In Python, the two most important types of ranges are those defined by the built-in function range() and those defined by the built-in function xrange().
Use the range function to create a list of numbers from 1 to 10 using Python’s built-in range() function. You might think you have to use the xrange() function in order to get your results—but not so fast. range() is the built-in Python function for creating a list of numbers from one to another. xrange() creates a range of numbers from one to the next. In this example, let’s see how you can use range() instead of xrange().
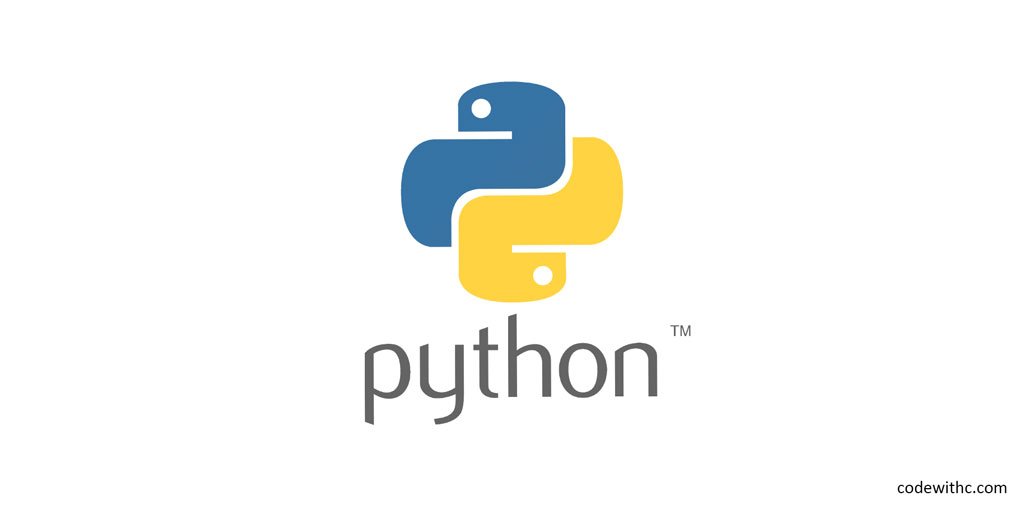
Here are some differences between range() and xrange(). This function will return a range between two numbers: range(x, y) The result is a list of integers between x and y. So if x is 3 and y is 6, it will return [3, 4, 5, 6]. If x is 0 and y is 10, it will return [0, 1, 2, 3, 4, 5, 6]. This function will return a generator object: xrange(x, y) It will iterate through all the numbers in the given range. You could iterate over all the items in a list or dictionary, for example: for i in xrange(1, 20): print i In Python 3, xrange() returns a generator object, whereas range() returns a list object: >>> xrange(1, 5) (1, 2, 3, 4) >>> range(1, 5) [1, 2, 3, 4] # If you use the xrange() in a for loop, you can’t use range(): >>> for i in xrange(1, 5): pass # SyntaxError: invalid syntax.
from future import print_function
import sys
r = range(1000)
x = xrange(1000)
for v in r: # 0..999
pass
for v in x: # 0..999
pass
print(sys.getsizeof(r)) # 8072
print(sys.getsizeof(x)) # 40