What is an iterator? – An iterator is a special object in Python which can be used for storing a sequence. It is an object which has a built-in next() method.
In Python 3.5, there was a small addition to the standard library called the Iterator protocol. This protocol allows any object to provide iteration methods. In addition to the list() method, objects that support the protocol can also provide methods to retrieve elements in a loop. The protocol is designed to be easily implemented, and it’s been used in many libraries to provide various kinds of iteration.
There are two problems with the Iterator protocol. The first problem is that it was limited to Python 3.5 and higher. The second problem is that the protocol only provides basic iteration methods. To provide more complex iteration, we have to extend the protocol.
One way to extend the protocol is to add new methods to the iterator class. However, this would mean that every iterator would need to extend the iterator class. The problem with this approach is that we would lose the simplicity of the protocol.
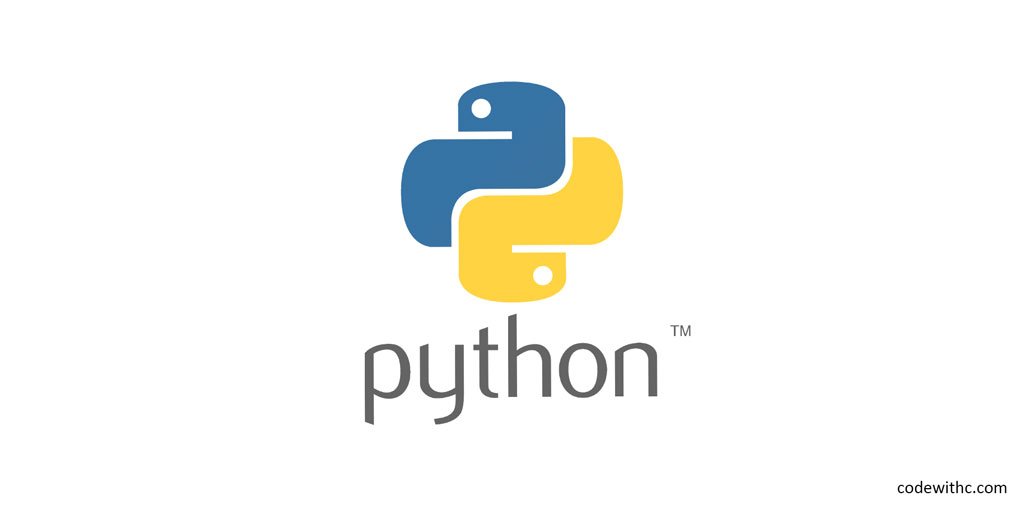
Another option is to provide an extension to the iterator class that can be applied to any iterator. This approach solves the problem of having to extend every iterator, but it doesn’t solve the problem of having to apply a new extension method. This is because Python doesn’t have a way to automatically apply the extension to existing iterators.
To solve both of these problems, an alternative approach to extending the Iterator protocol. The idea is to provide a new function that returns a custom iterator. A custom iterator is a new type that can be used in place of the built-in iterator type. We can then add iteration methods to the custom iterator.
This new function is the Iterator Protocol Generator. The Iterator Protocol Generator is available in Python 3.6 and higher. It is part of the collections module, so you need to include import collections in order to use it.
The Iterator Protocol Generator is designed to generate custom iterators. The generator takes two arguments. The first argument is a class that defines the iterator protocol. The second argument is a function that generates an instance of the iterator class. The function can return an instance of the iterator class directly or it can return a custom subclass of the iterator class.
To use the Iterator Protocol Generator, you need to call the make_iterator() method on the generator. The method takes two arguments, the first being a class that implements the Iterator protocol and the second being a function that returns an instance of that class.
Python iterator Program – pairwise
def pairwise(iterable):
"s -> (s0,s1), (s2,s3), (s4, s5), ..."
i = 0
while i+1 < len(iterable):
t= (iterable[i], iterable[i+1])
i += 2
yield t
l = [1, 2, 3, 4, 5, 6]
1for x, y in pairwise(l):
print(f"{x} + {y} = {x + y}")
Python iterator Program – grouped
def grouped(iterable, n):
"""s -> (s0,s1,s2,...sn-1),
(sn,sn+1,sn+2,...s2n-1),
(s2n,s2n+1,s2n+2,...s3n-1), ..."""
i = 0
while i+n-1 < len(iterable):
t = tuple(iterable[i:i+n])
i += n
1yield t
l = [1, 2, 3, 4, 5, 6, 7, 8, 9]
for x, y, z in grouped(l, 3):
print("{} + {} + {} = {}".format(x, y, z, x + y + z))
Python iterator Program – groupby
from itertools import groupby
def groupby_even_odd(items):
f = lambda x: 'even' if x % 2 == 0 else 'odd'
gb = groupby(items, f)
print(gb)
for k, items in gb:
print('{}: {}'.format(k, ','.join(map(str,
items))))
groupby_even_odd([1, 3, 4, 5, 6, 8, 9, 11])