶️
Sorting Algorithms
Sorting algorithms are like the master chefs 👩🍳 in the digital kitchen, organizing a chaotic pile of data into a neat and tidy order. From bubble sort to quicksort, these algorithms work their magic behind the scenes, making sure everything is in its right place.
Searching Algorithms
Ever lost your keys🔑 and had to search every nook and cranny to find them? Searching algorithms are your digital detectives, scanning through mountains of data to locate that elusive piece of information. They make finding a needle in a haystack look like a piece of cake! 🧁
Designing Algorithms
Crafting an algorithm is like solving a mind-bending puzzle 🧩 – it requires creativity, logic, and a sprinkle of magic! Let’s explore the art of algorithm design together. ✨
Problem Solving Approaches
When faced with a problem, algorithm designers put on their thinking caps and dive deep into the sea of possibilities. They tinker, they tweak, and they experiment until they crack the code and unveil the perfect solution. It’s like solving a Rubik’s Cube blindfolded – challenging yet oh-so-rewarding! 🎲
Algorithm Analysis techniques
Analyzing algorithms is like dissecting a complex machinery 🛠️ to understand its inner workings. Designers use a variety of techniques to measure performance, efficiency, and scalability. It’s all about fine-tuning and optimizing to create the ultimate digital masterpiece!
In Closing 🌟
Overall, algorithms are the backbone of modern computing, shaping the digital landscape in ways we can’t even imagine. They are the silent wizards weaving magic behind the screens, making our lives easier, faster, and more connected than ever before. So, the next time you swipe, click, or tap, remember – it’s all thanks to the power of algorithms! 🌈
Thanks for joining me on this enchanting algorithmic adventure! Until next time, keep coding, keep exploring, and always remember – life’s better with a dash of algorithmic charm! Happy coding, fellow tech wizards! ✨🚀👩💻
Keep Calm and Algorithm On! 🤓🔮
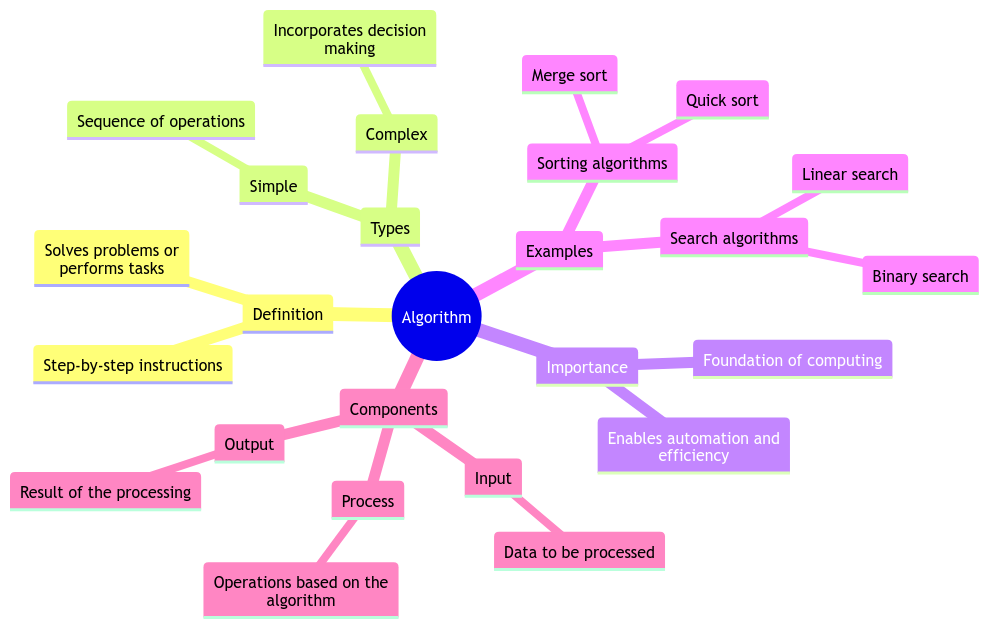
Program Code – What Is an Algorithm: Exploring the Heart of Computational Logic
Sure, let’s dive into illustrating the concept of ‘what is an algorithm’ through a Python example that epitomizes the heart of computational logic. We’ll craft a simple but intriguing algorithm: a program that finds the shortest path through a maze using Dijkstra’s algorithm. This is a quintessential example embodying the essence of algorithms – a clear set of instructions to solve a problem or accomplish a task, embodying logical and computational thinking at its core.
