Solving quadratic equations or finding the roots of equations of second degree is a popular problem in many programming languages. The equations of second degree which resemble the standard form: ax2+bx+c=0, are known as quadratic equations. A large number of quadratic equations need to be solved in mathematics, physics and engineering. In this tutorial, we’re going to discuss a program for Solving Quadratic Equations in C programming language.
Here, I’ve briefly discussed the working mechanism or algorithm for solving quadratic equations in C; the algorithm can be used to write a program to solve quadratic equations in any high level programming language. The program source code, as shown in the output screens, is applicable for both types of roots of the quadratic equations: real and imaginary.
Algorithm:
The working principle of this program to find the roots of a quadratic equation is similar to manual algebraic solution. The calculation procedure or the formulas to find out the roots is same. The program presented here follows the following steps:
- Enter the value of a, b and c
- After getting these values, the program calculates the value of discriminant, dis= b2-4ac.
- It checks the value of discriminant whether it is less than zero or greater than zero.
- If the dis< 0, the roots are imaginary.
r1 = -b/2a +√dis*i/2a
r1 = -b/2a -√dis*i/2a - Otherwise, there exist two real roots: r1 and r2.
r1 = (-b + √dis)/2
r2 = (-b – √dis)/2 - Finally, the C program displays the roots as output.
Solving Quadratic Equations in C:
#include<stdio.h>
#include<math.h> //for sqrt() function
main()
{
float a,b,c,dis,r1,r2;//a,b,c are constant coefficients, dis= discriminant and r1,r2 are roots
printf ("\nQuadratic Equation is of the form: ax^2 + bx + c = 0 \n");
printf("\nEnter the values of a, b and c: ");
scanf("%f %f %f",&a,&b,&c);
dis = pow(b,2) - 4*a*c; // calculation of discriminant
if(dis < 0) //checking the value of discriminant
{
printf("\nThe roots are imaginary.\n\n");
printf("Root1= %.3f % + .3fi",-b/(2*a),sqrt(-dis)/(2*a));
printf("\nRoot2= %.3f %+ .3fi\n",-b/(2*a),-sqrt(-dis)/(2*a));
}
else
{
r1 = (-b + sqrt(dis))/(2.0*a);
r2 = (-b - sqrt(dis))/(2.0*a);
printf("\nThe first root is = %f\nThe second root is = %f\n",r1,r2);
}
return 0;
}
This program to find the roots of quadratic equations has two header files: stdio.h and math.h. The first header file is to control the console inputs and outputs whereas the math.h is for finding out the square roots during calculation of discriminant.
As the program is executed, it asks for the values of a, b and c which have been defined as float data type; it is better to use float than int as the roots mostly come in decimal points. Then, the program calculates the value of discriminant and compares it with 0. If the discriminant is found to be less than zero, the values of imaginary roots are displayed, otherwise the real roots are displayed as solution.
When the values of a, b and c are entered as 1, -5 and 5, real roots are displayed. For input values of 4, 5 and 6, the roots come out to be imaginary as shown in the output screens below.
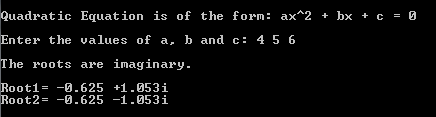
This source code for solving quadratic equations in C is short and simple to understand. You need to compile the source code in Code::Blocks IDE. If you have any queries or suggestions regarding this post or source code, mention/discuss them below in the comments section.
You can find more C Tutorials here.