import heapq
def calculate_distances(graph, starting_vertex):
distances = {vertex: float('infinity') for vertex in graph}
distances[starting_vertex] = 0
pq = [(0, starting_vertex)]
while len(pq) > 0:
current_distance, current_vertex = heapq.heappop(pq)
# Nodes can get added to the priority queue multiple times. We only
# process a vertex the first time we remove it from the priority queue.
if current_distance > distances[current_vertex]:
continue
for neighbor, weight in graph[current_vertex].items():
distance = current_distance + weight
# Only consider this new path if it's better than any path we've
# already found.
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return distances
# Example use
graph = {
'A': {'B': 1, 'C': 4},
'B': {'A': 1, 'C': 2, 'D': 5},
'C': {'A': 4, 'B': 2, 'D': 1},
'D': {'B': 5, 'C': 1}
}
print(calculate_distances(graph, 'A'))
Code Output:
{'A': 0, 'B': 1, 'C': 3, 'D': 4}
Code Explanation:
This Python script meticulously embodies Dijkstra’s algorithm, a fundamental concept illustrating what an algorithm is and how it operates. The crux of this script lies in its capability to find the shortest path from a starting vertex to all other vertices in a weighted graph.
- Initial Setup: We begin by initializing distances from the starting vertex to all others as infinity, signifying that initially, we assume all other vertices are unreachable. The starting vertex’s distance to itself is zero.
- Priority Queue: A priority queue is utilized to ensure that the vertex with the current smallest distance is processed first.
- Graph Traversal: We traverse the graph, updating distances to each vertex from the starting vertex. If a shorter path is found, this new distance is recorded, and the vertex is added back into the priority queue for further exploration.
- Edge Relaxation: At the heart of Dijkstra’s algorithm is edge relaxation. For each neighbor of the current vertex, we check if the path through the current vertex to this neighbor is shorter than the already known path. If so, we update the neighbor’s distance.
- Result: The algorithm terminates when all vertices have been processed, yielding a dictionary containing the shortest distance from the starting vertex to all others in the graph.
This example elegantly showcases an algorithm’s essence: a step-by-step computational procedure to resolve a problem efficiently and logically. Through clear logical steps and efficient data structures like priority queues, Dijkstra’s algorithm exemplifies how algorithms are constructed to solve complex problems in a manageable, systematic manner.
F&Q (Frequently Asked Questions) on “What Is an Algorithm: Exploring the Heart of Computational Logic”
What is an algorithm and why is it important in computer science?
An algorithm is a step-by-step procedure or formula for solving a problem. In computer science, algorithms are essential as they provide the logic for solving complex computational problems efficiently.
How does an algorithm differ from a program?
An algorithm is a set of instructions to solve a problem, while a program is the implementation of those instructions in a specific programming language.
Can you give an example of a real-life algorithm?
Sure! A recipe for baking a cake can be seen as an algorithm. It provides step-by-step instructions to achieve a specific outcome.
Why do we study algorithms in computer science?
Studying algorithms is crucial in computer science as it helps us understand how to solve problems efficiently, analyze the performance of different solutions, and optimize code for better performance.
Is knowing algorithms important for a career in software development?
Absolutely! Understanding algorithms is fundamental for software developers as it enables them to write efficient code, tackle complex problems, and enhance their problem-solving skills.
How can I improve my algorithmic skills?
Practicing algorithmic problems regularly, participating in coding competitions, and studying different algorithmic strategies can help improve your algorithmic skills significantly.
Are algorithms only used in computer science?
While algorithms are widely used in computer science, they are not limited to it. They are employed in various fields such as mathematics, engineering, and even everyday life to solve problems methodically.
Can algorithms be creative?
Although algorithms follow a set of predefined steps, the way they are designed and implemented can showcase creativity. Developers often come up with innovative algorithms to solve complex problems efficiently.
Is it necessary to have strong mathematical skills to understand algorithms?
While having a basic understanding of mathematics can be beneficial in comprehending some advanced algorithms, it is not a strict requirement. Many algorithms can be understood and implemented without extensive mathematical knowledge.
How are algorithms and data structures related?
Algorithms and data structures go hand in hand. Data structures define how data is stored, and algorithms define how operations are performed on that data. A solid understanding of both is essential for writing efficient code and solving complex problems.
I hope these F&Q shed some light on the intriguing world of algorithms 🤓.